What Is The Difference Between The Throw And Throws Keyword In Java
- The throw keyword is used to manually throw the exception to the calling method.
- And the throws keyword is used in the function definition to inform the calling method that this method throws the exception. So if you are calling, then you have to handle the exception.
Example –
classMainpublicstaticvoidmaincatch }}
Here in the above snippet, the method testExceptionDivide throws an exception. So if the main method is calling it then it must have handled the exception. Otherwise, the main method can also throw the exception to JVM.
And the method testExceptionDivide ‘throws the exception based on the condition.
Top 8 Java Programs Asked In Interview For Freshers
Team CodeQuotient / /Coding Exams
The IT industry is actively expanding, and almost every company is looking for candidates to fill open positions. Java is perhaps one of the widely used programming languages today. Understanding the fundamentals of Java is critical for anyone looking to break into the IT industry. Because of its popularity, it is one of the most sought-after programming languages in the IT industry.
Java is a general-purpose language that supports both object-oriented and procedural programming styles. It has been around since 1995 and is one of the first object-oriented programming languages to be created.
The language is used to develop a wide variety of applications and is important for businesses, government agencies, schools, and anyone who wants to create an app.
As a fresher, you might be wondering what kind of Java programs you will be asked to write in an interview. While the specific programs will vary depending on the company and position you are interviewing for, here are some commonly asked general categories.
What Is The Main Objective Of Garbage Collection
The main objective of this process is to free up the memory space occupied by the unnecessary and unreachable objects during the Java program execution by deleting those unreachable objects.
- This ensures that the memory resource is used efficiently, but it provides no guarantee that there would be sufficient memory for the program execution.
You May Like: What Should You Ask In An Interview
How To Implement Binary Search
The array elements must be sorted for implementing binary search. The binary search algorithm is based on the following conditions. If the key is less than the middle element, then we now need to search only in the first half of the array. If the key is greater than the middle element, then we need to only search in the second half of the array. And if the key is equal to the middle element in the array, then the search ends. Finally, if the key is not found in the whole array, then it should return -1. This indicates that the element is not present.
public static int binarySearch else if else mid = / 2 }if return -1 }
How Is The New Operator Different From The Newinstance Operator In Java

Both new and newInstance operators are used to creating objects. The difference is- that when we already know the class name for which we have to create the object then we use a new operator. But suppose we dont know the class name for which we need to create the object, Or we get the class name from the command line argument, or the database, or the file. Then in that case we use the newInstance operator.
The newInstance keyword throws an exception that we need to handle. It is because there are chances that the class definition doesnt exist, and we get the class name from runtime. So it will throw an exception.
Also Check: How Much Is Interview Kickstart
What Are The Differences Between Hashmap And Hashtable In Java
HashMap | |
---|---|
HashMap is not synchronized thereby making it better for non-threaded applications. | HashTable is synchronized and hence it is suitable for threaded applications. |
Allows only one null key but any number of null in the values. | This does not allow null in both keys or values. |
Supports order of insertion by making use of its subclass LinkedHashMap. | Order of insertion is not guaranteed in HashTable. |
Write A Program In Java To Show Inheritance In Java
This program is for testing the concepts of inheritance in Java and the usage of extends keyword. Following is an example program in which a class SmartPhone extends a class Phone. This is a real-life example as a phone has basic features of calling and messaging whereas a smartphone has several other features like clicking pictures, playing music, etc.
Java Code for showing Inheritance
class Phone void setNumber int getNumber public void call public void message }class SmartPhone extends Phone public void playMusic public void pauseMusic public void stopMusic }class Main }
Sample Output
Phone number is: 9863472Call is madeMusic Started Playing
Also Check: Where To Get Interview Clothes
Q6 How Does An Exception Propagate In The Code
If an exception is not caught, it is thrown from the top of the stack and falls down the call stack to the previous procedure. If the exception isnt caught there, it falls back to the previous function, and so on, until its caught or the call stack reaches the bottom. The term for this is Exception propagation.
Java Networking Interview Questions
In your interview for a fresher Java role, you might be asked about networking. Some of the questions you might anticipate include:
-
What is Java socket programming?
