String Coding Interview Questions
Along with array and linked list data structures, a string is another popular topic on programming job interviews. I have never participated in a coding interview where no string-based question was asked.
A good thing about the string is that if you know the array, you can solve string-based questions easily, because strings are nothing but a character array.
So all the techniques you learn by solving array-based coding questions can be used to solve string programming questions as well.
Here is my list of frequently asked string coding questions from programming job interviews:
Ready for the solutions to the problems?
Here are the solutions to each of these string problems:
Coding Question In C: 91+ Most Asked C Coding Questions
This Page has a massive bunch of most commonly asked and most important tricky coding question in c for freshers to crack the coding interview rounds.
Along with the of pattern programming in C, in coding rounds, interviewers commonly gives you some question to write a program on it.
This Programs may contain some operations to perform on numbers, strings, arrays or on linked list.
Below are the c programs asked in coding round which are categoried section wise. Prepare each variety of coding questions which is very important and will help you to crack your coding rounds.
How To Use Foreach Method
The forEach method provides a shortcut to perform an action on all the elements of an iterable. Letâs say we have to iterate over the list elements and print it.
List< String> list = new ArrayList< > Iterator< String> it = list.iterator while )
We can use forEach method with lambda expression to reduce the code size.
List< String> list = new ArrayList< > list.forEach
Also Check: What Are The Most Questions Asked At A Job Interview
How To Remove Values To A Python Array
Elements can be removed from the python array using pop or remove methods.
pop: This function will return the removed element .
remove:It will not return the removed element.
Consider the below example :
x=arr.arrayprint)print)x.removeprint
Output:
3.61.1 # element popped at 3 rd indexarray
Are you interested in learning Python from experts? Enroll in our online Python Course in Bangalore today!
Top 100 Interview Questions 1 Of 2
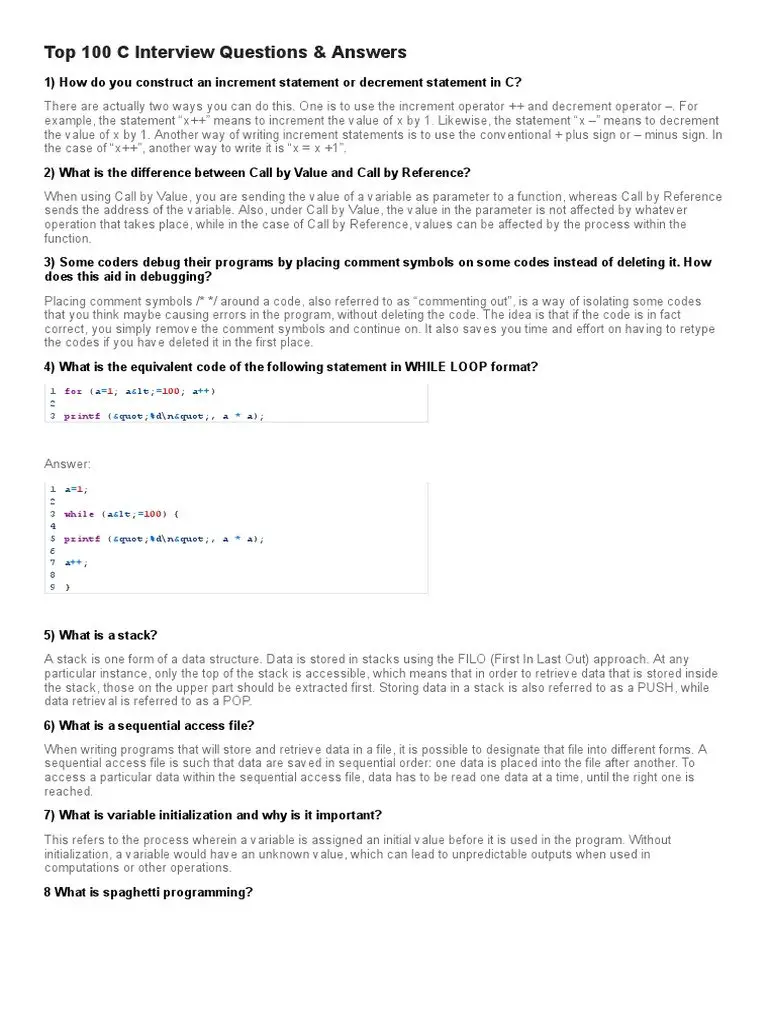
This is a sub-category to find the top 100 LeetCode interview questions asked in FAANG.
