Check Whether A List Is Empty Or Not In C#
The Count property can be used to check if a list is empty or not. This property gets the number of elements in the list. So, if the Count property gives 0 for a list, this means that the list is empty.
A program that checks if the list is empty or not using the Count property in C# is given as follows:
using System using System.Collections.Generic class Demo else } if else } }}
The output of the above program is as follows:
The elements in List 1 are:71593List 2 is empty
In the above program, if the list is empty then that is printed. Otherwise all the list elements are printed.
Oop C# Interview Questions
Q8). How is operator Overloading achieved in C#? Explain for example?
In C# most of the built-in operators are available and can be overloaded or redefined using the operator keyword. The example below explains the syntax used to implement the addition operator for a user-defined class. public static Rectangle operator+
Q9). Differentiate between object type and dynamic type variables.
Dynamic type variables handle type checking at the runtime. Object type variables handle type checking during compile time.
Q10). What is encapsulation? How is encapsulation handled in C#?
Encapsulation is a classic object-oriented design principle. Encapsulation reduces coupling between objects and increases maintainable code. It involves enclosing objects within a logical pack by limiting access to implementation details. In C# encapsulation is achieved through the access specifiers public, private, protected, internal, and protected internal.
Q11). Predict the output of the code:
delegate void Iterator static void Main ) } Foreach } the number 15 is printed fifteen times.
Explanation The program tests the developer on their knowledge working with loops and delegates. As the delegate is added within the for loop, and because the delegate is the only reference for the variable instead of the value itself, the loop sets the value of the variable I to 15 before it is called within each delegate.
Q12). What are generics in C#?
Q14). Differentiate between Array and ArrayList in C#?
Whats Difference Between Delegate And Events
An event is just a wrapper for a multicast delegate. Adding a public event to a class is almost the same as adding a public multicast delegate field. In both cases, subscriber objects can register for notifications, and in both cases the publisher object can send notifications to the subscribers. However, a public multicast delegate has the undesirable property that external objects can invoke the delegate, something wed normally want to restrict to the publisher. Hence events an event adds public methods to the containing class to add and remove receivers, but does not make the invocation mechanism public.
You May Like: Should I Do An Exit Interview
What Is Nested Types In C# And How To Use It
Types are usually declared directly inside a namespace. You can, however, also declare types inside a class or struct declaration. Types declared inside another type declaration are called nested types. Like all type declarations, nested types are templates for an instance of the type. A nested type is declared like a member of the enclosing type. A nested type can be any type. An enclosing type can be either a class or a struct.
For example, the following code shows class MyClass, with a nested class called MyCounter.
class MyClass // Enclosing class ... }
Master C Programming With Simplilearn
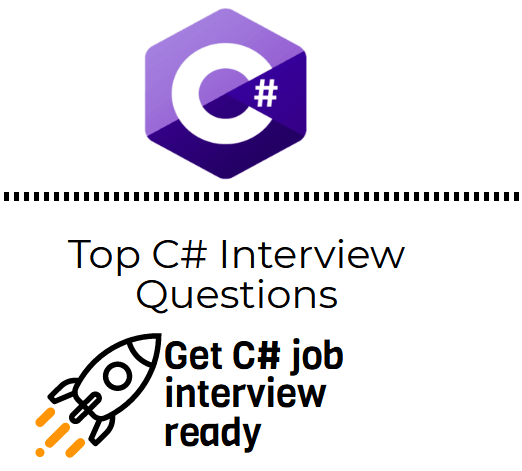
C# is the primary programming language which helps in every higher level programming language. C# developers are in high demand and can find lucrative job opportunities in fields like data science, front end development, backend development, or app development. And since Microsoft backs it, the popularity of C# will continue to increase.
Learning C# can really help accelerate your career. You can enroll in the Full Stack Web Developer Mean Stack course by Simplilearn, which provides full understanding of software development and testing technologies such as JavaScript, Node.js, Angular, Docker, and Protractor You’ll create an end-to-end application, test and deploy code, and use MongoDB to store data.
