Timers In Javascript Settimeout Setinterval Clearinterval
I hope you watched the video. It mentioned timers. Let’s talk about them more now. These are very frequently asked about in interviews.
The setTimeout method calls a function or evaluates an expression after a specified number of milliseconds.
setInterval does the same for specified intervals.
setTimeout => , 2000) const timer = setInterval => , 2000)
You use clearInterval to stop the timer.
clearInterval
Let’s go over some questions that use these concepts.
console.log setTimeout => , 0) console.log // output Hello reader lovely
Here’s a slightly trickier one:
for , i * 1000) }// output66666
And here’s a short explanation of what’s going on there: when setTimeout comes again into the picture, the entire loop has run and the value of i has become 6,
Now, let’s say we want the outcome to be 1 2 3 4 5 what do we do?
Instead of var use let.
Why this will work?
var is globally scoped but let is locally scoped. So for let a new i is created for every iteration.
Remove Duplicates From Array Of Objects
- Arrays of Objects: These are some handy methods that we can use to remove duplicates from an array of objects.
let users = let uniqueUsersByID = _.uniqBy //
We can check unique data with multiple properties with this code.
const uniquewithMultipleProperties = _.uniqWith => a.id === b.id || a.name === b.name)
var set1 = Array.from => m.set, new Map).values) //
You can check out the StackBlitz here.
The Stages Of The Technical Interview
Every company has its own technical interview process, but most of them would have four different stages of interviews:
- Phone screening
- Coding interview
The order of those stages may be different from company to company, but the ideas behind them are pretty much a standard.
Next, well learn about each of these stages and what can you do to prepare yourself.
Read Also: Technical Interview Questions For Engineers
Compare Two Arrays Of Objects Remove Duplicates Merge Objects Based On Property
We do get a requirement to compare two different arrays of objects and want to merge both objects if they match specific property values. It can be achieved using the filter method.
The filter method creates a new array with all elements that pass the test implemented by the provided function.
Lets create test data.
let array1 = let array2 =
Lets create functions.
}) => === )) console.log) //
What Are Classes In Javascript
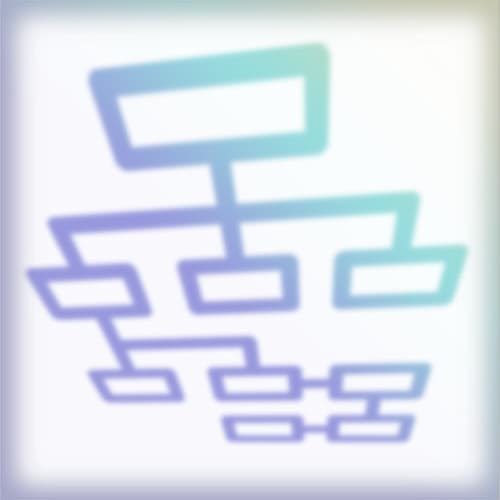
Introduced in the ES6 version, classes are nothing but syntactic sugars for constructor functions. They provide a new way of declaring constructor functions in javascript. Below are the examples of how classes are declared and used:
// Before ES6 version, using constructor functionsfunctionStudent// Way to add methods to a constructor functionStudent.prototype.getDetails = function, Roll no: $, Grade: $, Section:$' }let student1 = new Student student1.getDetails // Returns Name: Vivek, Roll no:354, Grade: 6th, Section:A// ES6 version classesclassStudent// Methods can be directly added inside the classgetDetails, Roll no: $, Grade:$, Section:$' }}let student2 = new Student student2.getDetails // Returns Name: Garry, Roll no:673, Grade: 7th, Section:C
Key points to remember about classes:
- Unlike functions, classes are not hoisted. A class cannot be used before it is declared.
- A class can inherit properties and methods from other classes by using the extend keyword.
- All the syntaxes inside the class must follow the strict mode of javascript. An error will be thrown if the strict mode rules are not followed.
Recommended Reading: How To Pass An Amazon Interview
Wrapping Up And Resources
Congratulations! As with every other discipline, you can only get better at identifying and implementing design patterns in JavaScript by practicing frequently. With a firm grasp of these patterns, you will fare better in future interviews. Consistent practice is the key!
To get you started, I recommend Educatives course JavaScript Design Patterns for Coding Interviews, which will adequately prepare you for all the aspects of an interview process.
This course is designed around the main design patterns. By the end of this course, youll confidently use design patterns to tackle advanced coding interview questions!
