How Do You Implement A Bucket Sort Algorithm
The Bucket sort is another awesome algorithm that can sort an array without even comparing elements. Its known as a non-comparison sorting algorithm and can give O performance for the selected input.
If you dont know about the non-comparison-based sorting Algorithm, please see Introduction to Algorithms book.
What Kind Of Support Do You Think Management Should Give You For Improving Your Problem Solving
A candidate’s answer to this problem solving interview question demonstrates how much they might demand from management. An assertive answer indicates an applicant who has confidence in their value and abilities and is willing to demand a supportive environment for their work. What to look for in an answer:
-
Assertive, confident answer
-
Clear communicator
Example:
If management is hiring me for a position with certain high-level responsibilities, Id definitely hope they can provide me with the resources and technical support to do my job smoothly. I also expect to be treated with respect, without being blamed for any problems that arent due to something I did wrong.
How Do You Reverse A Linked List In Java
LinkedList descendingIterator returns an iterator that iterates over the element in reverse order. The following example code shows how to use this iterator to create a new Linked List with elements listed in the reverse order:
LinkedList< Integer> ll =newLinkedList< > ll.add ll.add ll.add System.out.println LinkedList< Integer> ll1 =newLinkedList< > ll.descendingIterator.forEachRemaining System.out.println
Learn more about reversing a linked list from a data structures and algorithms perspective.
Read Also: Geeks For Geeks Interview Questions
Communication Be Clear & Concise
Coding is not only a way of communicating your requirements to the machine, but also a way of communicating your intent to fellow team members.
For each programming exercise the candidates solve, both on the automated Codility coding assessments, online CodeLive video interview, and in face-to-face interviews, we look at the code they write and assess whether it speaks to us clearly. If you look at the code and you immediately know whats going on, thats a plus, if you dont thats a red flag.
Bonus points:
Greg is the founder and principal scientist at Codility.
Consider Using Star Interview Method

The STAR interview technique is a method of developing in-depth responses that thoroughly answer a hiring manager’s questions. You can provide a comprehensive approach by following these steps:
-
Situation: Describe an environment where you faced a challenge or where you handled a project. For instance, you could describe how you managed a team of programmers.
-
Task: Next, describe the roles you had during the event. Perhaps you had a deadline and had to lead the team to finish the project by the deadline.
-
Action: Describe how you completed the task. Focus on how you influenced the result as opposed to what your team did to overcome the challenge.
-
Result: Finally, describe the outcome of your approach to the task. You can also relate what you learned in the role.
You won’t need to answer every question using the STAR interview technique. Many Java coding interview questions are technical and require a more direct answer, but it’s usually best to use the STAR method when faced with a behavioral-based question.
Related:How To Use the STAR Interview Response
Nonverbal communication is one of many tools that can help you make a good impression in interviews and in your professional life. However, candidate assessments should be based on skills and qualifications, and workplaces should strive to be inclusive and understanding of individual differences in communication styles.
Don’t Miss: Data Science Internship Interview Questions
Whats The Runtime Of The Following Code
Josh Tucholski, Tech Elevator.
Josh Tucholski, Tech Elevator
What skillset is this testing for? Another common term thats used is algorithmic complexity. The question could be: If Im going to give someone a block of code, can you tell me what are the worst-case scenarios that I have to consider? Because thats what BigO and algorithmic complexity really mean.
Thats kind of a cue to the interviewee to think about, What are some of the worst-case or edge-case scenarios that I have to be aware of? And thats important, because those things, while they account for maybe one or two percent of the number of inputs, theyre the kinds of things that often will crash our programs.
Is this a good interview question? As software developers, we all have a tendency, when given a problem, to immediately jump into all of the what ifs and the edge cases that we have to worry about especially after weve been kind of burned a couple times. We do tend to try and problem solve and prevent those worst-case scenarios.
Being able to ask in plain English, What is this code doing and whats the worst-case scenario? is an easier way to communicate than to recall the difference between BigO or linear or logarithmic or n2 or exponential time, because then youre counting on someone having been exposed, again, to that type of formal education that covered that content.
How Do You Write An Interface With Default And Static Method
Java 8 introduced default and static methods in interfaces. This bridged the gap between interfaces and abstract classes. The following example code shows one way to write an interface with the default and static method:
publicinterfaceInterface1staticbooleanisNull}
Learn more about about default and static methods in interfaces in Java 8 interface changes.
