Python Coding Challenges On Array
You must practice these Python coding challenges on Array as they are frequently asked in technical interviews. The interviewer will ask you to write a program in Python to –
How To Create A Python Namespace
To interactively test my python script, I would like to create a Namespace object, similar to what would be returned by argparse.parse_args. The obvious way,
> > > import argparse> > > parser = argparse.ArgumentParser> > > parser.parse_argsNamespace> > > parser.parse_argsusage: : error: unrecognized arguments: - a
Process Python exited abnormally with code 2 may result in Python repl exiting on a silly error.
So, what is the easiest way to create a Python namespace with a given set of attributes?
E.g., I can create a dict on the fly ) but I cannot use it as a Namespace:
AttributeError: dict object has no attribute a
Answer#
#You can create a simple class:class Namespace: def __init__: self.__dict__.update#and it'll work the exact same way as the argparse Namespace class when it comes to attributes:> > > args = Namespace> > > args.a1> > > args.b'c'#Alternatively, just import the class it is available from the argparse module:from argparse import Namespaceargs = NamespaceAs of Python 3.3, there is also types.SimpleNamespace, which essentially does the same thing:> > > from types import SimpleNamespace> > > args = SimpleNamespace> > > args.a1> > > args.b'c'
The two types are distinct SimpleNamespace is primarily used for the sys.implementation attribute and the return value of time.get_clock_info.
Further comparisons:
Is It Possible To Call Static Method From Within Class Without Qualifying The Name In Python
class MyClass: @staticmethod def foo: print "hi" @staticmethod def bar: MyClass.foo
Is there a way to make this work without naming MyClass in the call? i.e. so I can just say foo on the last line?
Answer#
There is no way to use foo and get what you want. There is no implicit class scope, so foo is either a local or a global, neither of which you want.
You might find classmethods more useful:
class MyClass: @classmethod def foo: print "hi" @classmethod def bar: cls.foo#This way, at least you don't have to repeat the name of the class.
You May Like: Interview Technical Questions For Freshers
Java Interview Coding Challenges
Some of the popular Java interview coding challenges are based on these –
- Write a Java program to count the number of words in a string using HashMap.
- Implement explicit wait condition check using Java.
- Write a Selenium code to switch to the previous tab.
- Write a Java program to find duplicate characters in a string.
Python Framework Interview Questions
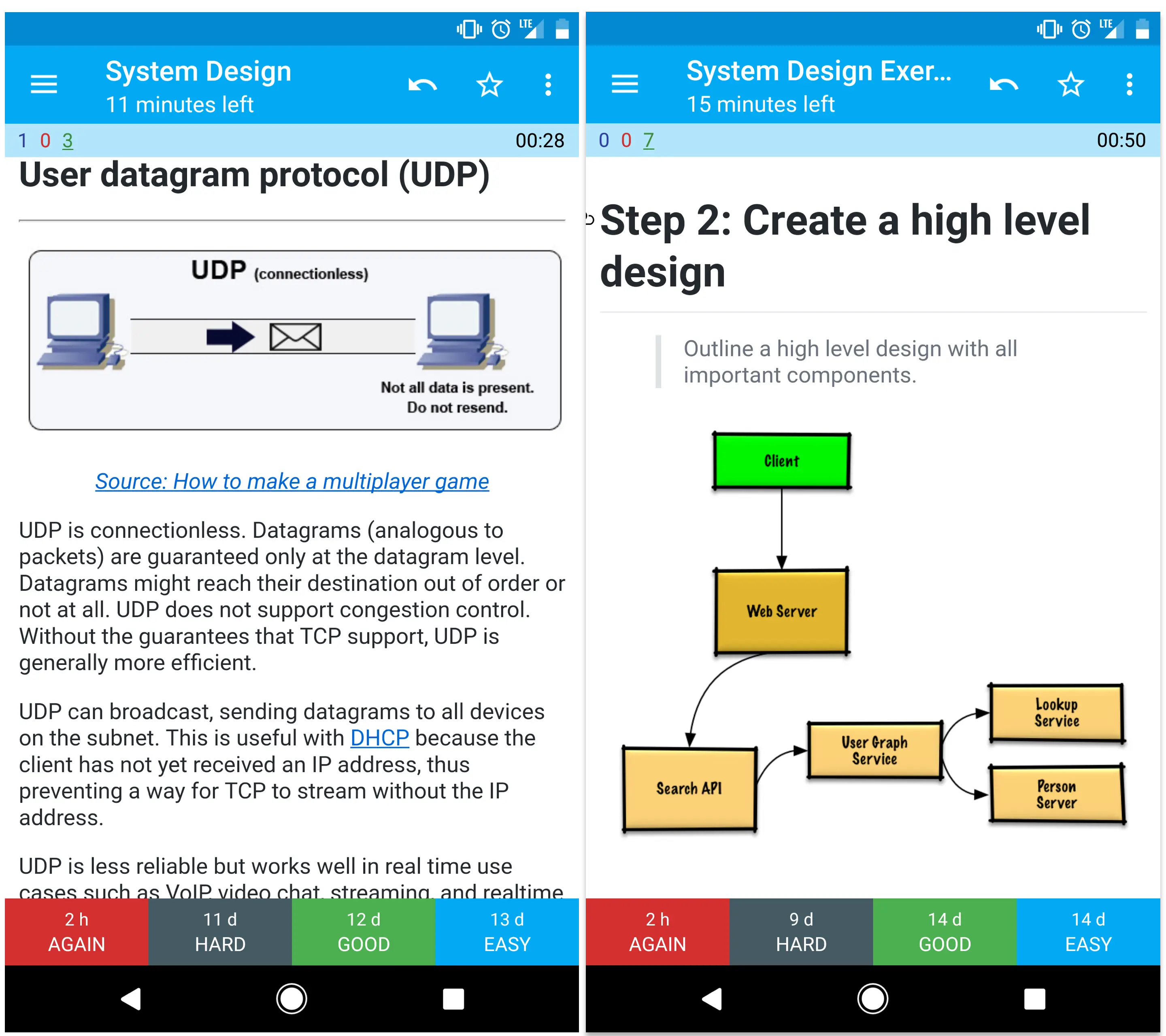
Q.53. Differentiate between Django, Pyramid, and Flask.
These are three major frameworks in Python. Here are the differences:
- We can also use Django for larger applications. It includes an ORM.
- Flask is a microframework for a small platform with simpler requirements. It is ready to use and you must use external libraries.
- The pyramid is for larger applications. It is flexible and you can choose the database, the URL structure, the templating style, and much more. It is also heavily configurable.
