Q 42 Why Are Generics Used In Java Programming
Compile-time type safety is provided by using generics. Compile-time type safety allows users to catch unnecessary invalid types at compile time. Generic methods and classes help programmers specify a single method declaration, a set of related methods, or related types with an available class declaration.
Q What Is Java Reflection Api
Java Reflection is the process of analyzing and modifying all the capabilities of a class at runtime. Reflection API in Java is used to manipulate class and its members which include fields, methods, constructor, etc. at runtime. The java.lang.Class class provides many methods that can be used to get metadata, examine and change the run time behavior of a class.
There are 3 ways to get the instance of Class class. They are as follows:
- forName method of Class class
- getClass method of Object class
- the .class syntax
1. forName method of Class class
- is used to load the class dynamically.
- returns the instance of Class class.
- It should be used if you know the fully qualified name of class.This cannot be used for primitive types.
classSimple classTest }
Output
Simple
2. getClass method of Object class
It returns the instance of Class class. It should be used if you know the type. Moreover, it can be used with primitives.
classSimple classTest publicstaticvoidmain }
Output
Simple
3. The .class syntax
If a type is available but there is no instance then it is possible to obtain a Class by appending “.class” to the name of the type.It can be used for primitive data type also.
classTest }
Output
How Is The Routing Carried Out In Mvc
The RouteCollection contains a set of routes that are responsible for registering the routes in the application. The RegisterRoutes method is used for recording the routes in the collection. The URL patterns are defined by the routes and a handler is used which checks the request matching the pattern. The MVC routing has 3 parameters. The first parameter determines the name of the route. The second parameter determines a specific pattern with which the URL matches. The third parameter is responsible for providing default values for its placeholders.
Recommended Reading: Cracking The Coding Interview Com
How We Can Set The Spring Bean Scope And What Supported Scopes Does It Have
A scope can be set by an annotation such as the @Scope annotation or the “scope” attribute in an XML configuration file. Spring Bean supports the following five scopes:
- Step 1: 163 => 1+6+3 = 10
- Step 2: 10 => 1+0 = 1 => Hence 163 is a magic number
publicclassIBMagicNumber sumOfDigits += num % 10 num /= 10 } // If sum is 1, original number is magic number if else }}
Example Java Basic Interview Questions And Answers
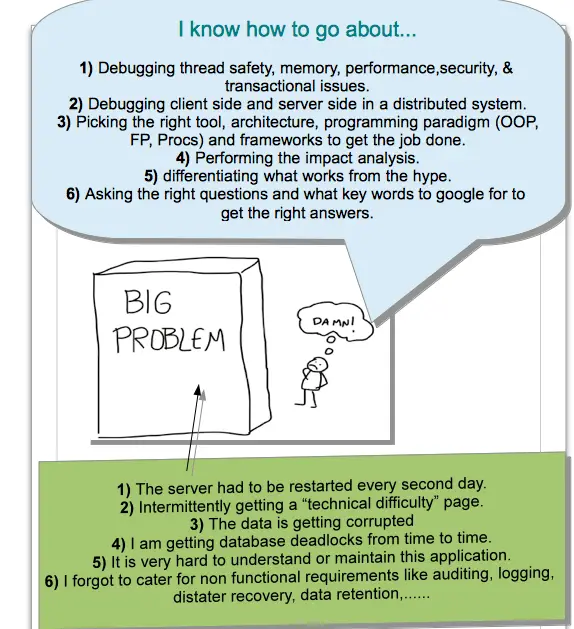
Note: Important keywords are underlined in the answers. Bonus points if the candidate mentions them!
Question 1: What is inheritance?Requirement: Object-Oriented Programming
Answer: Its the means by which a new class is derived from an existing class. These new classes can inherit or acquire the properties and methods of other classes. In other words, a subclass takes on the characteristics of its superclass or ancestor.
Question 2: Explain public static void main.Requirement: Foundational Java knowledge
Answer: This is a good basic Java interview question since its one of the first things you have to memorize when you code in this language.
public: This is an access modifier, which means it specifies who can access this method. It means that this method is accessible by any class.
static: This identifies your program as class-based that is, it can be accessed without first creating the instance of a class.
void: This defines the methods return type, and it says that the method wont return any value.main: This is the name of the method. JVM searches for this method as a starting point for an application with only this particular signature.
String args: The parameters that can be passed to the main method.