Recommended Reading: How To Do A Perfect Interview
Explain What A Binary Search Tree Is
- A binary search tree is used to store data in a manner that it can be retrieved very efficiently.
- The left sub-tree contains nodes whose keys are less than the nodes key value.
- The right sub-tree contains nodes whose keys are greater than or equal to the nodes key value
Fig: Binary Search Tree
Full Stack Developer Job Guarantee Program
String Programming Interview Questions
1) Write code to check a String is palindrome or not? 2) Write a method which will remove any given character from a String? 3) Print all permutation of String both iterative and Recursive way? 4) Write a function to find out longest palindrome in a given string? 5) How to find the first non repeated character of a given String?6) How to count the occurrence of a given character in a String?7) How to check if two String are Anagram?8) How to convert numeric String to int in Java? 1) What is the difference between String, StringBuilder, and StringBuffer in Java?2) Why String is final in Java? 3) How to Split String in Java?4) Why Char array is preferred over String for storing password? Master the Coding Interview: Data Structures + Algorithms course by Andrei Negaoie20 string coding questions
Recommended Reading: Big Data Analytics Interview Questions
How Would You Solve The Problem Of A Complete Payment System Failure In The Middle Of A Busy Sales Hour At One Of Our Store Locations
How a candidate answers this question demonstrates their ability to give measured, thoughtful responses to unexpectedly specific questions. Its particularly useful when framed around issues that are somewhat outside of their experience. The quality of their answer will indicate how well they can think on their feet. What to look for in an answer:
-
Stays calm even with unexpected questions
-
Quickly comes up with a solution to the problem
-
Job-specific knowledge
Example:
I would rapidly set up a note and paper accounting system for all customers who wanted to pay in cash. For customers who wanted to pay by credit card, Id try to patch a digital transfer payments setup together to send all sales-related funds to one of our business accounts through a mobile platform of some kind.
How Would You Remove Duplicate Characters From A Given Array In Java
This is a pretty technical question, one that works well in a coding interview or more technical job interview with a member of the engineering team.
The correct answer recognizes that the problem in dealing with an array is not finding duplicate characters, but removing them. An array is a static, fixed-length data structure.
You cannot change its length. As a result, the solution to deleting duplicate characters is to find duplicate characters using the first non-repeated character and create a new array, copying content into that array.
If there are multiple duplicates, then the software engineer would need to come up with a strategy to minimize how many temporary arrays are created copying content can put a burden on both memory and CPU.
Recommended Reading: What Makes A Great Interview
Best Tips To Crack Coding Interviews In 2022
Here are a few of my practical tips to crack your next coding interview. These are hard-earned and I learned from my own mistakes and experience.
How Do You Implement A Binary Search In Java
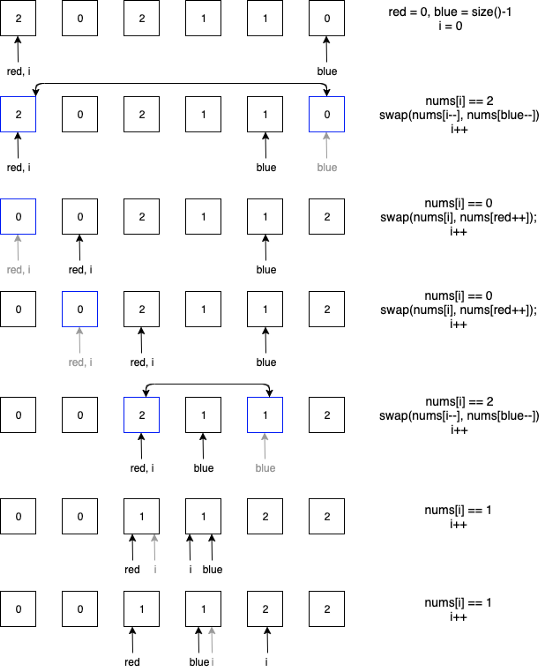
The array elements must be sorted to implement binary search. The binary search algorithm is based on the following conditions:
- If the key is less than the middle element, then you now need to search only in the first half of the array.
- If the key is greater than the middle element, then you need to search only in the second half of the array.
- If the key is equal to the middle element in the array, then the search ends.
