How Do You Identify Missing Values And Deal With Missing Values In Dataframe
Identification:
isnull and isna functions are used to identify the missing values in your data loaded into dataframe.
missing_count=data_frame1.isnull.sum
There are two ways of handling the missing values :
Replace the missing values with 0
df.fillna
Replace the missing values with the mean value of that column
df = df.fillna))
What Are Some Of The Most Commonly Used Built
Python modules are the files having python code which can be functions, variables or classes. These go by .py extension. The most commonly available built-in modules are:
"abdc1321".isalnum #Output: True"xyz@123$".isalnum #Output: False
Another way is to use match method from the re module as shown:
import reprint)) # Output: Trueprint)) # Output: False
Additional Situational Interview Questions For Python Developers
What do you do if theres a disagreement within your team? |
Are you comfortable giving in-depth presentations? |
Are you able to explain complex technologies in simple terms? |
What is your preferred way of communication with the team? |
How do you convince someone to agree with you? |
How to deal with a team member who disagrees with you? |
Have you ever worked directly with clients or have been in a customer-facing role in the past? If not, would you like to? |
What was the last presentation you gave? |
What are the qualities of a successful team or project leader? |
Recommended Reading: How To Prepare Resume For Interview
What Is Type Conversion In Python
Python provides you with a much-needed functionality of converting one form of data type into the needed one and this is known as type conversion.
Type Conversion is classified into types:
1. Implicit Type Conversion: In this form of type conversion python interpreter helps in automatically converting the data type into another data type without any User involvement.
2. Explicit Type Conversion: In this form of Type conversion the data type inn changed into a required type by the user.
Various Functions of explicit conversion are shown below:
int function converts any data type into integer.float function converts any data type into float.ord function returns an integer representing the Unicode characterhex function converts integers to hexadecimal strings.oct function converts integer to octal strings.tuple function convert to a tuple.set function returns the type after converting to set.list function converts any data type to a list type.dict function is used to convert a tuple of order into a dictionary.str function used to convert integer into a string.complex function used to convert real numbers to complex numbers.
Python/django Developer Interview Questions And Answers
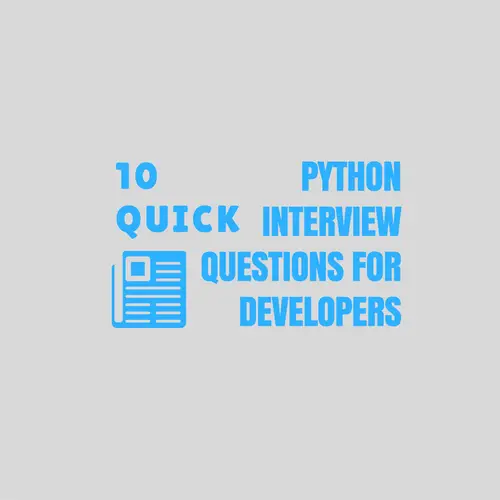
All the questions asked in my interview for a Web Developer role focusing on Python and Django. Backend Engineer | Software Engineer
Most of the software engineers are intimidated by technical rounds and I have recently started to give interviews. So, this time I am planning to write up all the questions I get asked in my technical rounds. Hoping this will be helpful to some of you to get an idea on what type of questions are being asked. Use this series as a guide to prepare and ace that interview.
Some questions might be specific to Python and Django but most of them will be applicable to any software engineering role and web application developers.
This interview was for a Python developer role and they were looking for a candidate proficient in Django too, hence you will be seeing many questions related to Django architecture and design as well.
1.Lets work on a recursive implementation of Fibonacci series.Passed n as a parameter, return the fibonacci value at the nth position.
Solution: Fibonacci series are created in one of the two ways, iterative and recursive. Interviewer was looking for a recursive solution only.
def fibonacci: if n == 0 or n == 1: return n
This solution worked fine for smaller numbers, n=10, took 0:00:00.001004 sec
However, for n=35, it took 0:00:05.157989 sec, which is too long.So next I was asked to optimize this.