One of the most frequently asked software interview questions is the Trapping Rain Water Problem. In this post, Ill go over the solution to the Trapping Rain Water problem, as well as the algorithm you can use to solve it. Question: Calculate how much water can be trapped after raining using n non-negative integers representing Read more
Find Diameter of Binary Tree is the most frequently asked software interview question nowadays. It tests your skill and understanding of the Binary Tree and its height calculation. What is Diameter of the Binary Tree? The number of nodes on the longest path between two end nodes is the diameter of Read more
Are you looking to solve and get the solution of Product of Array Except self? This is a very common interview question in the Software Engineering field by big companies. Question: Given an integer array numberArray, return an array result such that result is equal to the product of all the elements of nums except numberArray . The product of any prefix Read more
You May Like: How To Study For A Job Interview
What Is Function Overloading
Function overloading is a method that allows a developer to define multiple functions that share the same name but have different functionalities. This is achieved by creating the different function versions with different arguments. The compiler then knows which function is needed by the type and number of arguments supplied.
Explain What A Loop Is In A Linked List
If a linked list doesnt have an end, developers label this a loop. Your candidates may mention that developers can use hashing to remove loops from linked lists, which works through traversing linked lists and entering found nodes into a hash set. If developers find that the node is already in the hash set, this means that it has already been seen.
You May Like: What Are Great Interview Questions
Does A Derived Class Inherit Or Doesnt Inherit
When a derived class is constructed from a particular base class, it basically inherits all the features and ordinary members of the base class.But there are some exceptions to this rule. For instance, a derived class does not inherit the base classs constructors and destructors.
Each class has its own constructors and destructors. The derived class also does not inherit the assignment operator of the base class and friends of the class.The reason is that these entities are specific to a particular class and if another class is derived or if it is the friend of that class, then they cannot be passed onto them.
How Would You Implement Linked Lists With Stacks
Your applicants should know that developers can use two stacks to simulate linked lists. They may explain that the first stack is called the list and that developers use the second for temporary storage. Developers can either add, remove, or insert items from the stack after they have completed the following steps:
- Include the header files that the program uses
- Define node pointer and set the pointer to NULL
- Implement main methods by displaying the menu operations list
Also Check: What To Say During A Phone Interview
What Is Self In Python
Self is an object or an instance of a class. This is explicitly included as the first parameter in Python. On the other hand, in Java it is optional. It helps differentiate between the methods and attributes of a class with local variables.
The self variable in the init method refers to the newly created object, while in other methods, it refers to the object whose method was called.
Syntax:
Class A:def func:print
What Are Void Pointers
A void pointer is a pointer which is having no datatype associated with it. It can hold addresses of any type.
For example-
void*ptr char*str p=str // no error str=p // error because of type mismatch
We can assign a pointer of any type to a void pointer but the reverse is not true unless you typecast it as
str= ptr
Read Also: Educative Grokking The Coding Interview Review
Common Java Coding Interview Questions About Sorting
Ask your interviewees some of these 15 interview questions about sorting in Java to assess their knowledge in depth.