Question 3: What is an object?Requirement: Object-Oriented Programming
Answer: In Java, an object is an instance of a class. It has a state , and a behavior .
Question 5: How would you learn about a new Java class?Requirement: learning on the job
Read Also: What Are Questions You Get Asked At A Job Interview
What Is Encapsulation In Java
The idea behind encapsulation is to hide the implementation details from users. If a data member is private, it can only be accessed within the same class. No outside class can access private data member of other class.
However, if we set up public getter and setter methods to update )and read ) the private data fields then the outside class can access those private data fields via public methods.
Explain The Mvc Application Life Cycle
Web applications usually have 2 primary execution steps. These are:
- Understanding the request.
- Sending an appropriate response based on the type of request.
The same thing can be related to MVC applications also whose life cycle has 2 foremost phases:
- For creating a request object.
- For sending the response to any browser.
Don’t Miss: How To Reject Applicant After Interview
Q 52 Explain The Various Directives In Jsp
Directives are instructions processed by JSP Engine. After the JSP page is compiled into a Servlet, Directives set page-level instructions, insert external files, and define customized tag libraries. Directives are defined using the symbols below:
start with “< %@” and then end with “% > ”
The various types of directives are shown below:
- Include directive
It includes a file and combines the content of the whole file with the currently active pages.
Page Directive defines specific attributes in the JSP page, like the buffer and error page.
Taglib declares a custom tag library, which is used on the page.
Q21 What Are The Different Types Of Garbage Collectors In Java
Garbage collection in Java a program which helps in implicit memory management. Since in Java, using the new keyword you can create objects dynamically, which once created will consume some memory. Once the job is done and there are no more references left to the object, Java using garbage collection destroys the object and relieves the memory occupied by it. Java provides four types of garbage collectors:
- Serial Garbage Collector
Read Also: Hr Manager Interview Questions Shrm
Top 10 Java 8 Coding And Programming Interview Questions And Answers
Java 8 Interview questions and answerscoding and programming interview questions and answersRead Also:Java 8 Interview Questions and AnswersQ1 Given a list of integers, find out all the even numbers exist in the list using Stream functions?
importjava.util.* importjava.util.stream.* publicclassJavaHungry}
Output:10, 8, 98, 32 Q2 Given a list of integers, find out all the numbers starting with 1 using Stream functions?
importjava.util.* importjava.util.stream.* publicclassJavaHungry}
Output:10, 15Q3 How to find duplicate elements in a given integers list in java using Stream functions?
importjava.util.* importjava.util.stream.* publicclassJavaHungry}
Output:98, 15Q4 Given the list of integers, find the first element of the list using Stream functions?
importjava.util.* importjava.util.stream.* publicclassJavaHungry}
Output:10Q5 Given a list of integers, find the total number of elements present in the list using Stream functions?
importjava.util.* importjava.util.stream.* publicclassJavaHungry}
Output:9 Q6 Given a list of integers, find the maximum value element present in it using Stream functions?
importjava.util.* importjava.util.stream.* publicclassJavaHungry}
Output:98Q7 Given a String, find the first non-repeated character in it using Stream functions?
importjava.util.* importjava.util.stream.* importjava.util.function.Function publicclassJavaHungry}
Output:j Q8 Given a String, find the first repeated character in it using Stream functions?Output:a
Q How Can We Create An Immutable Class In Java
Immutable class means that once an object is created, we cannot change its content. In Java, all the wrapper classes and String class is immutable.
Rules to create immutable classes
- The class must be declared as final
- Data members in the class must be declared as final
- A parameterized constructor
Also Check: What Questions Will Be Asked In A Job Interview
Q What Is Difference Between String Stringbuilder And Stringbuffer
String is immutable, if you try to alter their values, another object gets created, whereas StringBuffer and StringBuilder are mutable so they can change their values.
The difference between StringBuffer and StringBuilder is that StringBuffer is thread-safe. So when the application needs to be run only in a single thread then it is better to use StringBuilder. StringBuilder is more efficient than StringBuffer.
Situations:
- If your string is not going to change use a String class because a String object is immutable.
- If your string can change and will only be accessed from a single thread, using a StringBuilder is good enough.
- If your string can change, and will be accessed from multiple threads, use a StringBuffer because StringBuffer is synchronous so you have thread-safety.
