What Is The Difference Between A Class Method And A Static Method When Should You Use Which
A static method is a method that knows anything about the class or the instance that had called it. Its a method that logically belongs to the class but doesnt have implicit arguments. A static method can be either called on the class or any of its instances.
A class method is a method that gets passed the class it was called on, much like selfis passed to other instance methods in a class. The mandatory argument of a class method isnt a class instance: its actually the class itself.
A typical use-case of class methods is providing an alternative way to construct instances: a class method that does this is known as a factory of the class.
Heres an Employee class that uses a class method that creates an instance in a slightly different way than the main constructor of the class.
What Are The Applications Of Graph Data Structure
- Transport grids where stations are represented as vertices and routes as the edges of the graph
- Utility graphs of power or water, where vertices are connection points and edge the wires or pipes connecting them
- Social network graphs to determine the flow of information and hotspots
- Neural networks where vertices represent neurons and edge the synapses between them
Top 30+ Data Structure Interview Questions And Answers
This tutorial provides frequently asked Data Structure interview questions and answers with explanations to help you prepare for the interview:
Computer programs use data that gets converted into binary and digital format so that computers understand its value.
Computer software and applications are developed using binary data as a reference or as information that will be processed by a computer in a central processing unit. The method to store, organize and efficiently use this data is termed a data structure.
There are many ways data can be organized in memory in order to utilize it efficiently without occupying space or memory of storage. There are a set of rules and sequences applied by a computer in computing this data, known as an algorithm.
Algorithm and Data Structure are the foundation for understanding how data is organized, stored, and optimized for efficient digital outcomes.
What You Will Learn:
Read Also: What Do You Wear To An Interview
What Is The Difference Between Linear And Non
A data structure that stores data in a linear order is known as a linear data structure.You can only traverse the data structure using that linear series.Arrays and stacks are examples of linear data structures.Data is organised in non-linear ways with non-linear data structures.For example, a graph is made up of nodes connected by edges.The edges that connect the nodes, not the order in which they are joined, determine the relationships between the data values.Trees are examples of non-linear data structures.
Using List Comprehension Print The Odd Numbers Between 0 And 100

List comprehensions are a feature in Python that allows us to work with algorithms within the default list data structure in Python. Here, were looking for odd numbers.
A list comprehension allows us to simplify our range and filtering algorithm so we can pack it into one line of code. This piece of code returns every element between 0 and 100 if it is divisible by 2.
You should be prepared to describe the list comprehension in detail and with the right notation.
Also Check: How To Get Prepared For An Interview
What Is The Difference Between Django Pyramid And Flask
Flask is a microframework primarily build for a small application with simpler requirements. In a flask, you dont have to use external libraries. Flask is ready to use.
Pyramids are built for larger applications. It provides flexibility and lets the developer use the right tools for their project. The developer can choose the database, URL structure, templating style, and more. Like Pyramid, Django can also be used for larger applications. It includes an ORM.
What Is Python Panda Used For
Ans: Pandas is a data manipulation and analysis software library for the Python programming language. It includes data structures and methods for manipulating numerical tables and time series, in particular. Pandas is open-source software licensed under the BSD three-clause license.
Also Read Related Article: Python Tutorial |
Also Check: What Are Frequently Asked Questions In A Job Interview
What Is The Distinction Between Python Arrays And Python Lists
Arrays in Python can only include components of the same data type, hence the data type of the array must be homogeneous.It’s a small wrapper for C language arrays that utilises a fraction of the memory of lists.
Lists in Python can contain components of several data types, making list data types heterogeneous.
importarraya=array.arrayforiina:print#OUTPUT: 1 2 3a=array.array#OUTPUT: TypeError: an integer is required a=foriina:print#OUTPUT: 1 2 string
Describe The Different Kinds Of Tree Traversals For A Binary Search Tree
There are three ways to traverse a tree. They are:
Inorder Traversal
Traverse the tree starting at the left subtree, then to the root of the tree, and finishing off at the right subtree.
Preorder Traversal
Preorder traversal starts at the root, then moves to the left subtree and finally the right subtree.
Postorder Traversal
This involves covering the tree starting from the left subtree and moving to the right subtree. You then move from the right subtree to the root to complete the traversal.
You May Like: What Are Good Interview Questions To Ask The Interviewee
Are Stack And Queue The Same Thing If Not Explain Why
Stacks and queues are different data structures. The key differences between them are:
- Stacks follow a first-in-first-out logic whereas queues employ a last-in-first-out logic.
- You can insert and delete elements only at the top of a stack. On the other hand, elements are added at one end of a queue and deleted at the other.
