What Are The Important Points To Keep In Mind For The Mediator Design Pattern
- This Pattern is used when there are little complications in the communication logic between two Objects.
- The mediator pattern is used by Java Message Service to publish data to other applications.
- One should refrain from using Mediator Design Pattern to achieve loose coupling as the process can get a little complicated in the future.
Design Thinking Certification Training Course
Explain Mvc Pattern In Java
MVC Pattern stands for Model-View-Controller Pattern. This pattern is used to separate applicationâs concerns.
Model â Model represents an object or JAVA POJO carrying data. It can also have logic to update controllers if its data changes.
View â View represents the visualization of the data that the model contains.
Controller â Controller acts on both model and view. It controls the data flow into the model object and updates the view whenever data changes. It keeps view and model separate.
What Is The Dependency Injection Pattern
Dependency Injection Pattern helps transform our application from a complex one to a loosely coupled one. It allows for removing hard-coded dependencies. It ensures that the application is extendable and maintainable. Dependency Injection Pattern can be injected in Java to shift the dependency resolution from compile-time to runtime.
Don’t Miss: What To Ask In A Job Interview Employer
What Problem Does Builder Pattern Try To Solve
A builder pattern is a type of creational design pattern that lets to construct complex objects in a step by step manner. The pattern lets to produce different representations of an object using the same construction logic. It helps in creating immutable classes having a large set of attributes. In the Factory and Abstract Factory Design Patterns, we encounter the following issues if the object contains a lot of attributes:
- When the arguments are too many, the program will be error-prone while passing from the client to the Factory Class in a specific order. It becomes tedious to maintain the order of arguments when the types are the same.
- There might be some optional attributes of the object and yet we would be forced to send all parameters and optional attributes as Null.
- When the object creation becomes complex due to heavy attributes, the complexity of this class would become confusing.
The above problems can also be solved by using constructors of required parameters alone. But this causes an issue when there would be new parameters that are added as part of new requirements. This would result in inconsistency. Thats where Builder comes into the picture. This pattern solves the issue of a large number of optional attributes and the inconsistent state by providing means to build an object in a step-by-step way and return the final object utilizing another method.
Builder pattern can be implemented by following the below steps:
The output of the above code would be:
Q Explain Singleton Design Pattern In Java

1. Eager initialization:In eager initialization, the instance of Singleton Class is created at the time of class loading.
Example:
publicclassEagerInitializedSingleton publicstaticEagerInitializedSingletongetInstance}
2. Static block initializationStatic block initialization implementation is similar to eager initialization, except that instance of class is created in the static block that provides option for exception handling.
Example:
publicclassStaticBlockSingleton // static block initialization for exception handlingstaticcatch }publicstaticStaticBlockSingletongetInstance}
3. Lazy InitializationLazy initialization method to implement Singleton pattern creates the instance in the global access method.
Example:
publicclassLazyInitializedSingleton publicstaticLazyInitializedSingletongetInstancereturninstance }}
4. Thread Safe SingletonThe easier way to create a thread-safe singleton class is to make the global access method synchronized, so that only one thread can execute this method at a time.
Example:
publicclassThreadSafeSingleton publicstaticsynchronizedThreadSafeSingletongetInstancereturninstance }}
5. Bill Pugh Singleton ImplementationPrior to Java5, memory model had a lot of issues and above methods caused failure in certain scenarios in multithreaded environment. So, Bill Pugh suggested a concept of inner static classes to use for singleton.
Example:
Don’t Miss: How To Rock An Interview
What Is Abstract Factory Pattern
Abstract Factory Pattern states that define an abstract class or interface for creating families of related objects but without specifying their concrete sub-classes. That means Abstract Factory allowed a class to return a factory of classes. That is why the Abstract Factory Pattern is one level higher than the Factory Pattern.
- Abstract Factory patterns work around superclasses, which creates other classes.
- The Abstract Factory Pattern comes under Creational Pattern as this pattern provides one of the best ways to create an object.
- In the Abstract Factory pattern, an interface is liable for creating a factory of related objects without explicitly identifying their classes.
- Each generated factory can give the objects according to the Factory pattern.