@decorator is just a syntactic sugar, it is equivalent to res = decorator)
4. What is WSGI and UWSGI?
Recommended Reading: Office Assistant Interview Questions To Ask
How Are Arguments Passed By Value Or By Reference In Python
- Pass by value: Copy of the actual object is passed. Changing the value of the copy of the object will not change the value of the original object.
- Pass by reference: Reference to the actual object is passed. Changing the value of the new object will change the value of the original object.
In Python, arguments are passed by reference, i.e., reference to the actual object is passed.
defappendNumber: arr.appendarr = print #Output: => appendNumberprint #Output: =>
Questions On Design Patterns And Etl Concepts
In large applications, youll often use more than one type of database. In fact, its possible to use PostgreSQL, MongoDB, and Redis all within just one application! One challenging problem is dealing with state changes between databases, which exposes the developer to issues of consistency. Consider the following scenario:
Now, youve got yourself an inconsistent and outdated result! The results returned from the second database wont reflect the updated value in the first one. This can happen with any two databases, but its especially common when the main database is a NoSQL database, and information is transformed into SQL for query purposes.
Databases may have background workers to tackle such problems. These workers extract data from one database, transform it in some way, and load it into the target database. When youre converting from a NoSQL database to a SQL one, the Extract, transform, load process takes the following steps:
Read Also: What Questions Do You Have For Me Interview
Explain The Difference Between Shallow And Deep Copy When Using Python
This question will test the candidates deep understanding of the coding language. They should explain both the basic and advanced features of Python. Pay attention to how well the candidates can explain complex Python features and terminologies. Remember, communication skills are as critical as technical skills in a software development team.
Sample answer:
In Python, both are commands to copy objects. What makes them different is that Shallow Copy, while faster, only creates a second variable that references the original. If you make changes to either the original, the copy will reflect the said changes and vice versa.
Meanwhile, Deep Copy will allow you to create a second instance of the original object. This means that a change in either one will not reflect in the other.
When Would You Use A List Vs A Tuple Vs A Set In Python
The differences between these data types come down to what they are being used for. A list is a widely used data structure that is good for many uses. They are ordered, changeable, and allow duplicate values. If you are working with a data set that shares many of these same characteristics, but the data needs to remain unchangeable, then you should use a tuple instead. Tuples are also ordered and allow duplicate members but remain unchanged.
For the purposes of memory usage, a tuple is a data type that would likely be used for memory-heavy tasks. As it is more specialized than a list, it takes up less memory and allows for faster iterations. While lists can be used for these kinds of programs, they would likely slow the program down and would not be ideal for use on large data sets that require a significant amount of memory usage.
You May Like: How To Transcribe An Interview In Apa Format
What Are Negative Indexes And Why Are They Used
To access an element from ordered sequences, we simply use the index of the element, which is the position number of that particular element. The index usually starts from 0, i.e., the first element has index 0, the second has 1, and so on.
When we use the index to access elements from the end of a list, its called reverse indexing. In reverse indexing, the indexing of elements starts from the last element with the index number 1. The second last element has index 2, and so on. These indexes used in reverse indexing are called negative indexes.
Become A Python Developer
Speak to a Learning Advisor to learn more about how our bootcamps and courses can help you become a Python Developer.
Once you have been invited for a Python Developer job interview, youll need to brush up on your programming language knowledge and review your projects and achievements.
The interview format will vary depending on the company. Typically, you can expect an on-site and/or take-home coding challenge. In addition, you will likely also be asked Python interview questions that assess essential soft skills, such as communication and teamwork.
To ace the interview, you should know the ins and outs of Python, including frameworks, data structures, functions, and libraries. You will be asked a mix of questions that test your knowledge and understanding, as well as your technical abilities. For technical questions, it is usually more important to clearly explain your thought process than arrive at the correct solution.