How To Create Enum In Java
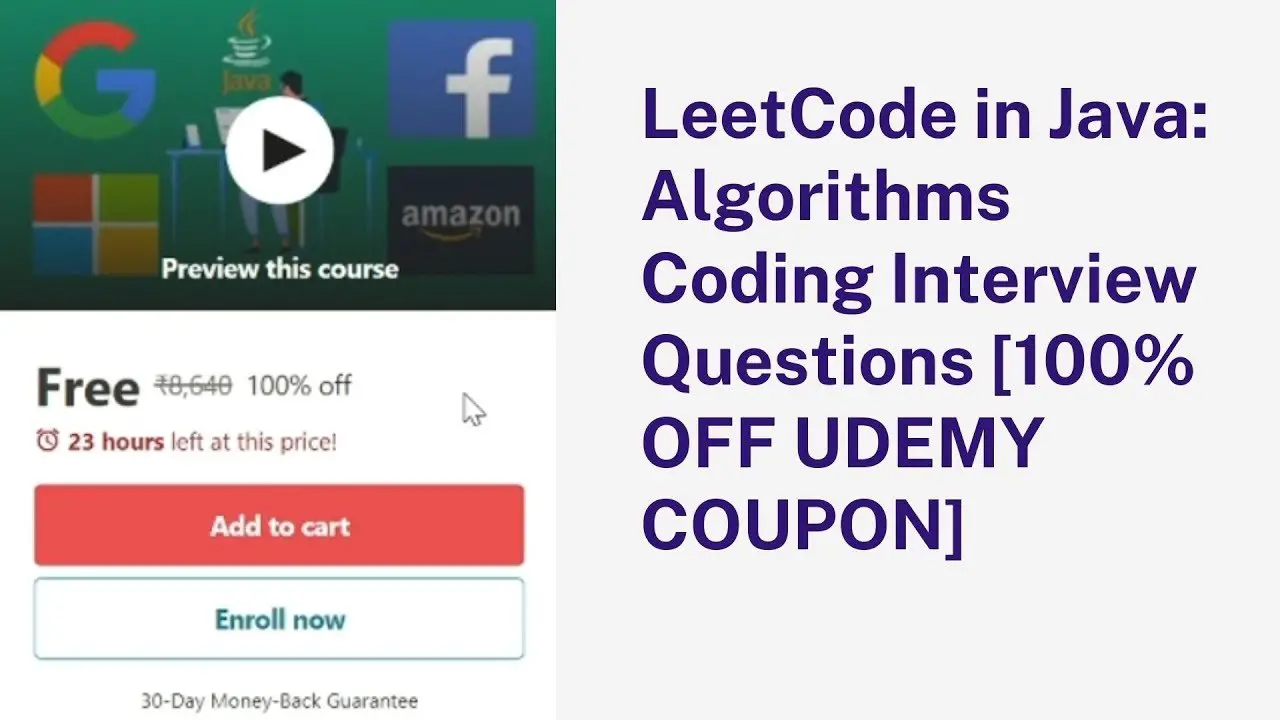
Letâs look at a simple Enum.
public enum ThreadStates
ThreadStates is the enum with fixed constants fields START, RUNNING, WAITING, and DEAD. All Enum implicitly extends java.lang.Enum class and implements Serializable and Comparable interfaces. Enum can have methods also, read more at Enums in Java.
You May Like: How To Conduct A Coding Interview
When There Are A Global Variable And Local Variable With The Same Name How Will You Access The Global Variable
When there are two variables with the same name but different scope, i.e. one is a local variable and the other is a global variable, the compiler will give preference to a local variable. In order to access the global variable, we make use of a scope resolution operator . Using this operator, we can access the value of the global variable.
#include< iostream.h> int x=10 intmain
Output:
Top 100+ Programming Interview Questions And Answers
Question 1. What Is Computer Programming?
Answer :
Computer Programming is also known as programming or coding. Programming is a process which includes procedures which includes coding, preserving, updating, debugging, writing, designing , and so on.
Question 2. How Programming Work?
Answer :
Programming contains a set of instructions for the computer to carry out specific tasks. In fact, those instructions are executable commands, every having a extraordinary reason.
Python Interview QuestionsQuestion three. What Is Debugging?
Answer :
Debugging is the procedure of locating and removing mistakes in a program. In this manner, this system is very well checked for errors. Then mistakes are mentioned and debugged.
Question 4. Name Different Types Errors Which Can Occur During The Execution Of A Program?
Answer :
There are three kinds of errors that can occur at some point of the execution of a application.
Syntax ErrorsQuestion five. When A Syntax Error Occurs?
Answer :
A syntax errors happens while the program violates one or greater grammatical regulations of the programming language. These errors are detected at compile time, i.E., while the translator attempts to translate this system.
Core Java Interview QuestionsQuestion 6. When A Runtime Error Occurs?
Answer :
Question 7. When A Logical Error Occurs?
Answer :
Core Java Tutorial Basic Programming Interview QuestionsQuestion eight. What Is Flowchart?
Answer :
Question 9. What Is An Algorithm?
Answer :
Answer :
Answer :
Don’t Miss: Microservices Design Patterns Interview Questions
Here Are A Few Sample Questions:
Application-based questions:
To master the topic, learn the array concept from the ground up and data structures and basic programming constructors such as loops, recursion, and fundamental operators.
Who This Course Is For:
- Who is interested in crashing the technical interview
- Who is interested in learning the algorithm
- Who is interested in learning data structure
- 889 Students
- 2 Courses
Hi, I am Zhengquan. I am a software engineer from FANG companies. I have worked with two fintech startups before and I witnessed their growth over the year. I switched my career from Finance to Software Engineering because of the excitement of problem-solving.
