Q 95 In Java Static As Well As Private Method Overriding Is Possible Comment On The Statement
In Java, you could indeed override a private or static method. If you create a similar method in a child class with the same return type and method arguments, it will hide the super class method this is known as method hiding. Similarly, you cannot override a private method in a subclass because it is not accessible from that.
What Is Garbage Collection
Java automatically manages heap memory. When an object is unreferenced, java can reclaim the allocated heap memory. This automatic memory reclamation process is called garbage collection.
Garbage collection is a two-step process, where the collector first has to analyse heap memory contents, identify the objects which are unreferenced and marking these memory areas. In a second stage, the collector will delete the unreferenced memory and may also compact the remaining referenced memory to simplify and speed up the process of future memory allocations.
An empirical analysis of applications shows that most objects are short-lived. To improve Garbage collection performance, Java separates heap memory into three main generations, namely young, tenured and permanent generation. Objects are created in the young generation and eventually promoted to the tenured generation after the objects reach a certain threshold age.
Can We Change The Scope Of The Overridden Method In The Subclass
Yes, we can change the scope of the overridden method in the subclass. However, we must notice that we cannot decrease the accessibility of the method. The following point must be taken care of while changing the accessibility of the method.
- The private can be changed to protected, public, or default.
- The protected can be changed to public or default.
- The default can be changed to public.
- The public will always remain public.
You May Like: What Motivates You Interview Question
Why Does The Java Array Index Start With 0
It is because the 0 index array avoids the extra arithmetic operation to calculate the memory address.
Example – Consider the array and assume each element takes 4-byte memory space. Then the address will be like this –
Now if we want to access index 4. Then internally java calculates the address using the formula-
. So according to this. The starting address of the index 4 will be – = 116. And exactly that’s what the address is calculated. Now consider the same with 1 index Array –
Now if we apply the same formula here. Then we get – 116 as the starting address of the 4th index. Which is wrong. Then we need to apply formula – .
And for calculating this, an extra arithmetic operation has to be performed. And consider the case where millions of addresses need to be calculated, this causes complexity. So to avoid this, ) the index array is supported by java.
Q4 What Is Data Encapsulation And Whats Its Significance
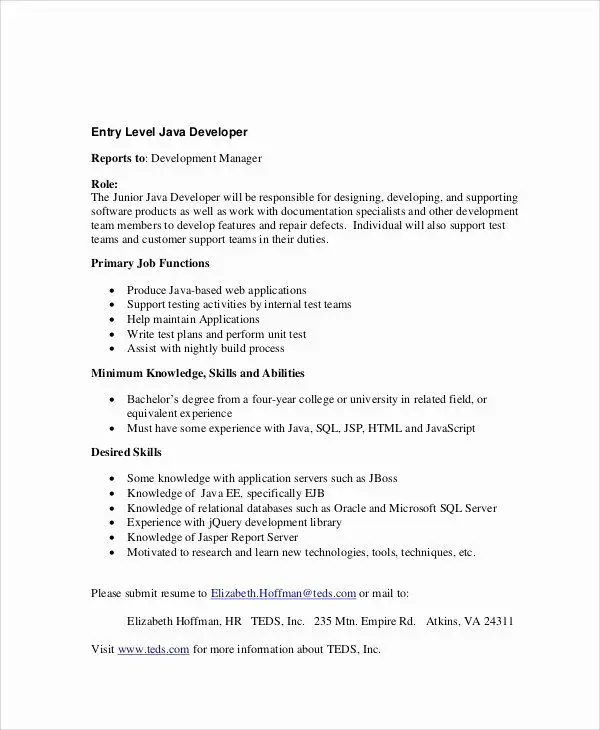
Ans: Encapsulation is a concept in Object Oriented Programming for combining properties and methods in a single unit.
Encapsulation helps programmers to follow a modular approach for software development as each object has its own set of methods and variables and serves its functions independent of other objects. Encapsulation also serves data hiding purpose.
Recommended Reading: How To Be Confident In An Interview
Why Is It Said That The Length Method Of String Class Doesn’t Return Accurate Results
- The length method returns the number of Unicode units of the String. Let’s understand what Unicode units are and what is the confusion below.