-
Explain the process of connecting two computers using TCP
-
How can you use Java to connect a client and a server?
-
Describe the process for converting an IP address to a hostname.
Recommended Reading: How To Prepare For Leadership Interview
Q5 Explain The Role Of Dispatcherservlet And Contextloaderlistener
DispatcherServlet is basically the front controller in the Spring MVC application as it loads the spring bean configuration file and initializes all the beans that have been configured. If annotations are enabled, it also scans the packages to configure any bean annotated with @Component, @Controller, @Repository or @Service annotations.
ContextLoaderListener, on the other hand, is the listener to start up and shut down the WebApplicationContext in Spring root. Some of its important functions includes tying up the lifecycle of Application Context to the lifecycle of the ServletContext and automating the creation of ApplicationContext.
What Makes A Hashset Different From A Treeset
Although both HashSet and TreeSet are not synchronized and ensure that duplicates are not present, there are certain properties that distinguish a HashSet from a TreeSet.
- Implementation: For a HashSet, the hash table is utilized for storing the elements in an unordered manner. However, TreeSet makes use of the red-black tree to store the elements in a sorted manner.
- Complexity/ Performance: For adding, retrieving, and deleting elements, the time amortized complexity is O for a HashSet. The time complexity for performing the same operations is a bit higher for TreeSet and is equal to O. Overall, the performance of HashSet is faster in comparison to TreeSet.
- Methods: hashCode and equals are the methods utilized by HashSet for making comparisons between the objects. Conversely, compareTo and compare methods are utilized by TreeSet to facilitate object comparisons.
- Objects type: Heterogeneous and null objects can be stored with the help of HashSet. In the case of a TreeSet, runtime exception occurs while inserting heterogeneous objects or null objects.
You May Like: How To Make An Interview
Top 10 Java 8 Coding And Programming Interview Questions And Answers
Java 8 Interview questions and answerscoding and programming interview questions and answersRead Also:Java 8 Interview Questions and AnswersQ1 Given a list of integers, find out all the even numbers exist in the list using Stream functions?
importjava.util.* importjava.util.stream.* publicclassJavaHungry}
Output:10, 8, 98, 32 Q2 Given a list of integers, find out all the numbers starting with 1 using Stream functions?
importjava.util.* importjava.util.stream.* publicclassJavaHungry}
Output:10, 15Q3 How to find duplicate elements in a given integers list in java using Stream functions?
importjava.util.* importjava.util.stream.* publicclassJavaHungry}
Output:98, 15Q4 Given the list of integers, find the first element of the list using Stream functions?
importjava.util.* importjava.util.stream.* publicclassJavaHungry}
Output:10Q5 Given a list of integers, find the total number of elements present in the list using Stream functions?
importjava.util.* importjava.util.stream.* publicclassJavaHungry}
Output:9 Q6 Given a list of integers, find the maximum value element present in it using Stream functions?
importjava.util.* importjava.util.stream.* publicclassJavaHungry}
Output:98Q7 Given a String, find the first non-repeated character in it using Stream functions?
importjava.util.* importjava.util.stream.* importjava.util.function.Function publicclassJavaHungry}
Output:j Q8 Given a String, find the first repeated character in it using Stream functions?Output:a
In The Below Java Program How Many Objects Are Eligible For Garbage Collection

classMain}
In the above program, a total of 7 objects will be eligible for garbage collection. Lets visually understand what’s happening in the code.
In the above figure on line 3, we can see that on each array index we are declaring a new array so the reference will be of that new array on all the 3 indexes. So the old array will be pointed to by none. So these three are eligible for garbage collection. And on line 4, we are creating a new array object on the older reference. So that will point to a new array and older multidimensional objects will become eligible for garbage collection.
Read Also: How To Prepare For Behavioral Based Interview Questions
Java Garbage Collection Interview Questions
Here are some questions your interviewer may ask you about garbage collection in Java:
-
Explain garbage collection in Java.
-
How do you use garbage collection for cleanup processing?