- Finally, if the key is not found in the whole array, then it should return -1. This indicates that the element is not present.
The following example code implements a binary search:
publicstaticintbinarySearchelseifelsemid =/2 }ifreturn-1 }
Recommended Reading: Basic Coding Questions For Interview
Common Java Coding Interview Questions
Tegan Griffiths is a technical writer with a passion for everything from artificial learning to machine learning. Tegan currently writes content for an association magazine in the tech business.
Related: Top Interview Tips: Common Questions, Body Language & More
In this video, we dissect an entire job interview from start to finish. We analyze everything from common interview questions to etiquette and how to follow up.
If you’re preparing to interview for a job that requires knowledge of Java, it’s important to learn about some of the common questions the hiring manager may ask. Practicing your responses can help you feel more confident when responding to questions or writing test code. Though the questions the interviewer asks vary, you can practice your responses to help refresh your knowledge of Java.
In this article, we review why Java is a popular language among employers and provide examples of Java coding interview questions and answers to help you prepare.
Please note that none of the organizations mentioned in this article are affiliated with Indeed.
Difference Between Comparison And Non
As the name suggests, in comparison-based sorting algorithms, you must compare elements to sort like quicksort, but in non-comparison-based sorting algorithms like Counting sort, you can sort elements without comparing them. Surprised?
Well yes, then I suggest you check out this course to learn more about sorting algorithms like Radix Sort, Counting Sort, and Bucket Sort. You can further seeData Structures and Algorithms: Deep Diveif you want to learn more about these sorting algorithms.
Also Check: Behavioral Interview Questions For Product Managers
Coding Interview Questions: Topics To Prepare
To crack coding interviews at FAANG+ companies, it is important to know what topics to prepare. Below is the list of topics to cover in order to be able to solve coding interviews at FAANG+ companies.
- Graph algorithms, including greedy algorithms
- Dynamic programming
For a comprehensive checklist of all coding interview topics you should prepare, check out the technical interview checklist.
Common Java Code Interview Questions
Interviewers usually ask basic Java code questions in interviews along with some theoretical aspects. You might be asked questions or tasks like these:
Write a Java program to swap two numbers using the third variable.
Write a Java program to swap two numbers without using the third variable.
Write a Java program to find whether a number is prime or not.
Write a Java program to find the duplicate characters in a string.
Write a Selenium code to switch to the previous tab.
What is an abstract class? How are abstract classes similar or different in Java from C++?
How is inheritance in C++ different from Java?
What is object cloning?
What are the differences between HashMap and HashTable in Java?
Can you overload or override static methods in Java?
Don’t Miss: Interview Questions Learning And Development
Break The Question Down Into Smaller Independent Parts
If the problem is large, start with a high-level function and break it down into smaller constituting functions, solving each one separately. This prevents you from getting overwhelmed with the details of doing everything at once and keeps your thinking structured.
Doing so also makes it clear to the interviewer that you have an approach, even if you don’t manage to finish coding all of the smaller functions.
Example
The Group Anagrams problem can be broken down into two parts – hashing a string, grouping the strings together. Each part can be solved separately with independent implementation details. You could start off with this code:
defgroup_anagrams: strings_hashes =return group_strings
And proceed to fill in the implementation of each function. However, do note that sometimes the most efficient solutions will require you to break some abstractions and do multiple operations in one pass of the input. If your interviewer asks you to optimize based on your well-abstracted solution, that is one possible path forward.
What Has Been One Of The Most Common Management
A candidates answer to this problem solving interview question indicates their ability to tell managers and supervisors about what they think needs to be improved. An assertive answer shows experience and confidence. It also might indicate what an employee expects from his or her superiors on the job. What to look for in an answer:
-
Confident response
Example:
I absolutely expect management to give me the space and support to do my job and to not micromanage or second guess every decision I make. I hope that any office politics or failures by others are kept from interfering with my work as much as possible.
You May Like: The Last Interview Eshkol Nevo
What Is A Linked List
- Like an array, a linked list refers to a linear data structure in which the elements are not necessarily stored in a contiguous manner.
- It is basically a sequence of nodes, each node points towards the next node forming a chain-like structure.
Fig: Linked List
- LIFO is an abbreviation for Last In First Out
- It is a way of accessing, storing and retrieving data.
- It extracts the data that was stored last first.