When prepping, practice a range of interview questions and answers, including programming and behavioral questions. You should also go over your Python projects. You will be asked about your past work, so practice talking about your projects and successes.
Python Developer Interview Tips
To help you prepare for the interview, we have put together a list of the top Python interview questions and answers.
You May Like: How To Cite An Interview Mla
Need Help Finding Great Python Developers
Python is such an in-demand programming language that it can be extremely hard to find enough qualified developers when you need them. You also need developers who have the right soft skills to work with your current team. But how do you find the best software developers? This is where we come in!
At Full Scale, we recruit, assess, hire, and retain the best software developers. We hire only the top 3% of developers we interview. Take a look at our rigorous assessment process:
Our recruitment process ensures that our talents have the skills and experience to succeed as a part of your team.Are you looking for world-class Python developers? Get in touch now and lets start setting up your dream development team!
What Are Python Modules
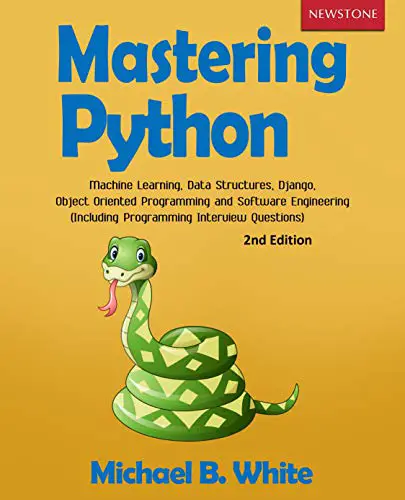
Files containing Python codes are referred to as Python Modules. This code can either be classes, functions, or variables and saves the programmer time by providing the predefined functionalities when needed. It is a file with .py extension containing an executable code.
Commonly used built modules are listed below:
Don’t Miss: How To Interview A New Employee
Explain How Can We Build Or Set Up The Database In Django
we can make use of the edit mysite/setting.py command, which is a simple Python module that consists of levels for presenting or displaying Django settings.
- Engines: By these engines you change the database by using commands such as django.db.backends.postgresql_psycopg2, django.db.backends.sqlite3, django.db.backends.oracle, django.db.backends.mysql, and so on.
- Name: This represents the name of your own database. If you are familiar with using SQLite as your database, in such cases database is available as a file on your particular system. Moreover, the name should be as a fully absolute or exact path along with the file name of the particular file.
- Suppose if we are not using SQLite as your database then additional settings such as password, user, the host must be added.
Django mainly uses SQLite as its default database to store entire information in a single file of the filesystem. If you want to use different database servers rather than SQLite, then make use of database administration tools to create a new database for the Django project. Another way is by using your own database in that place, and the remaining is to explain Django about how to use it. This is the place in which Pythons project settings.py file comes into the picture.
We need to add the below code to the setting.py file:
DATABASE = }
Things To Learn In Python
- Learn the basics of Python, its history, installations, syntax and other basic constructs such as operators, variables, and statements.
- Understand the applications of Python and the difference between Python 2 and Python 3.
- Learn the important concepts such as loops and decision making.
- Understand the basic data structure such as dictionaries, sets, and lists.
- Learn how to develop a virtual environment.
- Get started with functions as well as recursion.
- Understand object-based concepts such as classes, methods, overloading, and inheritance.
- Gain experience with modules like calendar, namedruple, and OS.
- Know how to do file handling and understand other complex concepts such as generators, decorators, and shallow and deep copying.
- Get started with building GUIs with Python.
- Know how to generate and use random numbers as well as regular expressions.
- Understand more complicated topics such as XML processing, networking, and multiprocessing.
- Know how to use Pandas, NumPy, and SciPy.
- Know how to debug log, unit test, serialize and access the database.