-
Can an object be unreferenced? Explain.
-
How do you reference an unreferened object?
-
Explain the Runtime class.
-
What is the relationship between the finalise method and garbage collection?
When Can You Use Super Keyword
- The super keyword is used to access hidden fields and overridden methods or attributes of the parent class.
- Following are the cases when this keyword can be used:
- Accessing data members of parent class when the member names of the class and its child subclasses are same.
- To call the default and parameterized constructor of the parent class inside the child class.
- Accessing the parent class methods when the child classes have overridden them.
Also Check: What To Say In Post Interview Email
What Will Happen If We Put A Key Object In A Hashmap Already There
Answer:
HashMap does not allow duplicate keys, so putting the same key again will overwrite the original mapping. The same key will generate the same hashcode and will end up at the same bucket position. Each bucket contains a linked list of maps.
This is an entry object that has both a Key and a Value. Now Java will compare each entrys Key object to this new key using the equals method, and if the comparison returns true, the value object in that entry will be replaced by the new value.
What Do You Mean By Default Methods In Java Se 8
The methods of the Java interface, which comprises the body solely, are acknowledged as the default methods in Java. These methods, as the name implies, use default keywords. The convenience of working with these default methods is “Backward Compatibility.” B??kw?rd ??m??tibility means, if JDK transforms any Interf??e , then the ?l?sses whi?h im?lement this Interf??e will bre?k.
?n the ?ther h?nd, if the user ?dds the def?ult meth?d in ?n interf??e, then they will be ?ble t? ?r?vide the def?ult im?lement?ti?n. This w?n’t ?ffe?t the im?lementing ?l?sses.
Syntax:
Don’t Miss: Do’s And Don Ts Of Interview
Java Works As Pass By Value Or Pass By Reference Phenomenon
Java always works as a pass by value. There is nothing called a pass by reference in Java. However, when the object is passed in any method, the address of the value is passed due to the nature of object handling in Java. When an object is passed, a copy of the reference is created by Java and that is passed to the method. The objects point to the same memory location. 2 cases might happen inside the method:
- Case 1: When the object is pointed to another location: In this case, the changes made to that object do not get reflected the original object before it was passed to the method as the reference points to another location.
For example:
classInterviewBitTest InterviewBitTest}classDriverpublicstaticvoidupdateObject}Output:20
- Case 2: When object references are not modified: In this case, since we have the copy of reference the main object pointing to the same memory location, any changes in the content of the object get reflected in the original object.
For example:
classInterviewBitTest InterviewBitTest}classDriverpublicstaticvoidupdateObject}Output:50
Write A Program In Java To Count The Digits In A Number
Let us consider the number 12345. This is a 5 digit number. The number of digits can be counted as follows:
In the image above, we have shown the way of extracting the digits of a number. However, in our questions, we just need to count the digits in the number. So, we have to count the number of times we can divide our input number by 10 before it becomes 0.
Let us write the code based on the above algorithm.
Java Program to Count the Number of Digits in a Number.
import java.util.* classMainreturn res }publicstaticvoidmain}
Output
Input: 0Output: The number of digits in 0 is: 1
- For Negative Number
Input: -12345Output: The number of digits in -12345 is: 5
- Corner Cases You Might Miss: We have used the loop and carried on iterations till the number becomes 0. What if the number was already 0? It still has 1 digit. So, we have handled that separately. Also, to avoid any confusion, the negative numbers are converted to positive in our function and then we calculate their number of digits.
- Time Complexity: O where N is the input number. This is because we keep dividing the number by 10.
- Auxiliary Space: O as we have not used any extra space.
You May Like: How To Prepare For A Project Manager Interview
Using Relevant Properties Highlight The Differences Between Interfaces And Abstract Classes
- Availability of methods: Only abstract methods are available in interfaces, whereas non-abstract methods can be present along with abstract methods in abstract classes.
- Variable types: Static and final variables can only be declared in the case of interfaces, whereas abstract classes can also have non-static and non-final variables.
- Inheritance: Multiple inheritances are facilitated by interfaces, whereas abstract classes do not promote multiple inheritances.