- We know that Java uses UTF-16 for String representation. With this Unicode, we need to understand the below two Unicode related terms:
- Code Point: This represents an integer denoting a character in the code space.
- Code Unit: This is a bit sequence used for encoding the code points. In order to do this, one or more units might be required for representing a code point.
Now if a string contained supplementary characters, the length function would count that as 2 units and the result of the length function would not be as per what is expected.
In other words, if there is 1 supplementary character of 2 units, the length of that SINGLE character is considered to be TWO – Notice the inaccuracy here? As per the java documentation, it is expected, but as per the real logic, it is inaccurate.
Can You Tell The Difference Between Equals Method And Equality Operator In Java
equals | |
---|---|
This is a method defined in the Object class. | It is a binary operator in Java. |
This method is used for checking the equality of contents between two objects as per the specified business logic. | This operator is used for comparing addresses , i.e checks if both the objects are pointing to the same memory location. |
Note:
- In the cases where the equals method is not overridden in a class, then the class uses the default implementation of the equals method that is closest to the parent class.
- Object class is considered as the parent class of all the java classes. The implementation of the equals method in the Object class uses the == operator to compare two objects. This default implementation can be overridden as per the business logic.
Recommended Reading: What Are The Most Common Interview Questions For Teachers
How Is Java Different From C++
- C++ is only a compiled language, whereas Java is compiled as well as an interpreted language.
- Java programs are machine-independent whereas a c++ program can run only in the machine in which it is compiled.
- C++ allows users to use pointers in the program. Whereas java doesnt allow it. Java internally uses pointers.
- C++ supports the concept of Multiple inheritances whereas Java doesn’t support this. And it is due to avoiding the complexity of name ambiguity that causes the diamond problem.
How To Remove Leading And Trailing Whitespaces From A String
Java String class contains two methods to remove leading and trailing whitespaces – trim, and strip. The strip method was added to the String class in Java 11. However, the strip method uses Character.isWhitespace method to check if the character is a whitespace. This method uses Unicode code points whereas the trim method identifies any character having codepoint value less than or equal to âU+0020â as a whitespace character. The strip method is the recommended way to remove whitespaces because it uses the Unicode standard.
String s = " abc def\t" s = s.strip System.out.println
Since String is immutable, we have to assign the strip output to the string.
You May Like: How To Interview A Therapist
What Is The Difference Between An Object
There are the following basic differences between the object-oriented language and object-based language.
- Object-oriented languages follow all the concepts of OOPs whereas, the object-based language doesn’t follow all the concepts of OOPs like inheritance and polymorphism.
- Object-oriented languages do not have the inbuilt objects whereas Object-based languages have the inbuilt objects, for example, JavaScript has window object.
- Examples of object-oriented programming are Java, C#, Smalltalk, etc. whereas the examples of object-based languages are JavaScript, VBScript, etc.
What Do You Consider When Choosing Between Hashset And Treeset When You Need A Set In Java
The interviewer may ask questions that test your decision-making skills and ability to think critically when working development projects. Show the interviewer your ability to make decisions that lead to successful project outcomes by giving examples of how you completed this type of task in your past roles.
Example:”**At first, it appears that a HashSet would be superior to a TreeSet, however, when I need to keep order over inserted elements or a query for inserted elements within a set, TreeSet is my go-to execution. For instance, in my last job, I would initiate the storage of time stamped objects. TreeSet made it easier for myself and my boss to look up multiple objects within the same time stamp, something which HashSet would require extra programming for.”
Don’t Miss: How To Answer Properly In A Job Interview
How To Create A Record In Java
Records is a preview feature introduced in Java 14. Records allow us to create a POJO class with minimal code. It automatically generates hashCode, equals, getter methods, and toString method code for the class. Records are final and implicitly extends java.lang.Record class.
import java.util.Map public record EmpRecord
Read more at Records in Java.
