Prepare Questions For The Interviewer
Interviewers often appreciate it when the candidate asks questions about the role, so they may leave time for this at the end of an interview. This makes it a good idea to prepare some questions before going into your interview. For example, you can ask questions about the role, the company or what the employer expects of you.
Related: 63 Thoughtful Questions To Ask During an Interview
What Is A Delegate
It is the definition of a method that encapsulates certain arguments and types of return. It allows passing a method as an argument of a function, as long as it matches its specific signature.
var fileDownloader = new FileDownloader fileDownloader.DownloadFile void PrintDownloadCompletedpublic class FileDownloader}
Q25 List The Difference Between The Virtual Method And The Abstract Method
Ans- A Virtual method must always have a default implementation. However, it can be overridden in a derived class by the keyword override.
An Abstract method doesnt have any implementation. It resides in the abstract class, and also it is mandatory that the derived class must implement abstract class. Use of override keyword is not necessary.
Don’t Miss: What Do They Ask In Exit Interviews
Give An Example Of Removing An Element From The Queue
The dequeue method is used to remove an element from the queue.
using System using System.Collections using System.Collections.Generic using System.Linq using System.Text using System.Threading.Tasks namespace DemoApplication Console.WriteLine Console.WriteLine Console.WriteLine Console.WriteLine) Console.ReadKey } }}
These interview questions will also help in your viva
Fundamental C# Programming Interview Questions
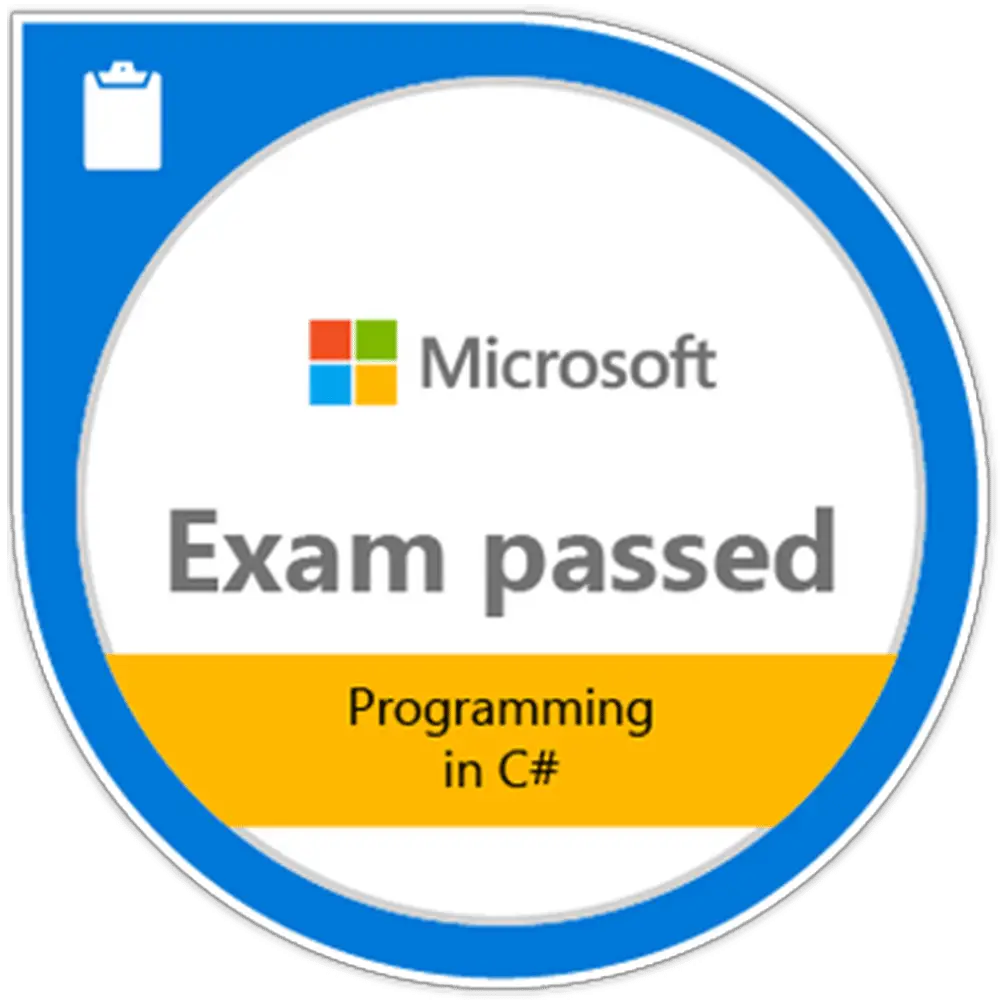
In any C# programming interview, you are bound to be thrown a few basic questions about the C# language. Any programmer will have good general knowledge about their language.