Q.54. Explain the Inheritance Styles in Django.
Talking on inheritance styles, we have three possible-
- Abstract Base Classes- For the parent class to hold information so we dont have to type it out for each child model
- Multi-table Inheritance- For subclassing an existing model and letting each model have its own database
- Proxy Models- For modifying the models Python-level behavior without having to change its fields
Q.55. What is Flask- WTF? Explain its features.
Flask-WTF supports simple integration with WTForms. It has the following features-
- Integration with wtforms
- Secure form with csrf token
- File upload compatible with Flask uploads
Q.56. What is Flask?
Python Flask, as weve previously discussed, is a web microframework for Python. It is based on the Werkzeug, Jinja 2 and good intentions BSD license. Two of its dependencies are Werkzeug and Jinja2. This means it has around no dependencies on external libraries. Due to this, we can call it a light framework.
Also Check: How To Dress For A Video Interview
Why Do Some Functions Have Underscores : : Before And After The Function Name In Python
Answer#
Descriptive: Naming Styles in Python
The following special forms using leading or trailing underscores are recognized :
_single_leading_underscore: weak internal use indicator. E.g. from M import * does not import objects whose name starts with an underscore.
single_trailing_underscore_: used by convention to avoid conflicts with Python keyword, e.g.
Tkinter.Toplevel
__double_leading_underscore: when naming a class attribute, invokes name mangling .
__double_leading_and_trailing_underscore__: magic objects or attributes that live in user-controlled namespaces. E.g. init, import or file. Never invent such names only use them as documented.
Note that names with double leading and trailing underscores are essentially reserved for Python itself: Never invent such names only use them as documented.
How To Crack Python Coding Interviews
Python developers are in high demand, and you must ace Python coding interview questions to land interviews at top tech firms. Python developer jobs have scaled over the last few years as the language is used in various coding scenarios, from games to web applications, by some of the world’s largest companies, including Netflix, PayPal, and Dropbox.
They hire Python developers to push the boundaries of emerging technologies such as data analytics, data science, AI, natural language processing, and AI/machine learning. If you’re preparing for a technical interview and use Python as your programming language, the Python coding interview questions below will help you understand what to expect. Learn what recruiters look for in Python developers, as well as the best tips for outperforming the competition.
If you are preparing for a tech interview for tech lead, software engineer, software developer, or engineering manager positions, check out our technical interview checklist, interview questions page, and salary negotiation e-book to get interview-ready!