Comment On Method Overloading And Overriding By Citing Relevant Examples
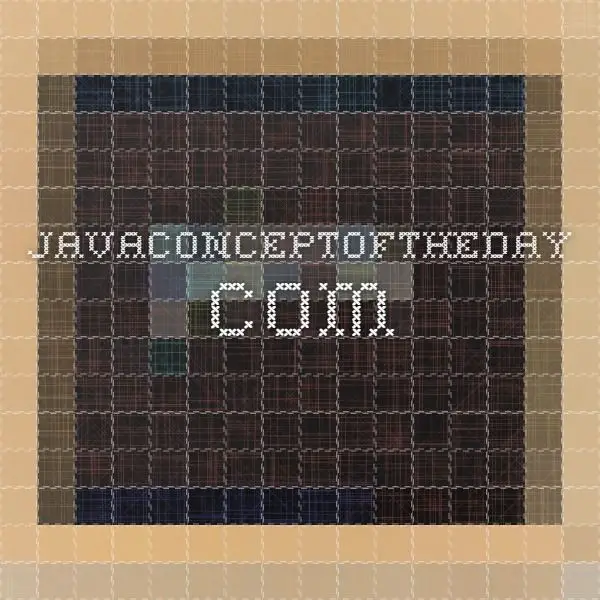
In Java, method overloading is made possible by introducing different methods in the same class consisting of the same name. Still, all the functions differ in the number or type of parameters. It takes place inside a class and enhances program readability.
The only difference in the return type of the method does not promote method overloading. The following example will furnish you with a clear picture of it.
classOverloadingHelppublicintfindarea}
Both the functions have the same name but differ in the number of arguments. The first method calculates the area of the rectangle, whereas the second method calculates the area of a cuboid.
Method overriding is the concept in which two methods having the same method signature are present in two different classes in which an inheritance relationship is present. A particular method implementation is possible for the derived class by using method overriding.Lets give a look at this example:
classHumanBeing}classAthleteextendsHumanBeing}
Both class methods have the name walk and the same parameters, distance, and time. If the derived class method is called, then the base class method walk gets overridden by that of the derived class.
You May Like: What Questions To Ask In An Executive Assistant Interview
Common Java Coding Interview Questions
Tegan Griffiths is a technical writer with a passion for everything from artificial learning to machine learning. Tegan currently writes content for an association magazine in the tech business.
Related: Top Interview Tips: Common Questions, Body Language & More
In this video, we dissect an entire job interview from start to finish. We analyze everything from common interview questions to etiquette and how to follow up.
If you’re preparing to interview for a job that requires knowledge of Java, it’s important to learn about some of the common questions the hiring manager may ask. Practicing your responses can help you feel more confident when responding to questions or writing test code. Though the questions the interviewer asks vary, you can practice your responses to help refresh your knowledge of Java.
In this article, we review why Java is a popular language among employers and provide examples of Java coding interview questions and answers to help you prepare.
Please note that none of the organizations mentioned in this article are affiliated with Indeed.
Although Inheritance Is A Popular Oops Concept It Is Less Advantageous Than Composition Explain
Inheritance lags behind composition in the following scenarios:
- Multiple-inheritance is not possible in Java. Classes can only extend from one superclass. In cases where multiple functionalities are required, for example – to read and write information into the file, the pattern of composition is preferred. The writer, as well as reader functionalities, can be made use of by considering them as the private members.
- Composition assists in attaining high flexibility and prevents breaking of encapsulation.
- Unit testing is possible with composition and not inheritance. When a developer wants to test a class composing a different class, then Mock Object can be created for signifying the composed class to facilitate testing. This technique is not possible with the help of inheritance as the derived class cannot be tested without the help of the superclass in inheritance.
- The loosely coupled nature of composition is preferable over the tightly coupled nature of inheritance.
Lets take an example:
package comparison publicclassTop}classBottomextendsTop}
In the above example, inheritance is followed. Now, some modifications are done to the Top class like this:
publicclassToppublicvoidstop}
classBottom}
Read Also: How To Interview Someone Online
What Is The Output Of The Below Code And Why
publicclassInterviewBit}
bit would have been the result printed if the letters were used in double-quotes . But the question has the character literals being used which is why concatenation wouldn’t occur. The corresponding ASCII values of each character would be added and the result of that sum would be printed.The ASCII values of b, i, t are:
98 + 105 + 116 = 319
Hence 319 would be printed.