Stack developers who have completed the Full Stack Web Developer Mean Stack course find good job prospects and salary hikes over a period of time.
Check out Simplilearns Cloud Architect Certification Course, which will build your AWS, Microsoft Azure and Google Cloud Platform expertise from the ground up. Youll learn to master the architectural principles and services of the top cloud platforms, design and deploy highly scalable, fault-tolerant applications and develop skills to transform yourself into an AWS, Azure cloud and GCP architect.
After completion of this cloud architect certification, you become eligible for some of the rewarding career opportunities as a Solutions Architect, Network Architect, Software Architect, and Cloud Engineer. Enroll today!
You May Like: What Questions To Ask Someone You Are Interviewing
List Down The Commonly Used Types Of Exceptions In Net
ArgumentException, ArgumentNullException, ArgumentOutOfRangeException, ArithmeticException, DivideByZeroException ,OverflowException, IndexOutOfRangeException, InvalidCastException, InvalidOperationException, IOEndOfStreamException , NullReferenceException, OutOfMemoryException, StackOverflowException etc.
What Does A Partial Class Mean
A partial class divides the definition of a class into different classes that can be found in the same or different source code files. When a class is created, all of the methods from all of the source files can be made accessible using the same object. A class meaning can be written in multiple files, but it is gathered as a single class at runtime. This is what the word “partial” means.
You May Like: Interview Questions For Infrastructure Project Manager
Role Of As Operator In C#
The as operator in C# is somewhat similar to the is operator. However, the is operator returns boolean values but the as operator returns the object types if they are compatible to the given type, otherwise it returns null.
In other words, it can be said that conversions between compatible types are performed using the as operator.
A program that demonstrates the as operator in C# is given as follows:
namespace IsAndAsOperators class B class Program ", func) System.Console.WriteLine) } public static string func }}
The output of the above program is given as follows:
The object obj1 is of class A? : This is an object of class A with value 9The object obj2 is of class A? : This is not an object of class A
If you are not sure of the parameters in the arrays, then you can use the param type arrays. The param arrays hold n number of parameters.
The following is an example:
using System namespace Application return mul } } class Demo ", mul) Console.ReadKey } }}
The output:
Result = 60
Here is another example of param arrays in C#:
using System namespace ArrayDemo return sum } static void Main ", sum) sum = SumOfElements Console.WriteLine sum = SumOfElements Console.WriteLine } }}
The output of the above program is as follows:
Sum of 5 Parameters: 23Sum of 2 Parameters: 12Sum of 3 Parameters: 26
How To Update Webconfig Programatically
This file is an XML file, and reading/writing XML is supported by .NET. You can choose one of the method as per your requirement:
- Use System.Xml.XmlDocument class. It implements DOM interface this way is the easiest and good enough if the size of the document is not too big.
- Use the classes System.Xml.XmlTextWriter and System.Xml.XmlTextReader this is the fastest way of reading
- Use the class System.Xml.Linq.XDocument this is to support LINQ to XML Programming.
Read Also: What Is Pi Test In Interview
Explain The Aspnet Page Life Cycle In Brief
The ASP.NET life cycle is divided into Application Life Cycle and Page Life Cycle. The page life cycle phases include initialization, instantiation of the controls on the page, restoration and maintenance of the state, execution of the event handler codes, and page rendering. ASP.NET Application life cycle consists of the following stages: application start, object creation, HttpApplication creation, dispose of, application end.
location is null
1/1/0001 12:00:00 AM
Although both variables are uninitialized, String is a reference type and DateTime is a value type. As a value type, an uninitialized DateTime variable is set to a default value of midnight of 1/1/1 , not null.
What Is C# Garbage Collection
The process of cleaning up memory that has been occupied by unwanted objects is known as garbage collection. A certain amount of memory is automatically assigned to a class object when it is created in the heap memory. Now that you’ve finished working on the object, the storage device it once occupied is wasted. Memory needs to be freed up. In three situations, garbage collection takes place:
- If the objects’ combined memory usage goes over a certain threshold.