Describe The Open Close Principle
Open-Closed Principle states or ensures that class, component, or entity should be open for extension but close for modification.
In detail, We can extend any class via Interface, Inheritance. or Composition whenever itâs required instead of opening a class and modifying its code.
For example, suppose you have implemented functionality to calculate the area of the Rectangle and after some time you need to calculate the area of the Square, then, In this case, you
should not modify your original class code to add extra code for square. Instead, you should create one base class initially and now you should extend this base class by
your square class.
Also Check: What Are Interviewers Looking For
Consider A Scenario Where You Are Writing Classes For Providing Market Data And We Have The Flexibility To Switch To Different Vendors Or We Can Be Directed To The Direct Exchange Feed How Will You Approach This Problem To Design The System
We can do this by having an interface called MarketData which will consist of the methods required by the Client. The MarketData should have the MarketDataProvider as the dependency by employing Dependency Injection. This ensures that even if the provider changes, the market data will not be impacted.
The implementation of this problem is left as an exercise to the reader.
What Are The Advantages Of Java Design Patterns
Design patterns are template-based reusable solutions to help developers work effortlessly in multiple projects. In Java, the design patterns are flexible and help to identify unwanted repetitive code easily. The architecture of the software can be customised as per the requirements. Some of the advantages of using design patterns in Java are:
- They are reusable and can be used in multiple projects.
- They provide template solutions for defining system architecture.
- They provide transparency to software design.
- They are well-tested and proven means of developing robust solutions effortlessly.
You May Like: What To Say In Thank You For Interview
What Is An Adapter Design Pattern
The adapter design pattern falls under the category of a structural design pattern that lets incompatible objects collaborate. It acts as a wrapper between 2 different objects. The adapter catches the call for one object and transforms them to be recognizable by the second object.
Let us understand this with the help of an example of a USB to Ethernet adapter that is used when we have an ethernet interface at one end and the USB interface on the other end. The USB and ethernet are incompatible with each other which is why we require an adapter. The adapter class has a Client class that expects some object type and it has an Adaptee class that offers the same feature but by exposing a different interface. Now to make these both communicate, we have an Adapter class. The client requests the Adapter by using the target interface. The Adapter class translates the request using the Adaptee Interface on the adaptee. The Client receives the results unaware of the adapters role. This has been described in the class diagram as shown below:
Class Diagram:
//MediaPlayer.javapublicinterfaceMediaPlayer
//AdvancedPlayer.javapublicinterfaceAdvancedPlayer
//AudioPlayer.javapublicclassAudioPlayerimplementsMediaPlayer //Make use of Adapter to support different formatselseif || format.equalsIgnoreCase)else } }
//Driver.javapublicclassDriver}
The output of this code would be:
MP3 file music1.mp3 Playing...WAV File music2.wav Playing...MP4 File music3.mp4 Playing...Format not supported
What Is A Bridge Design Pattern
The bridge pattern is a type of structural design pattern that lets to split large class or closely related classes into 2 hierarchies – abstraction and implementation. These hierarchies are independent of each other and are used whenever we need to decouple an abstraction from implementation. This is called a Bridge pattern because it acts as a bridge between the abstract class and the implementation class. In this pattern, the abstract classes and the implementation classes can be altered or modified independently without affecting the other one.
The above image is the UML representation of the Bridge Pattern. There are 4 main elements of Bridge Pattern. They are:
- Abstraction This is the core of the pattern and it defines its crux. This contains a reference to the implementer.
- Refined Abstraction This extends the abstraction and takes refined details of the requirements and hides it from the implementors.
- Implementer This is the interface for the implementation classes.
- Concrete Implementation These are the concrete implementation classes that implement the Implementer interface.
Read Also: How To Prepare For Dba Interview
What Is A Command Pattern
The command pattern is a type of behavioural design pattern that transforms a request into a stand-alone object containing all the details about the request. This pattern is a data-driven pattern because we make use of the information about the request by wrapping it as an object and is passed to the invoker object as a command. The invoker object checks for the object that can handle the command and passes it to that object to execute the command. The following diagram is the UML diagram that represents the command design pattern.