As a problem solver, I like to make the problem fun and easy to understand. It can help you learn the material with passion.
Not long ago, I have appeared in numerous interviews with top tech companies and some great start-up companies. I believe I can tell you the true feeling of stepping your foot in the door. So, let’s crash the technical interview together!
Also Check: Inventory Control Supervisor Interview Questions
Reverse Words In A Sentence
Reverse the order of words in a given sentence .
To solve this problem, well keep an array of size amount + 1. One additional space is reserved because we also want to store the solution for the 0 amount.
There is only one way you can make a change of 0, i.e., select no coin so well initialize solution = 1. Well solve the problem for each amount, denomination to amount, using coins up to a denomination, den.
The results of different denominations should be stored in the array solution. The solution for amount x using a denomination den will then be:
solution = solution + solution
Well repeat this process for all the denominations, and at the last element of the solution array, we will have the solution.
Enjoying the article? Scroll down to for our free, bi-monthly newsletter.
What Are Dynamic Data Structures
Dynamic data structures have the feature where they expand and contract as a program runs. It provides a very flexible method of data manipulation because adjusts based on the size of the data to be manipulated.
These 20 coding interview questions that test the conceptual understanding of the candidates give the interview a clear idea on how strong the candidates fundamentals are
You May Like: What Are The Interview Questions
Top 100 Coding Problems From Programming Job Interviews
Without wasting any more of your time, here is my list of 100 frequently asked coding problems from programming job interviews. In order to get most of this list, I suggest actually solving the problem.
Do it yourself, no matter whether you are stuck because thats the only way to learn. After solving a couple of problems you will gain confidence.
I also suggest you look at the solution when you are stuck or after you have solved the problem, this way you learn to compare different solutions and how to approach a problem from a different angle.
What Is : : Init: : In Python

Equivalent to constructors in OOP terminology, __init__ is a reserved method in Python classes. The __init__ method is called automatically whenever a new object is initiated. This method allocates memory to the new object as soon as it is created. This method can also be used to initialize variables.
Syntax
:class Human:# init method or constructordef __init__:self.age = age# Sample Methoddef say:printh= Humanh.say
Output:
Hello, my age is 22
Recommended Reading: What To Ask A Cfo In An Interview
Question 3: Largest Sum Contiguous Subarray
Largest sum contiguous subarray is the task of finding the contiguous subarray within a one-dimensional array of numbers which has the largest sum.For example :
3 | forthe sequence of values2,1,3,4,1,2,1,5,4 the contiguous subarray with the largest sum is4,1,2,1,with sum6 |
Solution :
What Is The Difference Between C And C++
Following are the differences between C and C++:
C | |
---|---|
C language was developed by Dennis Ritchie. | C++ language was developed by Bjarne Stroustrup. |
C is a structured programming language. | C++ supports both structural and object-oriented programming language. |
C is a subset of C++. | C++ is a superset of C. |
C does not support the data hiding. Therefore, the data can be used by the outside world. | C++ supports data hiding. Therefore, the data cannot be accessed by the outside world. |
C supports neither function nor operator overloading. | C++ supports both function and operator overloading. |
Reference variables are not supported in C language. | C++ supports the reference variables. |
Read Also: What Are Some Good Responses To Interview Questions
How Does Break Continue And Pass Work
These statements help to change the phase of execution from the normal flow that is why they are termed loop control statements.
Python break: This statement helps terminate the loop or the statement and pass the control to the next statement.
Pythoncontinue: This statement helps force the execution of the next iteration when a specific condition meets, instead of terminating it.
Pythonpass: This statement helps write the code syntactically and wants to skip the execution. It is also considered a null operation as nothing happens when you execute the pass statement.
Linked List Programming Questions
Linked list data structures complement array data structures, which makes it very common for programmers to use. A hiring manager may ask you questions about linked list data structures to determine how much experience you have working with them and how much you know about recursion. Here are some common questions about linked list programming:
-
How would you merge two sorted listed links?
-
What steps would you take to identify the middle element of a singly linked list?
-
What is the difference between a linked list and an array data structure?
-
How do you reverse a singly linked list without recursion?
-
How do you find the start of a loop?
-
How do you remove the Nth Node from the end of a linked list?
-
How do you find a cycle’s starting node?
-
Using Stack, how would you identify the sum of two linked lists?
You May Like: How To Write An Interview Thank You