Having trained over 10,000 software engineers, we know what it takes to crack the toughest tech interviews. Our alums consistently land offers from FAANG+ companies. The highest ever offer received by an IK alum is a whopping $1.267 Million!
At IK, you get the unique opportunity to learn from expert instructors who are hiring managers and tech leads at Google, Facebook, Apple, and other top Silicon Valley tech companies.
Also Check: How To Dress Casual For An Interview
Write A Python Program To Calculate The Square Root Of A Given Number
Here is the Python Program to calculate the square root:
# Input function to get the input from the usern = float)#Formula to calculate the square root of the numbern_sqrt = n ** 0.5#Printing the calculated square root of the given numberprint)
Output1 of the Python program to find the square root
Enter a number: 2The square root of 2.0 is 1.4142135623730951
Output2 of the Python program to find the square root
Enter a number: 4The square root of 4.0 is 2.0
What Are Python Namespaces And Explain How To Use Namespaces & How This Feature Makes Programming Better
Answer#
Namespace is a way to implement scope.
In Java the compiler determines where a variable is visible through static scope analysis.
In C, scope is either the body of a function or its global or its external. The compiler reasons this out for you and resolves each variable name based on scope rules. External names are resolved by the linker after all the modules are compiled.
In Java, scope is the body of a method function, or all the methods of a class. Some class names have a module-level scope, also. Again, the compiler figures this out at compile time and resolves each name based on the scope rules.
In Python, each package, module, class, function and method function owns a namespace in which variable names are resolved. Plus theres a global namespace thats used if the name isnt in the local namespace.
Each variable name is checked in the local namespace , and then checked in the global namespace.
Variables are generally created only in a local namespace. The global and nonlocal statements can create variables in other than the local namespace.
When a function, method function, module or package is evaluated a namespace is created. Think of it as an evaluation context. When a function or method function, etc., finishes execution, the namespace is dropped. The variables are dropped. The objects may be dropped, also.
Read Also: What To Ask During Exit Interview
How Would You Use *args And **kwargs In A Given Function
*args and **kwargs make Python functions more flexible by accepting a variable number of arguments.
- *args pass a variable number of non-keyworded arguments in a list
- **kwargs pass a variable number of keyword arguments in a dictionary
Heres an example of a function that takes a variable number of keyworded arguments that are collected in a dictionary called data
Python Coding Questions On Decorators And Itertools
You have to practice Python coding challenges based on decorators and itertools to create an impression on the interviewer. You will be asked to write a program in Python to –
Also, read about how long does it take to learn Python.
Read Also: How To Prepare For Consulting Interview
What Is The Meaning Of Single And Double Underscore Before An Object Name In Python
Answer#
Single Underscore In a class, names with a leading underscore indicate to other programmers that the attribute or method is intended to be used inside that class.
However, privacy is not enforced in any way. Using leading underscores for functions in a module indicates it should not be imported from somewhere else.
From the PEP-8 style guide:
_single_leading_underscore: weak internal use indicator. E.g. from M import * does not import objects whose name starts with an underscore.
Double Underscore
From the Python docs:
Any identifier of the form __spam is textually replaced with _classname__spam, where classname is the current class name with leading underscore stripped.
This mangling is done without regard to the syntactic position of the identifier, so it can be used to define class-private instance and class variables, methods, variables stored in globals, and even variables stored in instances. private to this class on instances of other classes.
And a warning from the same page:
Name mangling is intended to give classes an easy way to define private instance variables and methods, without having to worry about instance variables defined by derived classes, or mucking with instance variables by code outside the class.
Note that the mangling rules are designed mostly to avoid accidents it still is possible for a determined soul to access or modify a variable that is considered private.
Example:
What Is The Difference Between Ndarray And Array In Numpy

Where is their implementation in the NumPy source code?
Answer#
numpy.array is just a convenience function to create an ndarray it is not a class itself.
You can also create an array using numpy.ndarray, but it is not the recommended way. From the docstring of numpy.ndarray:
Arrays should be constructed using array, zeros or empty The parameters given here refer to a low-level method ) for instantiating an array.
Recommended Reading: Instacart In Store Shopper Interview
How Do I Implement Interfaces In Python
Answer#
Interfaces are not necessary in Python. This is because Python has proper multiple inheritance, and also ducktyping, which means that the places where you must have interfaces in Java, you dont have to have them in Python.
That said, there are still several uses for interfaces. Some of them are covered by Pythons Abstract Base Classes, introduced in Python 2.6. They are useful, if you want to make base classes that cannot be instantiated, but provide a specific interface or part of an implementation.