- Upon calling the trash collection method
- If your computer has insufficient physical memory
Also Check: How To Prepare For A Bank Teller Interview
What Is Static Constructor
- Static constructor is used to initialize static data members as soon as the class is referenced first time.
Related Tags: kurs c# , c# programmieren , tutorial c# visual studio , learn programming with c# , c# kurs online , the best way to learn c# , c# tutorial for complete beginners from scratch , tuto c# , manual c#
What Are Some Of The Features Of Generics In C#
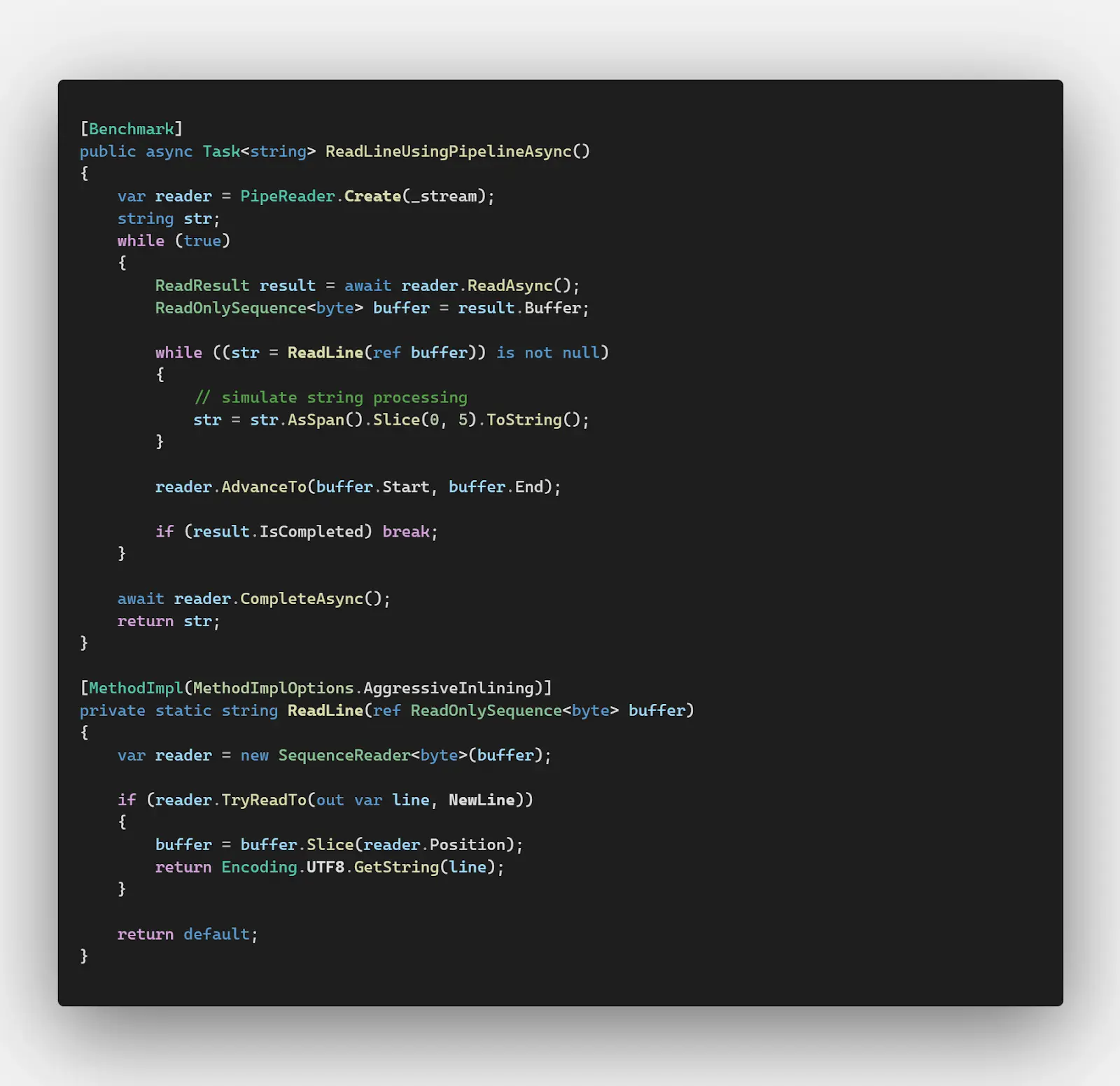
Generics allow a developer to define a class or method that can work with virtually any data type by delaying specification of the programming elements’ data types until they are needed.Generics come with a number of features.