Also Check: What Questions To Ask After An Interview
In Python What Is A Lambda Function
A Python lambda function is a function without any name, or an anonymous function. A lambda function is an anonymous function due to it not having a function name until its assigned one. It takes any number of arguments and then returns a single expression. They are typically used in order to take up less space for an argument. The syntax for a lambda function consists of an argument and an expression separated by a single : in the middle. There can be any number of arguments, but only one expression. For a lambda function, a return statement is not necessary, as it is able to return an expression on its own.
Name The Fundamental Python Features
- Interpreted language. Unlike C, theres no need to compile Python code before running it.
- Object-oriented language. Like Java and other OOP technologies, Python supports inheritance and class definition. On the other hand, theres no support for access specifiers .
- Code execution is slower than that of compiled languages. To find their way around the issue, developers use packages like Numpy.
Recommended Reading: Manual Testing Interview Questions For Experienced
What Is A Dynamically Typed Language
Before we understand a dynamically typed language, we should learn about what typing is. Typing refers to type-checking in programming languages. In a strongly-typed language, such as Python, “1” + 2 will result in a type error since these languages don’t allow for “type-coercion” . On the other hand, a weakly-typed language, such as Javascript, will simply output “12” as result.
Type-checking can be done at two stages –
- Static – Data Types are checked before execution.
- Dynamic – Data Types are checked during execution.
Python is an interpreted language, executes each statement line by line and thus type-checking is done on the fly, during execution. Hence, Python is a Dynamically Typed Language.
What Are Functions In Python
Ans: Functions in Python refer to blocks that have organized, and reusable codes to perform single, and related events. Functions are important to create better modularity for applications that reuse a high degree of coding. Python has a number of built-in functions like print. However, it also allows you to create user-defined functions.
Recommended Reading: How To Prepare For Code Review Interview
Coding Interview Questions On Arrays
Here are some sample coding interview questions on Arrays:
What Is The Lambda Function In Python
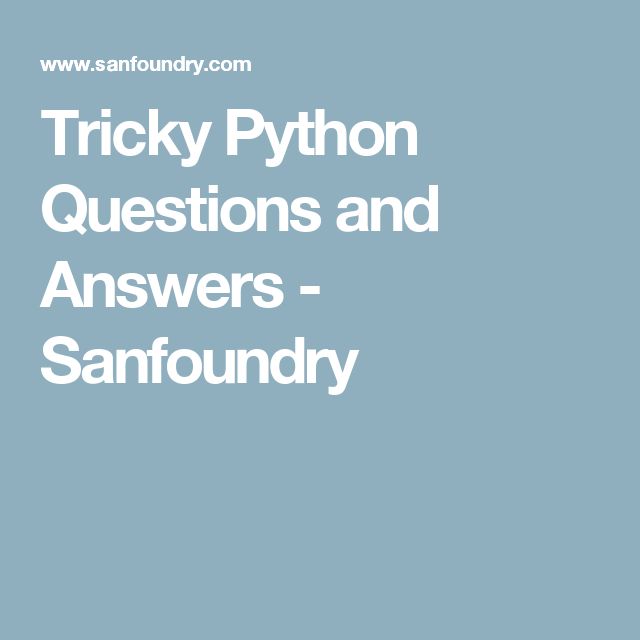
A lambda function is an anonymous function in Python. To define anonymous functions, we use the lambda keyword instead of the def keyword, hence the name lambda function. Lambda functions can have any number of arguments but only one statement.
For example:
l = lambda x,y : x*yprint)
Output:30
Any more queries? Feel free to share all your doubts with us in our Python Community and get them clarified today!
You May Like: How To Prepare For A Customer Service Interview
How Multithreading Is Achieved In Python
- Although Python includes a multi-threading module, it is usually not a good idea to utilize it if you want to multi-thread to speed up your code.
- As this happens so quickly, it may appear to the human eye that your threads are running in parallel, but they are actually sharing the same CPU core.
- The Global Interpreter Lock is a Python concept . Only one of your ‘threads’ can execute at a moment, thanks to the GIL. A thread obtains the GIL, performs some work, and then passes the GIL to the following thread.