Master The Coding Interview: Data Structures + Algorithms
This Udemy bestseller is one of the highest-rated interview preparation course and packs 19 hours worth of contents into it. Like Tech Interview Handbook, it goes beyond coding interviews and covers resume, non-technical interviews, negotiations. It’s an all-in-one package! Note that JavaScript is being used for the coding demos. Check it out
Don’t Miss: Google Senior Engineering Manager Interview
Advantages Of Closures In Javascript
let add = function }let addByTwo = addaddByTwo
- Data Hiding/Encapsulation
Suppose you want to create a counter application. Every time you call it, the count increases by 1. But you don’t want to expose the variable outside the function. How to do it?
You guessed it closures!
function Counter }console.log // Error: count is not definedvar adder = new Counteradder.incrementCount // 1
Don’t worry about this and new. We have a whole section devoted to them down below.
Architectural Design Pattern: Challenge And Examples
Architectural patterns help build an efficient architecture for an application. Think of these as the source of subsystems with assigned responsibilities, such as guidelines for constructing relationships between subsystems. The MVC Pattern, which stands for model-view-controller pattern, is a very popular architectural pattern for web development that we can use to organize code by splitting application functionality into components. The model component manages the application data. The view renders data on the user-facing parts of the application. The controller connects the model and view components.
Angular and Ember are two examples of JavaScript frameworks implementing the MVC pattern in their design. Its useful when you want to improve application organization in your application or speed up development, since developers can work on different components of the application at the same time. Alright, now lets try to solve a challenge by implementing the MVC pattern.
Recommended Reading: How To Prepare For A Citizenship Interview
What Are The Types Of Errors In Javascript
There are two types of errors in javascript.
What Is The Rest Parameter And Spread Operator
Both rest parameter and spread operator were introduced in the ES6 version of javascript.Rest parameter :
- It provides an improved way of handling the parameters of a function.
- Using the rest parameter syntax, we can create functions that can take a variable number of arguments.
- Any number of arguments will be converted into an array using the rest parameter.
- It also helps in extracting all or some parts of the arguments.
- Rest parameters can be used by applying three dots before the parameters.
functionextractingArgs// extractingArgs // Returns 9functionaddAllArgsreturn sumOfArgs }addAllArgs // Returns 117addAllArgs // Returns 8
**Note- Rest parameter should always be used at the last parameter of a function:
// Incorrect way to use rest parameterfunctionrandomFunc// Correct way to use rest parameterfunctionrandomFunc2
- Spread operator : Although the syntax of the spread operator is exactly the same as the rest parameter, the spread operator is used to spreading an array, and object literals. We also use spread operators where one or more arguments are expected in a function call.
functionaddFourNumberslet fourNumbers = addFourNumbers // Spreads as 5,6,7,8let array1 = let clonedArray1 = // Spreads the array into 3,4,5,6console.log // Outputs let obj1 = let clonedObj1 = // Spreads and clones obj1console.log let obj2 = let mergedObj = // Spreads both the objects and merges itconsole.log // Outputs
Don’t Miss: What Is Excel Test For Interview
Difference Between Prototypal And Classical Inheritance
Programers build objects, which are representations of real-time entities, in traditional OO programming. Classes and objects are the two sorts of abstractions. A class is a generalization of an object, whereas an object is an abstraction of an actual thing. A Vehicle, for example, is a specialization of a Car. As a result, automobiles are descended from vehicles .
Classical inheritance differs from prototypal inheritance in that classical inheritance is confined to classes that inherit from those remaining classes, but prototypal inheritance allows any object to be cloned via an object linking method. Despite going into too many specifics, a prototype essentially serves as a template for those other objects, whether they extend the parent object or not.
The Foreach Array Method
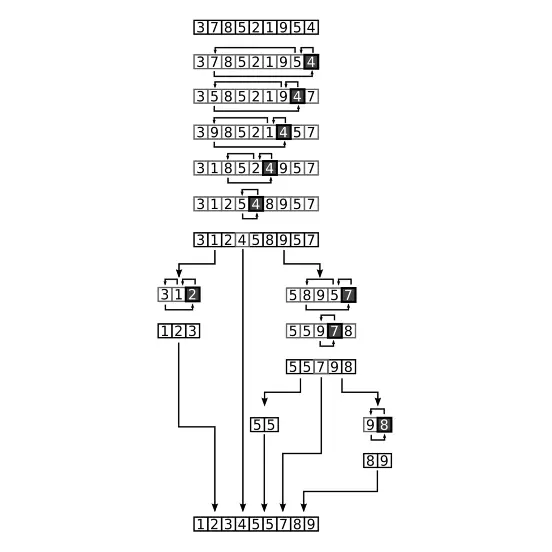
forEach is very similar to map but has two key differences:
First of all, map returns a new Array, but forEach doesn’t.