- Stacks use only one pointer, which is for the top of the stack. Queues need two pointers to address the front of the queue and back of the queue.
- Stacks are mainly used in problems requiring recursion whereas queues are better used in applications that require sequential processing.
Question 2: How Is Try/except Used In Python
An exception is an error that occurs while the program is executing. When this error occurs, the program will stop and generate an exception which then gets handled in order to prevent the program from crashing.
The exceptions generated by a program are caught in the try block and handled in the except block.
-
Try: Lets you test a block of code for errors.
-
Except: Lets you handle the error.
Don’t Miss: What Are The Most Popular Interview Questions
Ace Your Next Technical Interview
If youâre looking for guidance and help with your Python data structure interview prep, As pioneers in the field of technical interview prep, we have trained thousands of software engineers to crack the most challenging coding interviews and land jobs at their dream companies, such as Google, Facebook, Apple, Netflix, Amazon, and more!
What Are The Various Approaches For Developing Algorithms

There are 3 main approaches to developing algorithms:
- Divide and Conquer: Involves dividing the entire problem into a number of subproblems and then solving each of them independently.
- Dynamic Programming: Identical to the divide and conquer approach with the exception that all subproblems are solved together
- Greedy Approach: Finds a solution by choosing the next best option.
Don’t Miss: How To Perform In Interview
Mention Different Types Of Data Structures In Panda
Ans: Series and DataFrames are the two types of data structures that the Pandas library supports. Numpy serves as the foundation for both data structures. A DataFrame is a two-dimensional data structure in Pandas, while a Series is a one-dimensional data structure. A panel, a three-dimensional data structure that includes items, a major axis, and a minor axis, is another axis label.
Q25 Is Indentation Required In Python
Ans: Indentation is necessary for Python. It specifies a block of code. All code within loops, classes, functions, etc is specified within an indented block. It is usually done using four space characters. If your code is not indented necessarily, it will not execute accurately and will throw errors as well.
Don’t Miss: What Questions Do You Ask In An Interview
What Is A Binary Search When Is It Best Used
A binary search is an algorithm that starts with searching from the middle element. If the middle element is not the target element then it checks if it should search the lower half or the higher half. The process continues until the target element is found.
The binary search works best when applied to a list with sorted or ordered elements.
What Is Tuple Matching In Python
Tuple Matching in Python is a method of grouping the tuples by matching the second element in the tuples. It is achieved by using a dictionary by checking the second element in each tuple in python programming. However, we can make new tuples by taking portions of existing tuples.
Syntax:To write an empty tuple, you need to write as two parentheses containing nothing-tup1 =
Read Also: How To Prepare For A Dispatcher Interview
How Does The Selection Sort Work
Selection sort works by repeatedly picking the smallest number in ascending order from the list and placing it at the beginning. This process is repeated moving toward the end of the list or sorted subarray.
Scan all items and find the smallest. Switch over the position as the first item. Repeat the selection sort on the remaining N-1 items. We always iterate forward and swap with the smallest element .
Time complexity: best case O worst O
Space complexity: worst O
Can You Explain With An Example How Does A Variable Declaration Activity Will Consume Or Affect The Memory Allocation
The amount of space or memory is occupied or allocated depends upon the data type of the variables that are declared. So lets explain the same by considering an example: Lets say the variable is declared as an integer type then 32 bits of memory storage is allocated for that particular variable.
So based on the data type of the variable, the memory space will be allocated.
Explore Core Java Programming Tutorial For More Information. |
Recommended Reading: How To Crack Apple Interview
What Are Different Operations Available In Queue Data Structure
- enqueue: This adds an element to the rear end of the queue. Overflow conditions occur if the queue is full.
- dequeue: This removes an element from the front end of the queue. Underflow conditions occur if the queue is empty.
- isEmpty: This returns true if the queue is empty or else false.
- rear: This returns the rear end element without removing it.
- front: This returns the front-end element without removing it.
- size: This returns the size of the queue.