- They make C# code reusable, type safe, and performance optimized.
- They allow the developer to create generic classes, methods, events, delegates, and interfaces.
- They allow the developer to create generic collection classes in the System.Collections.Generic namespace.
- They make it possible to get real-time information on the types used in a generic data type at runtime via reflection.
- Microsoft Access
- Microsoft Power Automate
- Web Development
- User Authentication
Senior Unity DeveloperSkills: – Fluent in C# and Unity development- Clean, efficient, well-documented code- Task tracking systems experience such as JIRA, Pivotal Tracker, Trello, Asana, or TeamForge- Familiar with the Agile development method of doing sprints- Experienced with Source Control Software- 3D Math and applied linear algebra- Unit Testing with NUnit experience- Strong knowledge of OOP and Design Patterns- Debugging and profilingAbilities:- Fluent written English and well spoken English.- Detail oriented, quality driven, straightforward, self-motivated, and proactive- Able to work independently, creatively, and track multiple deadlines to success- Experienced with software development methodologies as well as build and release procedures
- API Development
- ASP.NET Web API
Read Also: How To Get A News Interview
C# Coding Interview Questions
49. Print the output of the below program? Error Line will throw any error in below code?
class A } class B:A } class Test }
Output: ‘Error Line’ will throw error – “Cannot implicitly convert type ‘A’ to ‘B’. An explicit conversion exists “. Output will be as below.A – display methodB – display method
55. What will be the output here? or will it throw any error?
public void display catch catch finally }
56. Print the output of this program?
int a = 0 try catch } catch
- Output will be – “inner ex” as we are not throwing anything from the inner catch block, so the outer catch block will not execute.
- If we uncomment ‘Line 1’ then output will be – “inner ex” and “outer ex” and outer catch will throw an exception.
- If we uncomment ‘Line 2’ then output will be the same – “inner ex” and “outer ex” and outer catch will throw an exception.
- If we uncomment ‘Line 3’ then output will be – “inner ex” and “outer ex” but the exception message in outer catch will be different, overridden by athrow message from inner catch block.
In C# What Is An Exception
- In coding and programming, much like any other discipline, people tend to make mistakes. Programming is not particularly known to be forgiving, that is why the slightest mistake in the code can result in unexpected conclusions. When programmers make an error in their code, the C# operation spontaneously generates an error message. The generation of an error message is called throwing an exception in C#. What one must note is that exceptions in C# can also be manipulated and used for various purposes by the coder or programmer. ArithmeticException, FileNotFoundException, IndexOutOfRangeException, TimeOutException, etc are just some of the exception classes available for programmers to use in C#
Don’t Miss: How To Ace Behavioral Interview
How To Use Nullable Types In Net
Nullable types allow you to create a value type variable that can be marked as valid or invalid so that you can make sure a variable is valid before using it. Regular value types are called non-nullable types.
The important things you need to know about nullable type conversions are the following:
There is an implicit conversion between a non-nullable type and its nullable version. That is, no cast is needed. There is an explicit conversion between a nullable type and its non-nullable version.
For example, the following lines show conversion in both directions. In the first line, a literal of type int is implicitly converted to a value of type int? and is used to initialize the variable of the nullable type. In the second line, the variable is explicitly converted to its non-nullable version.
int? myInt1 = 15 // Implicitly convert int to int?int regInt = myInt1 // Explicitly convert int? to int
Q50 What Is Thread Pooling
Ans- A Thread pool is a collection of threads that perform tasks without disturbing the primary thread. Once the task is completed by a thread it returns to the primary thread.
