What Do You Understand By Immutability Of Java String Objects
Strings in Java programming language are immutable, i.e. once a string object is created its value cannot be changed. When you change the value of a string reference variable, internally the java virtual machine creates a new string in memory and returns that value. The old string still exists in memory but is not being referenced.
//create new string 'abc'
How Do You Reverse The Words In A String
You are given a string. You have to reverse the words in the string. Let’s say the string is ‘interviewgrid.com is awesome’. You have to write a function that takes this string as input and prints ‘awesome is interviewgrid.com’. Assume that the words are separated by a single space.
Your approach to this function could be as follows.
1. Create a new StringBuilder object that will store the output string.2. Tokenize the string into tokens, i.e. words, with single space as delimiter.3. …
*** See complete answer and code snippet in the Java Interview Guide.
Question 1: Find Minimum Number Of Platforms Required For Railway Station
You are given arrival and departure time of trains reaching to a particular station. You need to find minimum number of platforms required to accommodate the trains at any point of time.
For example:
arrival=departure=No.of platforms required inabove scenario=4 |
Solution :
You May Like: What Is A Good Thank You Email After An Interview
Q 68 Could You Provide Some Implementation Of A Dictionary Having A Large Number Of Words
The simplest implementation that can be given is that of a List wherein one can place ordered words and perform a Binary search. The other implementation with a better search performance is HashMap where the key is used as the first character of the word and the value as a LinkedList.
Up another level, there are HashMaps like:
hashmap {
a -> hashmap
b -> hashmap
z -> hashmap
Up to n levels where n is the average size of the word in the dictionary.
Q8 What Is The Difference Between Continue And Break Statement

Ans: break and continue are two important keywords used in Loops. When a break keyword is used in a loop, loop is broken instantly while when continue keyword is used, current iteration is broken and loop continues with next iteration.
In below example, Loop is broken when counter reaches 4.
for system.out.println if }
In the below example when counter reaches 4, loop jumps to next iteration and any statements after the continue keyword are skipped for current iteration.
for system.out.println if system.out.println }
Also Check: How To Pass Any Job Interview
Q How To Create Marker Interface
An interface with no methods is known as marker or tagged interface. It provides some useful information to JVM/compiler so that JVM/compiler performs some special operations on it. It is used for better readability of code. Example: Serializable, Clonnable etc.
Syntax:
publicinterfaceInterface_Name
Example:
/*** Java program to illustrate Maker Interface ***/interfaceMarker classAimplementsMarker classMain }}
What Are Generic Types
A generic type is a generic class or interface that is parameterized over types.
– A generic class is declared using the format ‘class MyClass ‘. The angle brackets < > is the type parameter section and specifies the type parameters T1, T2 … for the generic class.
Instantiation of generic types – You instantiate a generic class using the new keyword as usual, but pass actual types within the parameter section. Example – ‘MyClass myClass = new MyClass ‘. In Java 7 and later you can eliminate the type and just have the angle brackets < > . Example – ‘MyClass myClass = new MyClass< > ‘. The empty angular brackets < > syntax is commonly referred to as the Diamond.
Read Also: How To Excel In A Job Interview
Interfaces And Abstract Classes Java Interview Questions
27. What do you know about Interfaces?
A Java interface is a template that has only method declarations and not method implementations. It is a workaround for achieving multiple inheritances in Java. Some worth remembering important points regarding Java interfaces are:
- A class that implements the interface must provide an implementation for all methods declared in the interface.
- All methods in an interface are internally public abstract void.
- All variables in an interface are internally public static final.
- Classes do not extend but implement interfaces.
28. How is an Abstract class different from an Interface?
There are several differences between an Abstract class and an Interface in Java, summed up as follows:
29. Please explain Abstract class and Abstract method.
An abstract class in Java is a class that can’t be instantiated. Such a class is typically used for providing a base for subclasses to extend as well as implementing the abstract methods and overriding or using the implemented methods defined in the abstract class.
To create an abstract class, it needs to be followed by the abstract keyword. Any abstract class can have both abstract as well as non-abstract methods. A method in Java that only has the declaration and not implementation is known as an abstract method. Also, an abstract method name is followed by the abstract keyword. Any concrete subclass that extends the abstract class must provide an implementation for abstract methods.
Explain In Brief The History Of Java
In the year 1991, a small group of engineers called Green Team led by James Gosling, worked a lot and introduced a new programming language called Java. This language is created in such a way that it is going to revolutionize the world.