Example:
classStringExample // Concatenates to StringBuilder publicstaticvoidconcat2 // Concatenates to StringBuffer publicstaticvoidconcat3 publicstaticvoidmain }
Output
Explain Event Loop In Nodejs

The event loop in JavaScript enables asynchronous programming. With JS, every operation takes place on a single thread, but through smart data structures, we can create the illusion of multi-threading. With the Event Loop, any async work is handled by a queue and listener. Consider the diagram below:
Therefore, when an async function needs to be executed, the main thread relays it to another thread, allowing v8 to continue processing or running its code. In the event loop, there are different phases, like pending callbacks, closing callbacks, timers, idle or preparing, polling, and checking, with different FIFO queues.
Recommended Reading: How To Get Better At Coding Interviews
What Are The Main Differences Between The Java Platform And Other Platforms
There are the following differences between the Java platform and other platforms.
- Java is the software-based platform whereas other platforms may be the hardware platforms or software-based platforms.
- Java is executed on the top of other hardware platforms whereas other platforms can only have the hardware components.
What Is The Inheritance
Inheritance is a mechanism by which one object acquires all the properties and behavior of another object of another class. It is used for Code Reusability and Method Overriding. The idea behind inheritance in Java is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields of the parent class. Moreover, you can add new methods and fields in your current class also. Inheritance represents the IS-A relationship which is also known as a parent-child relationship.
There are five types of inheritance in Java.
- Single-level inheritance
Recommended Reading: Healthcare Data Analyst Interview Questions
Which Among String Or String Buffer Should Be Preferred When There Are Lot Of Updates Required To Be Done In The Data
StringBuffer is mutable and dynamic in nature whereas String is immutable. Every updation / modification of String creates a new String thereby overloading the string pool with unnecessary objects. Hence, in the cases of a lot of updates, it is always preferred to use StringBuffer as it will reduce the overhead of the creation of multiple String objects in the string pool.
What Is Lambda Expression In Java
A lambda expression describes a block of code that can be passed to constructors or methods for subsequent execution. The constructor or method receives the lambda as an argument. Consider the following example: System.out.println This example identifies a lambda for outputting a message to the standard output stream. From left to right, identifies the lambdas formal parameter list , -> indicates that the expression is a lambda, and System.out.println is the code to be executed.
Read Also: What Are Common Interview Questions
Applet Java Interview Questions
Q.55. What is an Applet?
Answer. An applet is a Java program that is embedded into a web page. An applet runs inside the web browser and works at the client-side. We can embed an applet in an HTML page using the APPLET or OBJECT tag and host it on a web server. Applets make the website more dynamic and entertaining.
Q.56. Explain the life cycle of an Applet.
Answer.
The above diagram shows the life cycle of an applet that starts with the init method and ends with destroy method. Other methods of life cycle are start, stop and paint. The methods init and destroy execute only once in the applet life cycle. Other methods can execute multiple times.
Below is the description of each method of the applet life cycle:
init: The init is the initial method that executes when the applet execution starts. In this method, the variable declaration and initialization operations take place.
start: The start method contains the actual code to run the applet. The start method runs immediately after the init method executes. The start method executes whenever the applet gets restored, maximized, or moves from one tab to another tab in the browser.
stop: The stop method is used to stop the execution of the applet. The stop method executes when the applet gets minimized or moves from one tab to another in the browser.
Q.57. What happens when an applet is loaded?
Q.58. What is the applet security manager? What does it provide?
Q.59. What are the restrictions put on Java applets?
Answer.
No. |
Need Help Hiring An Entry Level And/or A Seniorjava Developer
Suppose you are still wondering what are the basic questions asked in Java interviews, or how to conduct a more technical interview, including specific coding questions. In that case, your best option is to get help from experts. Having technical background during interviews is necessary, but also interviewers need specific skills to know when a candidate will fit into a companys culture and when they are not. Plenty of candidates have a solid skill set but hate code reviews or cant stand working on teams. But dont worry! At DistantJob, all of our headhunters are skilled enough to identify the most talented junior or senior Java remote developers that will fit your company perfectly. All you need to do is:
1. Contact us.
2. Tell us the skills you are looking for in candidates.
In less than two weeks, youll be onboarding your new entry-level Java developer.
or Share this post
Read Also: How To Prepare Yourself For An Interview
Which Of The Below Generates A Compile
We get a compile-time error in line 3. The error we will get in Line 3 is – integer number too large. It is because the array requires size as an integer. And Integer takes 4 Bytes in the memory. And the number is beyond the capacity of the integer. The maximum array size we can declare is – .