However, if you dont get the answers 100% correct, dont sweat because it is still possible to do well in your interview if you can demonstrate that you have a general understanding of the language at a high level.
Here are some basic C# programming interview questions that you could encounter during an interview.
Don’t Miss: What Questions To Ask When Interviewing Someone For A Job
What Are Delegates And Its Types In C#
Delegates are type-safe objects containing information about the function rather than data. They can define their own type parameters
Below are the types of delegates in C#.
What Are Nullable Types
Value types that can accept a normal value or a null value are referred to as Nullable types. These are instances of the Nullable struct. For example, A Nullable< bool> is pronounced as “Nullable of bool” and can have the values true, false, or null. Nullable types can also be defined using the ? type modifier. This token is placed immediately after the value type is defined as nullable.
//assigning normal value Nullable< bool> flag = true //Or You can also define as bool? flag = true //assigning normal value Nullable< int> x = 20 //Or You can also define as int? x = 20
Note
You should not declare a string as nullable since it is implicitly nullable. Hence, declaring a string type like string? firstName causes a compile-time error.
Read Also: How Do You Handle Conflict Interview Question
How Does Linq Work
Internally build the correct query or generate the corresponding operations on the collections or parse the XML and returns the relevant data. It encapsulates all these behaviors and provides a single implementation. In this way, we can use the same queries, and the same language, independently of the underlying data source.
Recommended Reading: How To Interview Someone For A Job Questions
What Are Partial Classes In C#
Partial classes implement the functionality of a single class into multiple files. These multiple files are combined into one during compile time. The partial class can be created using the partial keyword.
publicpartial Clas_name
You can easily split the functionalities of methods, interfaces, or structures into multiple files. You can even add nested partial classes.
You May Like: Free Online Training For Motivational Interviewing
Predict The Output Of The Code Below
delegate void Iterator static void Main) } Foreach }
This program tests the prospective developer on their experience working with loops and delegates. At first glance, one would expect the program to output the numbers 0 to 15, fifteen times. Instead, the number 15 is printed fifteen times. Since the delegate is being added within the for loop, and because the delegate is only referencing the variable i instead of the value itself, the loop sets the value of the variable i to 15 before it is invoked within each delegate.
Discuss The Various Methods To Pass Parameters In A Method
The various methods of passing parameters in a method include –
- Output parameters: Lets the method return more than one value.
- Value parameters: The formal value copies and stores the value of the actual argument, which enables the manipulation of the formal parameter without affecting the value of the actual parameter.
- Reference parameters: The memory address of the actual parameter is stored in the formal argument, which means any change to the formal parameter would reflect on the actual argument too.
Read Also: Big Data Analytics Interview Questions
List The Differences Between Static Public And Void
Static Its a keyword which declares the Main method as the global one and can be called without creating a new instance of the class.
Public Its an access modifier keyword which tells the compiler that anyone can access the Main method.
Void Its a type modifier keyword which states that the Main method doesnt return any value.
How Are User Controls Created In C#

Sample answer:
In C#, user controls allow developers to write code that can be used in various areas of the program.
For example, if a website requires the same search control in multiple places, it can be created once as a user control and then dropped into different areas of the code. This serves the dual purposes of reusability and bug prevention.
Read Also: Where Can I Go For Tsa Precheck Interview
Some General Interview Questions For C#
1. How much will you rate yourself in C#?
When you attend an interview, Interviewer may ask you to rate yourself in a specific Technology like C#, So It’s depend on your knowledge and work experience in C#.