// Return a new array where even numbers are multiplied by 2 let arr = function consoleEven // ? is the ternary operator. If the condition is true - first statement is returned otherwise the second one.consoleEven
function consoleEven consoleEven
And second, you can do method chaining in map but not in forEach.
// Convert the new array back to originalfunction consoleEven consoleEven
Note:map and forEach don’t mutate the original array.
Read Also: What Are Standard Interview Questions
Why Do We Use Callbacks
A callback function is a method that is sent as an input to another function , and it is performed inside the thisFunction after the function has completed execution.
JavaScript is a scripting language that is based on events. Instead of waiting for a reply before continuing, JavaScript will continue to run while monitoring for additional events. Callbacks are a technique of ensuring that a particular code does not run until another code has completed its execution.
What Are Arrow Functions
Arrow functions were introduced in the ES6 version of javascript. They provide us with a new and shorter syntax for declaring functions. Arrow functions can only be used as a function expression.Lets compare the normal function declaration and the arrow function declaration in detail:
// Traditional Function Expressionvar add = function// Arrow Function Expressionvar arrowAdd = => a + b
Arrow functions are declared without the function keyword. If there is only one returning expression then we dont need to use the return keyword as well in an arrow function as shown in the example above. Also, for functions having just one line of code, curly braces can be omitted.
// Traditional function expressionvar multiplyBy2 = function// Arrow function expressionvar arrowMultiplyBy2 = num => num * 2
If the function takes in only one argument, then the parenthesis around the parameter can be omitted as shown in the code above.
var obj1 = }var obj2 = }obj1.valueOfThis // Will return the object obj1obj2.valueOfThis // Will return window/global object
The biggest difference between the traditional function expression and the arrow function is the handling of this keyword. By general definition, this keyword always refers to the object that is calling the function. As you can see in the code above, obj1.valueOfThis returns obj1 since this keyword refers to the object calling the function.
Recommended Reading: How To Interview At Google
What Is This In Javascript
Now, working with objects is different in JS than in other popular programming languages like C++. And to understand that properly, we need a good grasp of the this keyword.
Let’s try to understand it step-by-step.
In a program, at times, we need a way to point at stuff. Like saying this function right here belongs to this object. this helps us get this context.
You will understand what I am saying better when we look at some examples.
For now, think of this as something which provides context. And remember this important thing: its value depends on how and where it is called.
I know, I know. A lot of this . Let’s go over all this slowly.
Start a new program and just log this.
console.log
It will point to the window object.
Now, let’s take an example with an object:
function myFunc const obj = obj.myFunc
Now, this will point to the object. So what’s happening here?
In the first example, we had nothing left of the . so it defaulted to the window object. But in this example, we have the object obj.
If you do:
myFunc // window
We again get the window object. So, we can see that the value of this depends on how and where are we doing the calling.
What we just did above is called Implicit Binding. The value of this got bound to the object.
There is another way to use this. Explicit binding is when you force a function to use a certain object as its this.
Let’s understand why we need explicit binding through an example.
So, let’s get rid of displayName_2 and simply do:
Learn Ace Your Next Javascript Coding Interview By Mastering Data Structures And Algorithms
If youre nervous about your first coding interview, or anxious about applying to your next job, this is the course for you. I got tired of interviewers asking tricky questions that can only be answered if youve seen the problem before, so I made this course! This video course will teach you the most common interview questions that youll see in a coding interview, giving you the tools you need to ace your next whiteboard interview.
Coding interviews are notoriously intimidating, but there is one method to become a better interviewer– and that is practice! Practicing dozens of interview questions is what makes the difference between a job offer for a $120k USD and another rejection email. This course is going to not only give you dozens of questions to practice on, but it will also make sure you understand the tricks behind solving each question, so youll be able to perform in a real interview.
I have spent many hours combing through interview questions asked at and Amazonto make sure you know how to answer questions asked by the most well-paying companies out there. No stone is left unturned, as we discuss everything from the simplest questions all the way to the most complex algorithm questions.