Because the array requires the size in integer, none of the lines will give a compile-time error. The program will compile fine. But we get the runtime exception in line 2. The exception is – NegativeArraySizeException.
Here what will happen is – At the time when JVM will allocate the required memory during runtime then it will find that the size is negative. And the array size cant be negative. So the JVM will throw the exception.
What Is Enumeration In Java
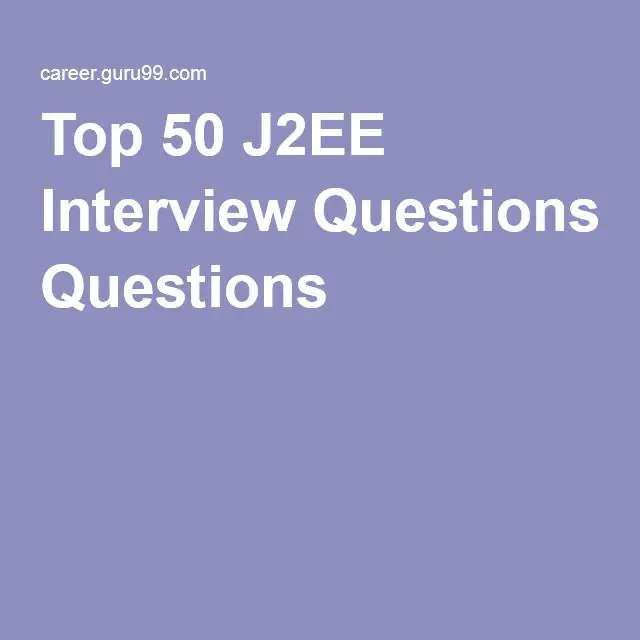
Enumeration means a list of named constants. In Java, enumeration defines a class type. An Enumeration can have constructors, methods, and instance variables. It is created using the enum keyword. Each enumeration constant is public, static, and final by default. Even though enumeration defines a class type and has constructors, you do not instantiate an enum using new. Enumeration variables are used and declared in much the same way as you do a primitive variable.
Don’t Miss: How Can I Watch Harry And Meghan Interview
Q 30 How Many Types Of Constructors Are Used In Java
There are two types of constructors that are used in Java.
Parameterized Constructors: Parameterized constructor accepts the parameters with which users can initialize the instance variables. Users can initialize the class variables dynamically at the time of instantiating the class.
Default constructors: This type doesnt accept any parameters rather, it instantiates the class variables with their default values. It is used mainly for object creation.
When Can You Use The Super Keyword
The use of the super keyword in Java is:-
The super variables are used with variables, methods, and constructors and it is a reference variable that is used to refer to parent class objects and super variables are used with variables, methods, and constructors.
1. The derived class and base class have the same data members if a super keyword is used with variables.
2. A super keyword is used when we want to call the parent class method if a parent and child class have the same-named methods.
3. The super keyword can also be used to access the parent class constructor.
Recommended Reading: Microsoft Data Engineer Interview Questions
Can The Static Methods Be Overridden
- No! Declaration of static methods having the same signature can be done in the subclass but run time polymorphism can not take place in such cases.
- Overriding or dynamic polymorphism occurs during the runtime, but the static methods are loaded and looked up at the compile time statically. Hence, these methods cant be overridden.
What Is The Purpose Of A Default Constructor
The purpose of the default constructor is to assign the default value to the objects. The java compiler creates a default constructor implicitly if there is no constructor in the class.
Output:
0 null0 null
Explanation: In the above class, you are not creating any constructor, so compiler provides you a default constructor. Here 0 and null values are provided by default constructor.
Recommended Reading: What Kind Of Questions Will They Ask In An Interview
Q10 What Is Multiple Inheritance Is It Supported By Java
If a child class inherits the property from multiple classes is known as multiple inheritance. Java does not allow to extend multiple classes.
The problem with multiple inheritance is that if multiple parent classes have the same method name, then at runtime it becomes difficult for the compiler to decide which method to execute from the child class.
Therefore, Java doesnt support multiple inheritance. The problem is commonly referred to as Diamond Problem.
In case you are facing any challenges with these java interview questions, please comment on your problems in the section below.