2. What challenges did you face while working on C#?
This question may be specific to your technology and completely depends on your past work experience. So you need to just explain the challenges you faced related toC# in your Project.
3. What was your role in the last Project related to C#?
It’s based on your role and responsibilities assigned to you and what functionality you implemented using C# in your project. This question is generallyasked in every interview.
4. How much experience do you have in C#?
Here you can tell about your overall work experience on C#.
5. Have you done any C# Certification or Training?
It depends on the candidate whether you have done any C# training or certification. Certifications or training are not essential but good to have.
We have covered some frequently asked C# Interview Questions and Answers to help you for your Interview. All these Essential C# Interview Questions are targeted for mid level of experienced Professionals and freshers. While attending any C# Interview if you face any difficulty to answer any question please write to us at . Our IT Expert team will find the best answer and will update on the portal. In case we find any new C# questions, we will update the same here.
What Is The Difference Between Iapplicationbuilderuse And Iapplicationbuilderrun
We can use both the methods in Configure methods of the startup class. Both are used to add middleware delegates to the application request pipeline. The middleware adds using IApplicationBuilder.Use may call the next middleware in the pipeline whereas the middleware adds using IApplicationBuilder.The run method never calls the subsequent middleware. After IApplicationBuilder.Run method, system stop adding middleware in the request pipeline.
You May Like: What To Say During An Interview
What Are The Differences Between Object And Var
A: The introduction of object was done with C# 1.0, while for Var it was C# 3.0. You can use object when you want to store several types of values in a single variable while Var is used when you are not sure about the type of assigned value. Object can be passed as a method argument, while the same cannot be done with Var.
Write A C# Method To Total All The Even Numbers In An Array Of Ints
This is an open-ended coding question that is likely to produce a variety of answers. What youre really looking for is how the developer chooses to solve the problem. Do they settle for the obvious one-liner, return intArray.Where.sum or will they notice the high probability of overflow and instead opt for something more nuanced like the sample answer below?
static long TotalAllEvenInts
Experienced C# developers will take this as an opportunity to show off their knowledge of C# language constructs that make simple solutions like the one above possible.
Also Check: Product Manager Interview Case Study Presentation
Differentiate Between Task And Thread In Net
Ans: The thread represents an actual OS-level thread, with its own stack and kernel resources, and allows the highest degree of control. You can choose to Abort or Suspend or Resume a thread, and set thread-level properties, like the stack size, apartment state, or culture. While a Task class from the Task Parallel Library is executed by a TaskScheduler to return a result and allows you to find out when it finishes.
What Is A Constructor And What Are Its Different Types
A constructor is like a method with the same name as the class, but it is a unique method. Even if it is not created, the compiler creates a default constructor in memory at the time of creating an object of the class.
The constructor is used to initialize the object with some default values.
Default constructor, parameterized constructor, copy constructor, static constructor, and private constructor are all different constructor types.
Below are examples of different constructor types.
public class Student private string fullName //default constructor public Student //parameterized constructor public Student //static constructor static Student //copy constructor public Student }
Read Also: How To Answer Retail Interview Questions
C# Coding Interview Question For Practice
You should be well-versed in data structures and algorithms to ace the C# coding problems in technical interview rounds.
String Calculator A Great C# Programming Problem

There is a place for a more detailed assessment of a potential recruits skills in any set of C# programming interview questions. This can involve asking them to complete a programming task that can then be discussed at length.
The following problem is a coding kata created by Roy Osherove. Its designed to be daily unit testing practice but also makes a wonderful interview question because it can be worked on in stages and all design choices discussed. A developer should be able to code a solution to this problem in 30 to 60 minutes.
Create a String Calculator by performing the following tasks in order and one at a time.
- The string of numbers will be a maximum of two numbers separated by commas
- The method will return their sum
Examples of input:
Examples of input:1,\n is invalid but you dont need to handle this scenario.
- The custom delimiter will be specified at the beginning of the string of numbers in a special format.