We have a client that calls the invoker to run a command. We have a Command interface that acts as an abstraction to the underlying concrete classes. Let us understand this with the help of an example of remote control that has only one button. Using this button, we will be controlling the behaviour of two objects tubelight and a radio. The command to control the objects will be implemented using the command design pattern.
- Create Command interface:
// Command InterfaceinterfaceCommand
- Create Tubelight class and its command classes that extend the above interface to control the tubelight.
// Tubelight classclassTubeLightpublicvoidlightOff }// Command class to turn on the tubelightclassTubeLightOnCommandimplementsCommandpublicvoidexecute }// Command class to turn off the tubelightclassTubeLightOffCommandimplementsCommandpublicvoidexecute }
- Create a Radio class and its command classes that extend the above interface to control the radio.
Explain About Intercepting Filter Design Pattern
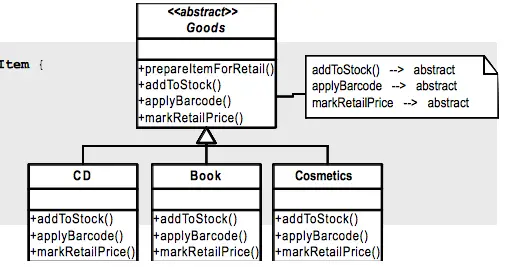
The intercepting filter design pattern is used when we want to do some pre-processing / post-processing with a request or response of the application.
Filters can do the authentication/ authorization/ logging or tracking of requests and then pass the requests to corresponding handlers.
Filter â Filter which will perform certain tasks prior to or after execution of request by the request handler.Filter Chain â Filter Chain carries multiple filters and helps to execute them in defined order on target.Target â The target object is the request handler.Filter Manager â Filter Manager manages the filters and Filter Chain.Client â The Client is the object who sends requests to the Target object.
Recommended Reading: What Are The Top 20 Questions Asked In An Interview
What Is Gang Of Four
A book titled Design Patterns Elements of Reusable Object-Oriented Software was published in 1994. The names of the authors were Erich Gamma, Ralph Johnson, Richard Helm, and John Vlissides. The book was based on establishing knowledge related to Design Patterns in Software Development. The four authors of this book are known as the Gang of Four .
What Is Decorator Design Pattern
- Create an interface and concrete classes that implement this interface.
- Create an abstract decorator class that implements the above interface.
- Create a concrete decorator class that extends the above abstract class.
- Use the concrete decorator class to decorate the interface objects and verify the output.
Let us understand this with the help of an example. Here, we will be creating a Shape Interface and concrete classes- Rectangle and Triangle that implement this Shape interface. We will be creating an abstract decorator class ShapeDecorator that implements the Shape interface. We will create RedColorDecorator that extends ShapeDecorator. We will be then using this decorator to implement the functionalities.
- Creating Shape Interface
// Shape.javapublicinterfaceShape
- Create concrete classes Rectangle.java and Triangle.java that implement Shape Interface.
// Rectangle.javapublicclassRectangleimplementsShape }
// Triangle.javapublicclassTriangleimplementsShape }
- Create ShapeDecorator abstract class that implements the Shape interface.
// ShapeDecorator.javapublicabstractclassShapeDecoratorimplementsShapepublicvoiddraw }
- Create the RedColorDecorator.java class that extends the ShapeDecorator class.
- Implement the Driver class to demo the decorator class.
// Driver.javapublicclassDriver }
- Validate the output. The output of the above code would be:
Don’t Miss: How To Interview For A Promotion
What Are The Advantages Of Composite Design Pattern In Java
Composite design pattern allows clients to operate collectively on objects that may or may not represent a hierarchy of objects.
Advantage of composite design patterns is as follows.
- It describes the class hierarchy that contains primitive and complex objects.
- It makes it easy to add new kinds of the component.
- It facilitates with the flexibility of structure with a manageable class or interface.
What Is The Mediator Design Pattern
As the name suggests, Mediator Design Pattern acts as the mid-point between different Objects in the system. This application turns out to be very helpful when varied Objects interact with each other. It steps in the process, leading to higher costs and more complexity. Instead of making Objects interact directly, leading to tightly coupled system components- the mediator aids in implementing loose coupling between Objects.