What Are Binary Trees
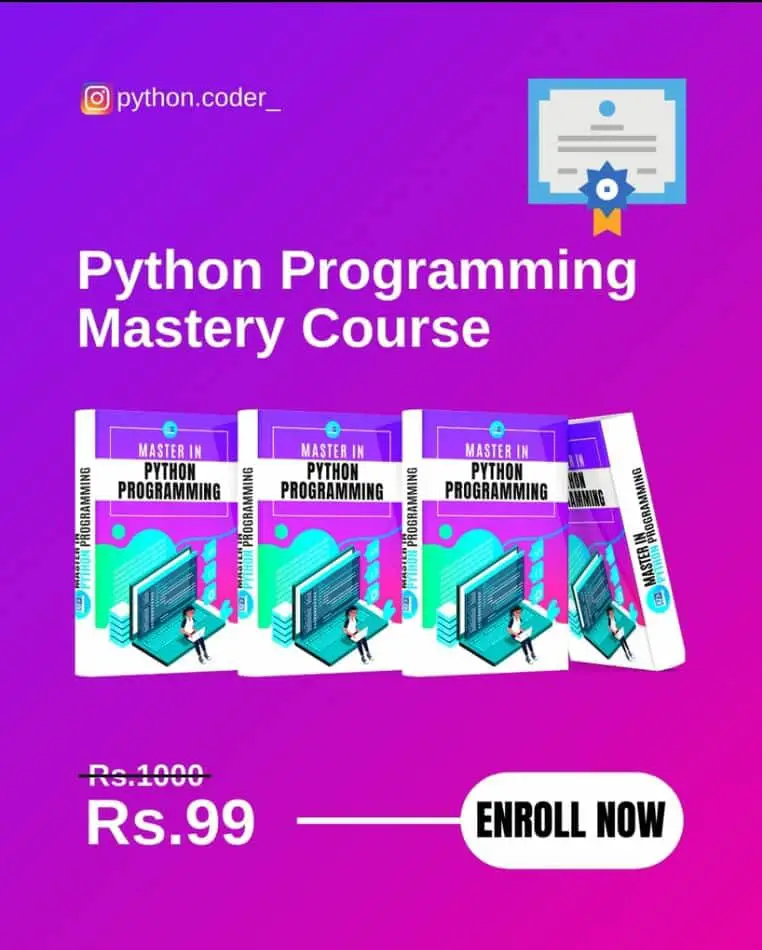
A binary tree is a tree data structure made up of nodes, each of which has two offspring, known as the left and right nodes. The tree begins with a single node called the root.
Each node in the tree carries the following information:
Data
A pointing device indicates the left kid.
An arrow pointing to the correct child
Don’t Miss: How To Prepare For A Qa Interview
List Out All Different Sorting Algorithms That Are Available And State Which Sorting Algorithm Is Considered As The Fastest
The list of all sorting algorithms are below:
Out of the above sorting options, none of the sorting algorithms can be tagged as the fastest algorithm, because each of these sorting algorithms is defined for a specific purpose. So based on the data structure and data sets available the sorting algorithms are used.
What Operations Can You Do With A Stack
A stack is a linear data structure that works on the same idea as a list, except that in a stack, components are only added and deleted from one end, the TOP.As a result, a stack is known as a LIFO data structure, because the last component inserted is the first component removed.A stack can carry out three basic operations:1. PUSH: When you use the push action, a new element is added to the stack.The new function is at the top of the priority list.However, before we insert the value, we must first verify if TOP=MAX1, since if it is, the stack is filled and no more insertions are possible. 2. POP: To remove the topmost member of a stack, use the pop action.We must first verify if TOP=NULL before deleting the item, because if it is, the stack is empty and no further deletions are permitted.You’ll get a UNDERFLOW notice if you try to remove a value from a stack that is already empty.
Read Also: How To Improve Interview Skills
What Are The Major Companies Hiring Data Science Experts In Chicago
The top firms hiring for the job title Data Science Experts are Mattersight, Uptake, and Allstate. Uptake has the highest reported compensation, with an average salary of $100,470. CCC Information Services and Allstate are firms that also pay well for this position. Doing a Data Science certification in Chicago would be a good choice to get a job in this field.
Important Python Data Structures Interview Questions And Answers
Python Data Structures is an important topic in every Python based Interviews hence it is absolutely necessary for a candidate to have complete understanding of the questions and answers that can be asked from this topic. In this article, I have gone through all the possible Python Data Structures questions based on my experience. Hopefully this will help you crack all kinds of Python based Interviews.
Recommended Reading: Google Product Manager Technical Interview
What Is A Queue How Is It Different From A Stack
A queue is a form of linear structure that follows the FIFO approach for accessing elements. Dequeue, enqueue, front, and rear are basic operations on a queue. Like a stack, a queue can be implemented using arrays and linked lists.
In a stack, the item that is most recently added is removed first. Contrary to this, in the case of a queue, the item least recently added is removed first.
These Are The Best Online Courses To Learn Data Structure And Algorithms In Python Algorithm Code Examples Are Given In The Python Programming Language
Hello Python Programmers, if you want to learn Data structure and Algorithms in 2022 and looking for the best online courses where you can find common data structure examples in Python then you have come to the right place.