In todays World, Java is not only invading the internet, but also it is an invisible force behind many of the operations, devices, and applications.
Recommended Reading: How To Send A Thank You For The Interview Email
You Have To Search The Element In O Time Complexity Without Using Any Extra Space Print True If The Element Exists In The Matrix Else Print False
The normal searching technique will take O time complexity as we will search every element in the matrix and see if it matches our target or not. The other approach uses the fact that the elements are sorted row-wise. We can apply binary search on every row. Hence, the time complexity will be OSince we want the solution in O, this approach is not the one we will use. We will use the Staircase Search Algorithm. See, we know that the elements are sorted column-wise and row-wise. So, we start from the last element of the first row as shown below
Let us say we want to search for 21. We know that 21 is larger than 15. Since the matrix is row-wise sorted, element 15 is the largest element of this row. So, we are not going to find 21 in this row. So, we move directly to the last element of the next row. The Same is the case here as well. So, we move to the next row. Here, the element is 22. So, 21 might be present in this row. So, we move one step backwards in this row only.
On moving one step backwards, we see that we reach 16. Since this number is smaller than our target of 21, we know that we will not find our target in this row. Hence, we move to the last element of the next row and the same happens here too. Now, we are in the last row. We know that element might exist in this row. So, we keep on moving back in this row and find element 21.
So, we will implement this same algorithm. This is called staircase search.
Java Code for Staircase Search
Sample Output
Popular Java Programming Interview Questions
Prog_1. Write a program to checkwhether a number is prime or not?Prog_2. Write a program to findFibonacci series for a given range.Prog_3. Write a program to checkwhether a given string is palindrome or not?Prog_4. Write a program to checkwhether a number is Armstrong number?Prog_5. Write a program to findduplicates in an array.Prog_6. Write a program to findduplicate character in a string.Prog_7. Write a program to check the number of times substring appeared in a string.Prog_8.Write a program to print the below pattern:Prog_9.Write a program to print the below pattern:Prog_10. Write a program to print Floyds triangle.
Author: Ankur Jain
My Name is Ankur Jain and I am currently working as Automation Test Architect.I am ISTQB Certified Test Manager,Certified UI Path RPA Developer as well as Certified Scrum Master with total 12 years of working experience with lot of big banking clients around the globe.I love to Design Automation Testing Frameworks with Selenium,Appium,Protractor,Cucumber,Rest-Assured, Katalon Studio and currently exploring lot in Dev-OPS as well.I am currently staying in Mumbai, Maharashtra. Please Connect with me through Contact Us page of this website.
Some simillar article from this label, you might also like
You May Like: How To Properly Answer Interview Questions
Top 80 Java Interview Questions And Answers
It’s a no-brainer that Java is one of the leading programming options for bagging a lucrative job. After all, the class-based, general-purpose, object-oriented programming language is one of the most widely used programming languages in the world.
With a plethora of great features, the programming language is preferred not only by seasoned experts but also pursued by those new to the programming world. Here are the top Java interview questions and answers that will help you bag a Java job or, at the very least, enhance your learning.
These Java interview questions are recommended for both beginners and professionals as well as for Software Developers and Android Applications Developers.
What Are The I/o Streams Java Programming Language
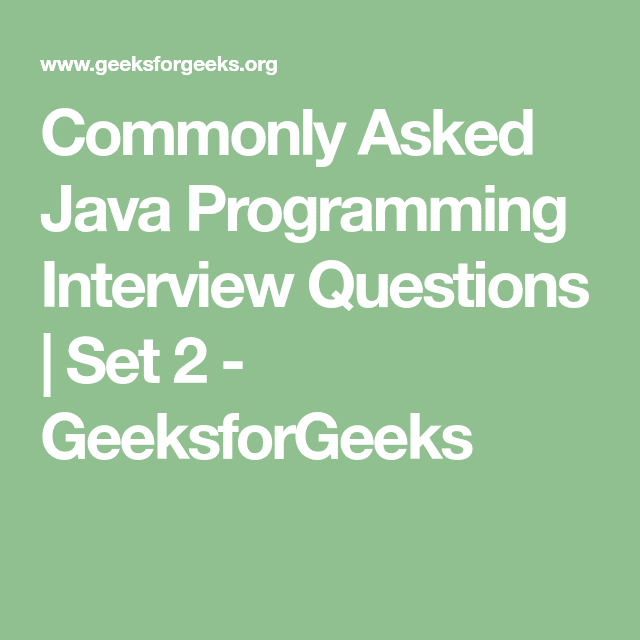
I/O streams in Java programming language represent input sources from which data is read, and output destinations to which data is written. Streams support different kinds of data including bytes, characters, primitive types and objects.