Can We Declare The Static Variables And Methods In An Abstract Class
Yes, we can declare static variables and methods in an abstract method. As we know that there is no requirement to make the object to access the static context, therefore, we can access the static context declared inside the abstract class by using the name of the abstract class. Consider the following example.
Output
hi !! I am good !!i = 102
Recommended Reading: How To Call And Set Up An Interview
How Is Jsp Better Than Servlet Technology
JSP is a technology on the servers side to make content generation simple. They are document-centric, whereas servlets are programs. A Java server page can contain fragments of Java program, which execute and instantiate Java classes. However, they occur inside an HTML template file. It provides the framework for the development of a Web Application.
What Are The Benefits Of Java Certification For Freshers And Experienced Developers
Freshers:
- Good programming language for beginners
- No need to switch languages, as your career progresses you can do more complex tasks with Java
- Since, its widely adopted, chances of finding a Java developer job is fairly easy
- Because Java can be used in many applications, it is very sustainable to learn.
Experienced:
- More opportunities
- Broad range of salary options based on skill set, In India salary ranges from
- In the US, average Salary of Professionals with Java skills is $110k
- It is one of most in-demand skill sets in the IT sector
Don’t Miss: What To Ask In An Interview As An Employer
+ Java Interview Questions For 2 To 3 Years Experienced Programmers
So, without wasting any more of your time, here is my list of some of the frequently asked Core Java Interview Questions for beginner programmers. This list focuses on beginners and less experienced devs, like someone with 2 to 3 years of experience in Java.
1) How does Java achieve platform independence? hint: bytecode and Java Virtual Machine
2) What is ClassLoader in Java? hint: part of JVM that loads bytecodes for classes. You can write your own.
3) Write a Java program to check if a number is Even or Odd? hint: you can use bitwise operator, like bitwise AND, remember, even the number has zero at the end in binary format and an odd number has 1 in the end.
4) Difference between ArrayList and HashSet in Java? hint: all differences between List and Set are applicable here, e.g. ordering, duplicates, random search, etc. See Java Fundamentals: Collections by Richard Warburton to learn more about ArrayList, HashSet and other important Collections in Java.
5) What is double-checked locking in Singleton? hint: two-time check whether instances are initialized or not, first without locking and second with locking.
6) How do you create thread-safe Singleton in Java? hint: many ways, like using Enum or by using double-checked locking pattern or using a nested static class.
7) When to use the volatile variable in Java? hint: when you need to instruct the JVM that a variable can be modified by multiple threads and give hint to JVM that does not cache its value.
Why Hire A Java Developer

For many, 2020 is known as the quarantine year. Most companies who had to lock their doors started strengthening their online presence. Some of them use LinkedIn strategies or any type of social media to connect with their customers. Others opt for a more significant digital presence by building business applications and websites. The results? More loyal customers and engaged users that turn into potential customers.
If you hire a Java developer, youll have someone on your team capable of designing, developing, and managing Java-based applications, depending of course if youll be hiring an entry-level java developer or a senior one. Different applications such as:
Don’t Miss: What Are The Most Common Interview Questions
Q42 Why Java Strings Are Immutable In Nature
In Java, string objects are immutable in nature which simply means once the String object is created its state cannot be modified. Whenever you try to update the value of that object instead of updating the values of that particular object, Java creates a new string object. Java String objects are immutable as String objects are generally cached in the String pool. Since String literals are usually shared between multiple clients, action from one client might affect the rest. It enhances security, caching, synchronization, and performance of the application.
Why Is Java Coding Popular Among Employers
One of the biggest reasons Java is popular among employers is because of its independence. As long as your computer has the Java Runtime Environment, you can execute a Java program on your computer. Most computers can handle a JRE, including Macintosh, Linux, Unix, Windows and selective mobile phones.
Features that make Java programming popular include:
-
It’s easy to learn and implement.
-
Any code written with Java can run on virtually any computing platform.
-
The code is robust enough to independently run Java programs without any external dependencies.
-
Java has experienced consistent development.
-
It’s a memory management and multithreaded programming language.
Related:12 Tough Interview Questions and Answers
Don’t Miss: What Is An Entrance Interview For Student Loans