Given An Array Of Integers Every Element Appears Twice Except For One Find That One
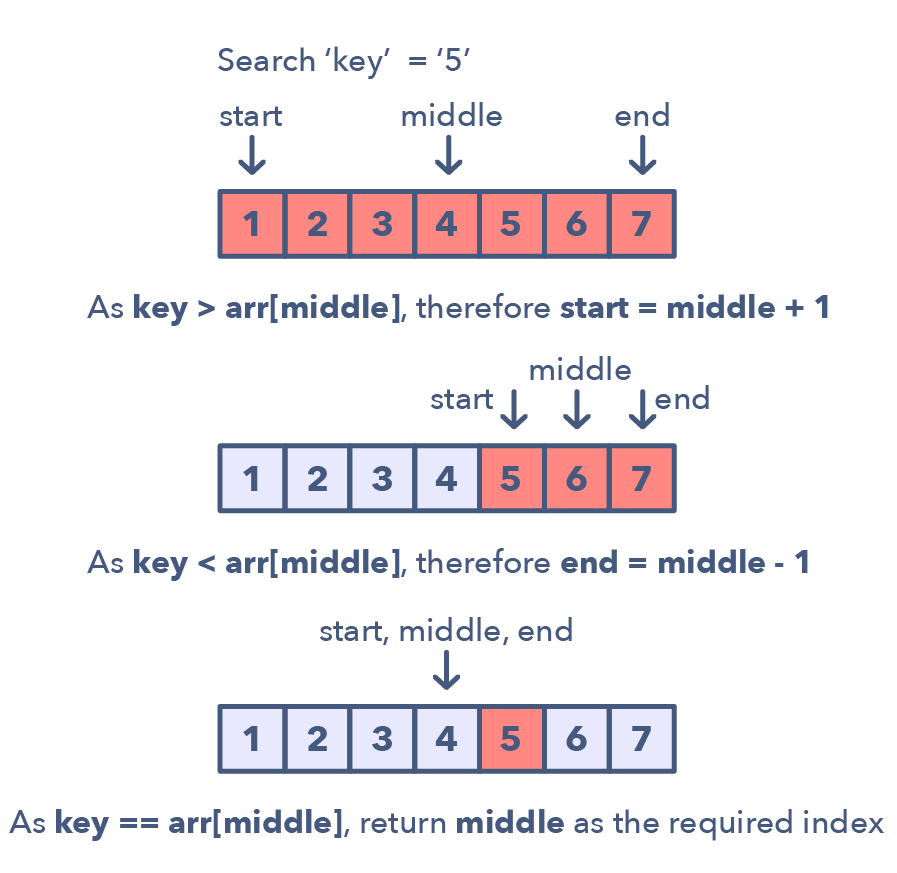
Anil Kadimisetty, director of engineering at Chainalysis
What skillset is this testing for? Not every interview candidate who gets selected actually solves a problem. That actually doesnt matter that much. Because in the real world, a single individual does not know the answers to everything theyre trying to solve. You are going to only succeed when youre able to collaborate with others, get others feedback and act on that. When you get stuck, what do you do? If someone is giving you a hint, are you responding well to the hint? That means youre probably great at collaborating later on when you come here.
Is this a good interview question? At Chainalysis, were about cryptocurrency. In an interview, if we ask about that because thats what we do on a day-by-day basis most people would fail, because no one really knows about cryptocurrencies.
So what you do is, you use the most abstract way of asking about those things without mentioning the topics, which are domain specific. If you abstract the domain knowledge out of it, you will come down to a basic problem like this. So thats what this is. Its actually making the hiring bar common across all candidates with different domain experiences. This is a good equalizer.
Recommended Reading: How To Prepare For Salesforce Developer Interview
Now Youre Ready For The Coding Interview
These are some of the most common questions outside of data structure and algorithms that help you to do really well in your interview.
I have also shared a lot of these questions on my blog, so if you are really interested, you can always go there and search for them.
These common coding, data structure, and algorithm questions are the ones you need to know to successfully interview any company, big or small, for any level of programming job.
If you are looking for a programming or software development job in 2019, you can start your preparation with this list of coding questions and if you are ready for an Interview then you can also take TripleBytes quiz and go directly to the final round of interviews with top tech companies like Coursera, Adobe, Dropbox, Grammarly, and many more.