- The basic format of the string of numbers will be //\n[numbers
- The custom delimiter is optional. If not present, comma and newline will be the default delimiters.
Example of input:// \n1 2 returns 3
Read Also: How To Prepare For A Machine Learning Interview
What Are User Control And Custom Control
Custom Controls are produced as compiled code. These are easy to use and can be added to the toolbox. Developers can drag and drop these controls onto their web forms. User Controls are almost the same as ASP include files. They are also easy to create. User controls, however, cant be put in the toolbox. They also cant be dragged and dropped from it.
What Are Custom Control And User Control
Custom Controls are controls generated as compiled code , those are easier to use and can be added to toolbox. Developers can drag and drop controls to their web forms. Attributes can, at design time. We can easily add custom controls to Multiple Applications . So, If they are private, then we can copy to dll to bin directory of web application and then add reference and can use them.
User Controls are very much similar to ASP include files, and are easy to create. User controls cant be placed in the toolbox and dragged dropped from it. They have their design and code-behind. The file extension for user controls is ascx.
Recommended Reading: How To Land A Job Interview
What Are A Class And An Object
Employers want to see that candidates not only know when to use different C# elements but can explain their fundamental differences. This demonstrates a deep understanding of the programming language. You can answer this question by defining both elements and how they relate to one another.
Example: “A ‘class’ is an encapsulation of methods and properties that programmers use to represent an entity in real time. Class brings all the instances together in a single unit. An ‘object’ is an instance of a class, or a block of allocated memory you can store in the form of variables, arrays or a collection.”
Related: 10 Steps To Get a Programming Job With No Experience
What Are The Different Types Of Operators In C#
A: There are a total of seven operators in C#, which include:
- Arithmetic Operators: These are the kind of operators that are used for performing arithmetic operations.
- Relational Operators: These operators are used for comparing values. These always result in true or false .
- Logical/Boolean Operators: These operators are also used for comparing values. These also always result in true or false .
- Bitwise Operators: These operators perform a bit-by-bit operation.
- Assignment Operators: These operators help in assigning a new value to the variable.
- Type Information Operators: These operators provide information about a certain type.
- Miscellaneous Operators: ?: , => , & , & & , *
Recommended Reading: How To Ask Behavioral Interview Questions
Explain Oop And Its Relation To The Net Framework
Ans: OOP is the acronym for Object-Oriented Programming. It is a programming structure that uses self-contained entities called objects instead of methods to achieve the desired functionality. OOP allows .NET developers to create modular programs and classes containing methods, properties, fields, events, and other logical modules.
Q50 What Is Thread Pooling
Ans- A Thread pool is a collection of threads that perform tasks without disturbing the primary thread. Once the task is completed by a thread it returns to the primary thread.
This brings us to the end of this article on C# Interview Questions. I hope it helped in adding up to your knowledge. Wishing you all the best for your interview. Happy learning.
In case you are looking for the most common Technical Interview Questions, read along:
You May Like: How To Prepare For A Big Interview
Learn To Relax While Under Pressure During Interviews
Everyone feels nervous when their livelihood is on the line. However, these emotions can get in your way if you dont get them under control. Ive almost lost jobs when Ive let my emotions get in my way. Ive also aced interviews when I went into them with an I dont care if I get this job mindset.
Before you set foot into the interviewing crucible, take some time to learn how to relax under pressure. It will make a world of difference and allow you to ace any programming interview.
Here are a few ways that can make a massive impact:
- Meditation Learn how to return to a place of calm by focusing on your breathing. Even 10 minutes of focused practice can give you an edge.
- Visualization Mentally walk through the entire interview process and how you will respond. This includes going over previous interviews and refining your responses until they flow naturally.
- Practice interviewing with a friend If you have a developer friend who would be willing to give you a hand, have them ask you C# programming interview questions to test your knowledge. Youll be able to gain valuable feedback and test your knowledge at the same time.
- Practice real interviewing Has it been a while since you last interviewed for a position? If you dont interview for positions on a regular basis you can get a bit rusty. The best way to avoid this trap is to interview for positions regularly even if youre happy with your current position. You never know, you may get an offer you cant refuse.