– java.io package has two abstract classes InputStream and OutputStream that represents input stream and output stream of byte data type.
Character Streams – java.io package has two abstract classes Reader and Writer that represents input stream and output stream of character data type.
Primitive data streams– java.io package has two interfaces classes DataInput and DataOutput that represents input stream and output stream of primitive data type.
Object streams– java.io package has two interfaces ObjectInput and ObjectOutput that represents input stream and output stream of object data type.
Read Also: What Is The Food Stamp Interview Number
What Are The Different Wrapper Classes That Act On Collections
Java programming language provides wrapper functionality that adds additional functionality on top of that of collection classes. Wrapper functionality follows the decorator design pattern. Implementations of these wrapper methods are defined in the java.utils.Collections class.
Three main categories of wrappers are provided in the java.utils.Collections class. Synchronization wrappers, Unmodifiable wrappers and Checked Interface wrappers…
*** See complete answer and code snippet in the Java Interview Guide.
Java Interview Guide has over 250 REAL questions from REAL interviews. Get the guide for $15.00 only.
Following are some frequently asked questions in Java – Lambda Expressions
Q Distinguish Between Static Loading And Dynamic Class Loading
1. Static Class Loading:
Creating objects and instance using new keyword is known as static class loading. The retrieval of class definition and instantiation of the object is done at compile time.
classTestClass }
2. Dynamic Class Loading:
Loading classes use Class.forName method. Dynamic class loading is done when the name of the class is not known at compile time.
Class.forName
Recommended Reading: Learn Data Structures And Algorithms For Interviews
What Are The Core Implementation Classes Of Queue Interface Defined In Java Collections Framework
Java collections framework provides classes LinkedList and PriorityQueue which are implementations of the Queue interface. In addition the java.util.concurrent package provides classes LinkedBlockingQueue, ArrayBlockingQueue, PriorityBlockingQueue, DelayQueue, SynchronousQueue…
*** See complete answer and code snippet in the Java Interview Guide.
Q18 What Is A Constructor Overloading In Java
In Java, constructor overloading is a technique of adding any number of constructors to a class each having a different parameter list. The compiler uses the number of parameters and their types in the list to differentiate the overloaded constructors.
class Demopublic Demo}
In case you are facing any challenges with these java interview questions, please comment on your problems in the section below. Apart from this Java Interview Questions Blog, if you want to get trained from professionals on this technology, you can opt for a structured training from edureka!
You May Like: What Kind Of Questions Do You Ask In An Interview
Give Examples For Both A Default Constructor And A Parameterized Constructor
An example of a default constructor is as follows:
//Java Program to create and call a default constructor class Mindmajix1 //main method public static void main }
Output: Welcome to Mindmajix
An example of the parameterized constructor is as follows:
//Java Program to demonstrate the use of the parameterized constructor. class Training //method to display the values void display public static void main }
Output:
Q7 What Are The Differences Between Servletcontext Vs Servletconfig
The difference between ServletContext and ServletConfig in Servlets JSP is in below tabular format.
ServletConfig | |
---|---|
Servlet config object represent single servlet | It represent whole web application running on particular JVM and common for all the servlet |
Its like local parameter associated with particular servlet | Its like global parameter associated with whole application |
Its a name value pair defined inside the servlet section of web.xml file so it has servlet wide scope | ServletContext has application wide scope so define outside of servlet tag in web.xml file. |
getServletConfig method is used to get the config object | getServletContext method is used to get the context object. |
for example shopping cart of a user is a specific to particular user so here we can use servlet config | To get the MIME type of a file or application session related information is stored using servlet context object. |
Recommended Reading: Sql And Python Interview Questions
Q The Difference Between Inheritance And Composition
Though both Inheritance and Composition provides code reusablility, main difference between Composition and Inheritance in Java is that Composition allows reuse of code without extending it but for Inheritance you must extend the class for any reuse of code or functionality. Inheritance is an “is-a” relationship. Composition is a “has-a”.