In this course, youll get:
- Clear, well-diagramed explanations for every single problem to make sure you understand the solution
Don’t Miss: 2nd Interview Questions To Ask Candidates
What Do You Mean By Bom
Browser Object Model is known as BOM. It allows users to interact with the browser. A browser’s initial object is a window. As a result, you may call all of the window’s functions directly or by referencing the window. The document, history, screen, navigator, location, and other attributes are available in the window object.
What Are Design Patterns
A design pattern in software engineering is a general, reusable solution to a commonly occurring problem, such as building JavaScript web applications or designing a common computer system.
Instead of being a ready-to-use solution, a design pattern provides a description or template for how to solve a problem, with applications for varying situations. These patterns allow us to reuse code structure for specific system design problems.
The illustration above shows an example of a commonly used design pattern: the observer pattern. This is useful in systems where changes to an entity can affect other dependent objects , but the dependents have no control over when or how these changes occur. Using a design pattern has many advantages:
- They provide generic templates that can solve a multitude of common problems
- Other developers can build upon a pattern, so less time is wasted on code structure
- Design patterns provide optimized solutions, so they reduce the size of a codebase
- They are the most optimized solution that has been derived from experienced programmers, so you can trust the results and efficiency
For a senior developer coding interview, design questions will be baked into the coding questions you face. There is no separate design interview, so you should be prepared to face system design questions at any stage.
Read Also: Jobs Hiring Walk In Interviews
Explain Weakmap In Javascript
In javascript, Map is used to store key-value pairs. The key-value pairs can be of both primitive and non-primitive types. WeakMap is similar to Map with key differences:
- The keys and values in weakmap should always be an object.
- If there are no references to the object, the object will be garbage collected.
const map1 = newMap map1.set const map2 = newWeakMap map2.set // Throws an errorlet obj = const map3 = newWeakMap map3.set
Async And Defer In Javascript
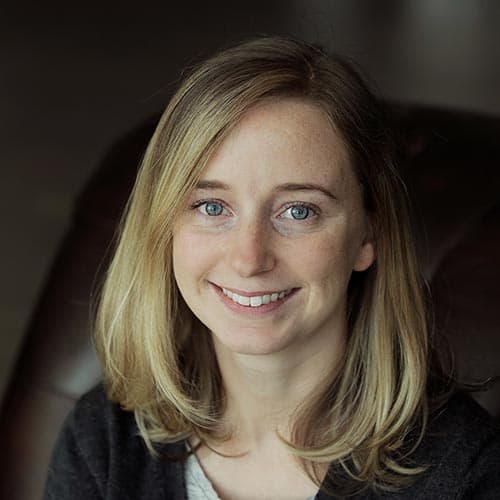
These concepts are frequently asked about in interviews by big corporations like Amazon, Walmart, and Flipkart.
To understand async and defer, we need to have an idea of how browsers render a webpage. First, they parse the HTML and CSS. Then DOM trees are created. From these, a render tree is created. Finally, from the render tree – a layout is created and the painting happens.
For a more detailed look, check out this video.
Async and defer are boolean attributes which can be loaded along with the script tags. They are useful for loading external scripts into your web page.
Let’s understand with the help of pictures.
If there are multiple scripts which are dependant on each other, use defer. Defer script are executed in the order which they are defined.
If you want to load external script which is not dependant on the execution of any other scripts, use async.
Note: The async attribute does not guarantee the order of execution of scripts.
You May Like: How To Record Video Interview
Structural Design Pattern: Questions And Examples
As we previously discussed, these patterns deal with object relationships and the structure of objects. With structural patterns, you can add functionalities to objects so that restructuring some parts of the system does not affect the rest. Structural patterns ease the process of system design and allow for flexibility when assembling large structures from objects or classes. We will be looking at the decorator pattern as an example of structural patterns in this section.
The Decorator Pattern is a structural pattern that allows behavior to be added to an individual object dynamically without affecting the behavior of other objects from the same class. Its useful in applications that have many distinct objects with the same underlying code. Instead of creating all of them using different subclasses, additional functionalities can be added to the objects using the decorator pattern.
Lets take a look at this in code to better grasp the concept.
class Text {const text = new Text const heading = applyHeadingStyles console.log console.log
This is a simple example using text formatting. Here, we create a new Text object with a value property. Next, we have two decorators which can change the appearance of the text, either by applying a set of styles to make it appear as a heading or by changing its font. These decorators can be applied later but are not needed in the creation of the text. Now, lets look at another example.