This brings us to the end of this article on C# Interview Questions. I hope it helped in adding up to your knowledge. Wishing you all the best for your interview. Happy learning.
In case you are looking for the most common Technical Interview Questions, read along:
You May Like: Interview With An Artist Questions And Answers
Why Do We Use The Dictionary Class Instead Of The Hashtable Class
Dictionary is a generic type, Hashtable is not. That means you get type safety with Dictionary, because you cant insert any random object into it, and you dont have to cast the values you take out.
Hashtable supports multiple reader threads with a single writer thread, while Dictionary offers no thread safety. If you need thread safety with a generic dictionary, you must implement your own synchronization.
How To Split String Using Regex In C#
The Regular Expressions Split methods are similar to the String.Split method, except that Regex.Split method splits the string at a delimiter determined by a Regular Expression instead of a set of characters.
When using Regular Expressions you should use the following namespace:
using System.Text.RegularExpressions string str = "test1\n \ntest2\n \ntest3\n \n \ntest4" string result = Regex.Split for MessageBox.Show //Output:test1test2test3test4
Also Check: Interview Questions For An Hr Manager
What Is The Difference Between Static Class And Singleton Instance
In c# a static class cannot implement an interface. When a single instance class needs to implement an interface for some business reason or IoC purposes, you can use the Singleton pattern without a static class. You can clone the object of Singleton but, you can not clone the static class object Singleton object stores in Heap but, static object stores in stack A singleton can be initialized lazily or asynchronously while a static class is generally initialized when it is first loaded
What Are Class Instances In C#
![Top 15 C# Interview Questions and Answers 2020 [Updated] Top 15 C# Interview Questions and Answers 2020 [Updated]](https://www.interviewprotips.com/wp-content/uploads/top-15-c-interview-questions-and-answers-2020-updated.png)
An object of a class is a class instance in C#. All the members of the class can be accessed using the object or instance of the class.
The definition of the class instance starts with the class name that is followed by the name of the instance. Then the new operator is used to create the new instance.
A program that demonstrates a class instance in C# is given as follows:
using System namespace Demo public int returnSum } class Test " , obj.returnSum) } }}
The output of the above program is given as follows:
The sum of 8 and 5 is: 13
Also Check: Mock Interview For Software Engineer
What Is A Delegate
A delegate is a reference type entity that is used to store the reference of a method. So whenever you need to call a method, you can easily use the delegate by initializing a pointer to that method, thereby helping to implement Encapsulation.
// C# program to show the use of Delegatesusing System namespaceDelegateExample + ) = ", a, b, a + b) }// Method differenceOf2Nums having same return type// and parameter type as that of delegatepublicvoiddifferenceOf2Nums - ) = ", a, b, a - b) }// Main MethodpublicstaticvoidMain }}
Delegates in C# are similar to function pointers in C/C++ and can be used to pass methods as arguments to other methods, chained together, or even can be used to call methods on events.
Q7 What Do You Understand About Polymorphism In C#
This is one of the most commonly asked C# interview questions and answers for experienced professionals. Polymorphism is one of the three main pillars of object-oriented programming after encapsulation and inheritance.
The term polymorphism means “many shapes.” It occurs when there are many classes related to each other by inheritance.
For instance, at runtime, derived class objects may be treated as base class objects in places like collections/ method parameters/ arrays. In such polymorphism, declared and runtime types of objects are different. Thus, polymorphism allows a class to have multiple implementations with the same name.
Also Check: How To Crack Amazon Data Scientist Interview
What Are Jagged Arrays
- Jagged arrays are one of the most important components of C#. A jagged array typically contains elements that are also of an array type. These elements can vary in size and dimensions. Simply put, an array of arrays is called a jagged array. Within your program it will look like the following:
int JaggedArray = new in JaggedArray=new int JaggedArray=new int JaggedArray=new int JaggedArray=new int