Example: Inheritance
classFruit classAppleextendsFruit
Example: Composition
Describe The Java Security Architecture
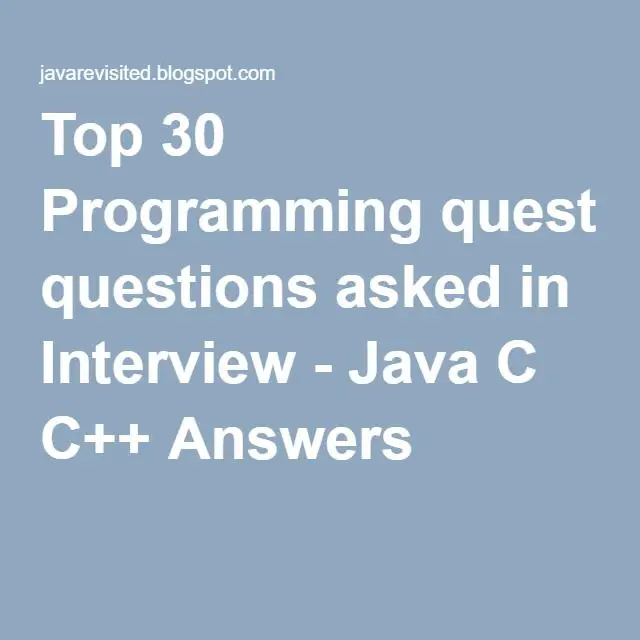
Security is provided by the Java platform through a number of mechanisms.
Secure environment – Java programs run in a secure and restricted environment. The level of access that a Java program can have to important system resources can be restricted based on the trustfulness of the program
Java language features – Java programming language provides a number of in-built features such as automatic memory management, garbage collection array and string range checking etc. which enhances the security of a Java application.
JVM features JVM is designed to provide secure environment for Java applications to be run in – JBytecode verifiers ensure that only legitimate and valid Java bytecodes are executed by the JVM. Java class loaders ensure that only legitimate and secure Java class files, which do not interfere with the running of other Java programs are loaded into the JVM. Access to important resources is provided through the JVM, and is pre-checked by SecurityManager class to ensure that access or restrictions of a resource to a specific resource.
Plugins – Additional security features can be plugged in into the platform and used by Java programs.
You May Like: Python Interview Questions For Devops
What Are Functional Interfaces
Functional interfaces are Java interfaces which have only one declared method. Functional interfaces can have other implemented methods such as default methods and static methods. Some of the common functional interfaces in Java are Runnable, Comparable, ActionListner etc.
Functional interfaces are generally implemented as inner classes and anonymous inner classes, which results in bulky code with many lines. This is sometimes referred to as ‘Vertical’ problem.
Lambda expressions are used to implement functional interfaces which define a single method. Lambda expressions avoid the vertical problem by simplifying the code of the traditional inner classes and anonymous inner classes.
Q What Is Classloader In Java What Are Different Types Of Classloaders
The Java ClassLoader is a part of the Java Runtime Environment that dynamically loads Java classes into the Java Virtual Machine. Java code is compiled into class file by javac compiler and JVM executes Java program, by executing byte codes written in class file. ClassLoader is responsible for loading class files from file system, network or any other source.
Types of ClassLoader:
a) Bootstrap Class Loader:
It loads standard JDK class files from rt.jar and other core classes. It loads class files from jre/lib/rt.jar. For example, java.lang package class.
b) Extensions Class Loader:
It loads classes from the JDK extensions directly usually JAVA_HOME/lib/ext directory or any other directory as java.ext.dirs.
c) System Class Loader:
It loads application specific classes from the CLASSPATH environment variable. It can be set while invoking program using -cp or classpath command line options.
You May Like: How To Prepare For A Phone Call Interview
How Long Do Primitive Variables Exist In Memory Define The Various Scopes Of Primitive Variables
After a primitive variable is declared and initialized how long it lives in memory is dependent on the scope of the variable. Scope of a variable is determined based on where it is declared within a java class. Following are the various scopes of a variable in a java program based on where they are declared.1. Class variable – Class variables are variables declared within the class body, outside of any methods or blocks, and declared with ‘static’ keyword. Class variables have the longest scope. They are created when the class is loaded, and remain in memory as long as the class remains loaded in JVM.2. Instance variables – Instance variable are variables declared within the class body, outside of any method or block, and declared without ‘static’ keyword. Instance variables have the second highest scope. Instance variables are created when a new class instance is created, and live until the instance is removed from memory…
*** See complete answer and code snippet in the Java Interview Guide.