- Data member accessibility: By default, the class data members of interfaces are of the public- type. Conversely, the class members for an abstract class can be protected or private also.
- Implementation: With the help of an abstract class, the implementation of an interface is easily possible. However, the converse is not true
Abstract class example:
publicabstractclassAthlete
Interface example:
publicinterfaceWalkable
Simple Java Example Programs For Beginners & Experienced Programmers
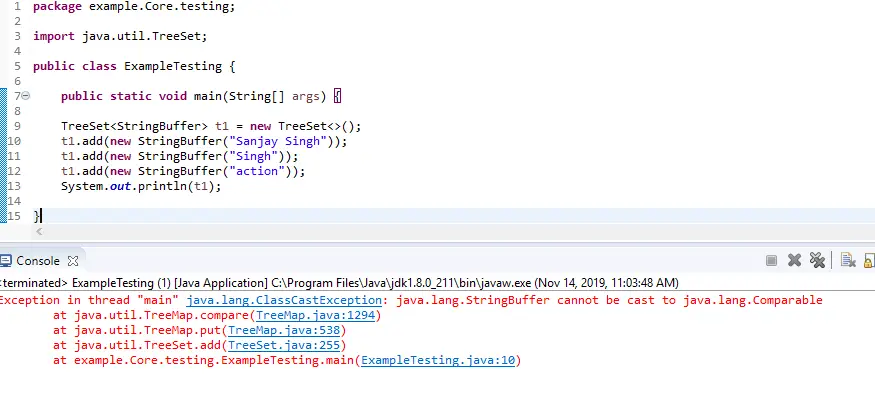
The following 10 simple java programming examples help beginners and expert programmers learn and practice the short logic of the program for quick usage and upskill your knowledge. Lets check these basic yet most commonly asked java programs in interviews:
1. Hello World Java Program:
One of the most important programs to learn by all developers is an introduction program to java by printing Hello World words. Also, check more details about Hello Word Program using Java from here.
2. Prime Number Program in Java:
Out of this plenty of basic java programs list, the most commonly asked programming example in interviews or examinations is Prime Number Java Program. Here is the simple & one-line code for the prime number program. Want to learn more variations about Java Program to Check if a Given Number is Prime Number? Make use of the link provided here.
3. Fibonacci Series in Java:
By using the following simple code, you can easily grasp the logic behind the Fibonacci series. If you really excited to learn the various methods like using recursion and without recursion check out this Java Program to Display Fibonacci Series
4. Factorial Using Java:
Here, we have given a simple code for a factorial java programming example. If you want to explore more about it then go for this Java Program to Find Factorial of a Number
5. Decimal to Binary Conversion in Java:
6. Matrix Transpose Java Code:
7. Armstrong Number in Java:
8. Palindrome Number using Java:
You May Like: How To Ace Your Interview
Java Exception Handling Interview Questions
When interviewing for a position that uses Java, you may answer questions about how you handle exceptions. Here are some examples of Java exception handling interview questions:
-
What types of exceptions can occur in a Java program?
-
Explain exception handling and the hierarchy of exception classes in Java.
-
What are checked and unchecked exemptions?
-
Is there a difference between “throw” and “throws?” Explain.
-
Describe a Java nested class.
-
Discuss the definition of a nested interface.
-
Explain exception propagation.
-
Explain a “finally” block.
-
What are the advantages of using string pool?
-
Explain how to use a string literal and a new keyword to create a string object.
-
What are the differences between String, StringBuffer and StringBuilder?
-
Describe the process of creating an immutable class.
-
Explain the types of inner classes used in Java.
-
Explain the difference between nested classes and inner classes.
-
Explain the difference between metacharacters and ordinary characters in Java.
Q12 What Is An Association
Association is a relationship where all object have their own lifecycle and there is no owner. Lets take the example of Teacher and Student. Multiple students can associate with a single teacher and a single student can associate with multiple teachers but there is no ownership between the objects and both have their own lifecycle. These relationships can be one to one, one to many, many to one and many to many.
You May Like: How To Research A Company For An Interview