Read Also: How To Be Good In A Job Interview
Explain About Prototype Design Pattern
Prototype pattern refers to creating duplicate objects while keeping performance in mind.
This pattern involves implementing a prototype interface that tells it to create a clone of the current object. This pattern is used when the creation of an object directly is costly. For example, an object is to be created after a costly database operation. We can cache the object, return its clone on the next request and update the database as and when needed, thus reducing database calls.
Same way Hibernate improves performance by using cache and delaying database calls.
In Your Opinion What Are The Advantages Of The Builder Design Pattern
The interviewer may ask a question like this based on the pattern’s specificity of usage and the different dimensions and steps involved.
Example: “In my experience, I’ve found the builder pattern to be advantageous in that it encapsulates the construction and representation coding in separate containers to enable the construction process to produce multiple representations.
Also, I enjoy the improved control it gives me over the process of creating an object by breaking it up into steps to make it seem less complex than it is. It also reduces the required parameters for increased simplicity and readability and allows for immutable objects to be built into the pattern quickly.”
Related: Top .NET Interview Questions and Answers in 2022
You May Like: How To Conduct A Technical Interview
What Is The Observer Pattern
Observer Design Pattern is categorized under Behavioral Design Pattern. This process is helpful for those who are interested in understanding the state of an Object and want to stay updated on further changes. In this process, the Object that keeps an eye on the state of another Object is called an observer, and the Object which is watched is called the Subject.
What Is Factory Pattern

- It is the most used design pattern in Java.
- These design patterns belong to the Creational Pattern as this pattern provides one of the best ways to create an object.
- In the Factory pattern, we don’t expose the creation logic to the client and refer the created object using a standard interface.
- Factory Pattern allows the sub-classes to choose the type of objects to create.
- The Factory Pattern is also known as Virtual Constructor.
You May Like: How To Answer Interview Question Why Should We Hire You
What Is A Prototype Pattern
Suppose you have an Object creation in mind. However, it turns out that it is costly and drains plenty of your time and resources. But if you have a similar existing Object, Prototype Pattern can help you copy the original Object to a new Object. One can also modify it according to the needs. Java cloning is used by Prototype Pattern to copy the Object.
Top 15 Java Design Pattern Interview Questions And Answers
design pattern interview questionsRead Also : Singleton Design Pattern in JavaQ1 What are Java Design Patterns?Q2 What are the different categories of Design Patterns?1. Creational Patterns –2. Structural Patterns –3. Behavioral Patterns –Q3 What is Singleton Design Pattern?Q4 How to implement a Singleton Java class?
publicclassSingleton /* if object exists, return the existing object. If not, create and return a
new object.*/ publicstatic Singleton getInstancereturn instance } publicvoiddisplayMessage}
Q5 What happens if the method returning instance of Singleton class is not synchronized in multi-threaded environment?Q6 What are the different ways to create Singleton design pattern in Java?Eager LoadingLazy LoadingQ7 How can you create a thread safe Singleton class in Java?Double check locking method:
publicclassDoubleCheckSingleton{//reference variableprivatestatic DoubleCheckSingleton instance /*prevent instantiation from outside of
class by making constructor private*/privateDoubleCheckSingleton//instance accessor methodpublicstatic DoubleCheckSingleton getInstance}}return instance }//method with some logicpublicvoiddisplayMessage}
Enum singleton implementation:
publicenum EnumSingleton }
Synchronized instance accessor method:
publicclassSynchronizedSingleton{ //reference variable privatestatic SynchronizedSingleton instance /*prevent instantiation from outside of class
Q10 What is Factory Design Pattern?
Don’t Miss: What Are Some Common Interview Questions
What Is The Difference Between Design Patterns And Design Principles
Design patterns are known as the reusable template solutions used for common issues that can be customized as per the requirements. These can be implemented efficiently, are safe to use and can be tested properly.
On the other hand, design principles are followed when designing software systems for any sort of platform by using a programming language. The SOLID principles are the design principles that are followed as guidelines to develop extensive, scalable, and robust software systems. These principles can be applied to every aspect of programming.