Another usage is if you somehow want to specify that an object implements a specific interface, and you can use ABCs for that too by subclassing from them. Another way is zope.interface, a module that is a part of the Zope Component Architecture, a really awesomely cool component framework.
Here you dont subclass from the interfaces, but instead mark classes as implementing an interface. This can also be used to look up components from a component registry. Supercool!
What Is A Python Interview
If you are applying for a job that requires coding and programming, then a solid working knowledge of programming languages will be essential.
While there are many programming languages in use for different functions, one of the most popular and most sought after seems to be Python.
Python is a general-purpose coding language. It can be used for web development like HTML and JavaScript, but it can also be used for other types of programming and software development.
It is popular as a programming language because it uses simple syntax and can be used on multiple platforms Windows, Mac, Linux, Raspberry Pi, etc.
Python seems to be the top programming language in emerging data science fields, so experienced Python coders are future-proofed for the development of the Internet.
Python can be used for:
- Web development, on the back end/server-side
- Software development, like software apps
- Mathematical processes, such as big data computations
- Creating system scripts and instructions
Many big tech firms, including Google and Facebook, use Python in their stacks, and it could be used by beginner coders, web and mobile app developers, software engineers, data scientists… the list goes on.
Software engineers, programmers and coders are in high demand thousands of job opportunities exist in big tech firms and startups alike. However, this doesnt make recruitment any less competitive.
You May Like: What Questions Do I Ask The Interviewer
Is It A Good Idea To Use The Class As A Namespace In Python
Answer#
Yes, indeed. You can use Python classes strictly for namespacing as that is one of the special things they can do and do differently than modules. Its a lot easier to define a class as a namespace inline in a file than to generate more files.
You should not do it without commenting on your code saying what its for. Python classes come in a lot of different forms and purposes and this makes it difficult to understand code you have not seen before.
A Python class used as a namespace is no less a Python class than one that meets the perception of what a class is in other languages. Python does not require a class to be instantiated to be useful. It does not require ivars and does not require methods. It is fairly flexible.
Classes can contain other classes too.
Lots of people have their ideas about what is or isnt Pythonic. But if they were all worried about something like consistency, theyd push to have things like len dir, and help be a method of objects rather than a global function.
Iterate With Enumerate Instead Of Range
This scenario might come up more than any other in coding interviews: you have a list of elements, and you need to iterate over the list with access to both the indices and the values.
Theres a classic coding interview question named FizzBuzz that can be solved by iterating over both indices and values. In FizzBuzz, you are given a list of integers. Your task is to do the following:
Often, developers will solve this problem with range:
> > > numbers=> > > foriinrange):... ifnumbers%3==0andnumbers%5==0:... numbers='fizzbuzz'... elifnumbers%3==0:... numbers='fizz'... elifnumbers%5==0:... numbers='buzz'...> > > numbers
Range allows you to access the elements of numbers by index and is a useful tool for some situations. But in this case, where you want to get each elements index and value at the same time, a more elegant solution uses enumerate:
> > > numbers=> > > fori,numinenumerate:... ifnum%3==0andnum%5==0:... numbers='fizzbuzz'... elifnum%3==0:... numbers='fizz'... elifnum%5==0:... numbers='buzz'...> > > numbers
For each element, enumerate returns a counter and the element value. The counter defaults to 0, which conveniently is also the elements index. Dont want to start your count at 0? Just use the optional start parameter to set an offset:
Also Check: How To Answer Interview Questions For Manager Position
How To Send An Email With Python Python Code For Sending An Email
Answer#: Python program for sending an email.
We recommend using the standard packages email and smtplib together to send emails.
Please look at the following example .
Notice that if you follow this approach, the simple task is indeed simple, and the more complex tasks are accomplished very rapidly.
# Import smtplib for the actual sending functionimport smtplib# Import the email modules we'll needfrom email.mime.text import MIMEText# Open a plain text file for reading. For this example, assume that# the text file contains only ASCII characters.with open as fp: # Create a text/plain message msg = MIMEText)# me == the sender's email address# you == the recipient's email addressmsg = 'The contents of %s' % textfilemsg = memsg = you# Send the message via our own SMTP server, but don't include the# envelope header.s = smtplib.SMTPs.sendmail)s.quit
For sending emails to multiple destinations, you can follow the below Python example:
As you can see, the header To in the MIMEText object must be a string consisting of email addresses separated by commas. On the other hand, the second argument to the sendmail function must be a list of strings .
So, if you have three email addresses: , , and , you can do as follows :