In the past, I have shared a lot of useful resources like best data structure courses, books, and tutorials to learn Data Structure and Algorithms for programmers.
I have also shared a lot of Algorithmic interview questions and their solutions in Java, but I have been constantly getting queries about good courses to learn Data Structure and Algorithms in Python.
Even though the topics are completely independent of the programming language, Python developers definitely like the courses and books which teach Data Structure and Algorithms in Python.
This time, I have focused more on coverage of essential data structure in a fun and interesting way, rather than picking the course which covers a huge number of data structure and algorithms but didnt do justice with that.
Another reason I have included more than a few courses is because not everybody connects to the instructor I like. Everybody is different and they should only join the course where they can connect to the instructor, I mean they like his voice, the style of explanation, and the content.
Recommended Reading: How To Write A Post Interview Thank You Note
Explain About Multi Indexing In Pandas
Ans: Multiple indexing is classified as fundamental indexing because it simplifies information inspection and control, especially when dealing with higher-dimensional data. It also allows us to store and handle data in lower-dimensional data structures like series and dataframes with an unlimited number of measurements.
What Is A Priority Queue
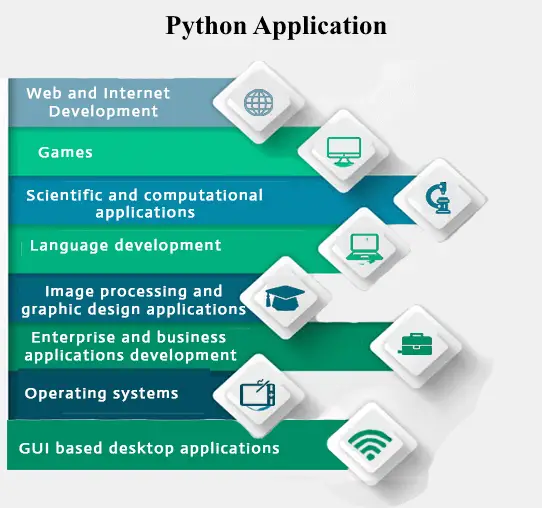
A priority queue is an abstract data type that resembles a normal queue but has a priority assigned to elements. Elements with higher priority are processed before the elements with a lower priority. A minimum of two queues are required in this case, one for the data and the other to store the priority.
Don’t Miss: Interview Questions For Nonprofit Executive Director
What Is Tuple In Python
A tuple is a built-in data collection type. It allows us to store values in a sequence. It is immutable, so no change is reflected in the original data. It uses brackets rather than square brackets to create a tuple. We cannot remove any element but can find in the tuple. We can use indexing to get elements. It also allows traversing elements in reverse order by using negative indexing. Tuple supports various methods like max, sum, sorted, Len etc.
To create a tuple, we can declare it as below.
Example:
Output:
6
It is immutable. So updating tuple will lead to an error.
Example:
Output:
tup=22 TypeError: 'tuple' object does not support item assignment
Python Certification Course Overview
This Python certification training will help you understand the high-level, general-purpose dynamic programming language of the decade. In this Python course, you will be exposed to both the basic and advanced concepts of Python such as Machine Learning, Deep Learning, Hadoop streaming, and MapReduce, and you will work with packages such as Scikit and SciPy.Read More
Recommended Reading: Interview Questions For Healthcare Recruiter
What Are The Advantages Of A Linked List Over An Array In Which Scenarios Do We Use Linked List And When Array
This is another frequently asked data structure interview question! Advantages of a linked list over an array are:
1. Insertion and Deletion
Insertion and deletion of nodes is an easier process, as we only update the address present in the next pointer of a node. Its expensive to do the same in an array as the room has to be created for the new elements and existing elements must be shifted.
2. Dynamic Data Structure
As a linked list is a dynamic data structure, there is no need to give an initial size as it can grow and shrink at runtime by allocating and deallocating memory. However, the size is limited in an array as the number of elements is statically stored in the main memory.
3. No Wastage of Memory
As the size of a linked list can increase or decrease depending on the demands of the program, and memory is allocated only when required, there is no memory wasted. In the case of an array, there is memory wastage. For instance, if we declare an array of size 10 and store only five elements in it, then the space for five elements is wasted.
4. Implementation
Data structures like stack and queues are more easily implemented using a linked list than an array.
Some scenarios where we use linked list over array are:
Some scenarios in which we use array over the linked list are:
In summary, we consider the requirements of space, time, and ease of implementation to decide whether to use a linked list or array.
The Ultimate Data Science Job Guarantee Program