What’s The Difference Between An Iterable And An Iterator In Python
In Python, the iterator is implemented via two methods: _iter_ and _next_.
An iterable is a container object that contains other objects known as “items”. For example, a list is iterable because lists contain other list items as well as other types of objects like numbers and strings. An iterable will have its _iter_ method defined.
An iterator is a way to iterate over the items in a container, such as a list of integers. Each item in the list can be iterated over one at a time using the iterator function. An iterator will have both_iter_ and _next_ defined, where _iter_ returns the iterator object, and _next_ returns the next element in the iteration.
Iterables are an important concept to master because they’re often used to loop over the contents of a container object.
Reflect: What other iterables can you name?
How To Start Your Interview Prep
I think that for most people starting out in this interview prep process, LeetCode is pretty hard. Here are two possible starting problems, though the best point of entry is admittedly Cracking the Coding Interview.
- FizzBuzz on LeetCode. I recommend it because it should not require any additional interview preparation, and will help you familiarize yourself with the LeetCode platform.
-
Use Git or checkout with SVN using the web URL.
Work fast with our official CLI.Learn more.
If nothing happens, and try again.
Launching GitHub Desktop
If nothing happens, and try again.
Launching Xcode
If nothing happens, and try again.
Launching Visual Studio Code
Your codespace will open once ready.
There was a problem preparing your codespace, please try again.
What Are The Python Coding Interview Questions
Python coding interview questions are asked to test your Python coding expertise and analytical skills. For example, you can expect the questions related to keywords, architecture, ORM, frameworks, how to solve a particular scenario, how to write a code or what is the output of the program, etc. Learn 30+ Python coding interview questions and answers here in this blog.
Recommended Reading: How To Prepare For Data Science Interview
Practice With Mock Interviews
The steps mentioned above can be rehearsed over and over again until you have fully internalized them and they become second nature to you. A good way to practice is by partnering with a friend and taking turns to interview each other.
A great resource for preparing for coding interviews is interviewing.io. This platform provides free and anonymous practice interviews with Google and Facebook engineers, which can lead to real jobs and internships.
Doing well in mock interviews will unlock the jobs page for candidates, and allow them to book interviews with top companies like Uber, Lyft, Quora, Asana, and more. For those who are new to coding interviews, a demo interview can be viewed on this site. Note that this site requires users to sign in.
I have used interviewing.io, both as an interviewer and an interviewee. The experience was great. Aline Lerner, the CEO and co-founder of interviewing.io, and her team are passionate about revolutionizing the process for coding interviews and helping candidates improve their interview skills.
She has also published a number of coding interview-related articles on the interviewing.io blog. I recommend signing up as early as possible with interviewing.io, even though it’s in beta, to increase the likelihood of receiving an invite.
Coding Patterns To Mastery

As I said above, you will also get access to almost 16 amazing patterns that will teach you everything you need to know.
Some of these patterns are
1. Merge Intervals,
11. Pattern: Topological Sort
12. Pattern: Bitwise XOR
Do not worry if all of these sound big and complex right now. You will master all of these by the time you are done. In addition to the help section, there are also snippets of solutions that are provided along with all the challenges. These snippets will make sure that you are not stuck and that you are on your way to expertise.
To be honest with you, these patterns are the single most reason I joined Grokking the Coding Interview: Patterns for Coding Questions course, they are simply invaluable.
You May Like: Data Engineer Interview At Amazon
Grokking The Coding Interview: Patterns For Coding Questions
This course on by Design Gurus expands upon the questions on the recommended practice questions but approaches the practicing from a questions pattern perspective, which is an approach I also agree with for learning and have personally used to get better at coding interviews. The course allows you to practice selected questions in Java, Python, C++, JavaScript and also provides sample solutions in those languages along with step-by-step visualizations. Learn and understand patterns, not memorize answers!Get lifetime access now
Review Of Grokking The Coding Interview Course
Grokking the Coding Interview: Patterns for Coding Questions is an online course developed by Design Gurus. According to its authors, this course has helped thousands of people to prepare for their Coding Interviews. Design Gurus are also famous for their system design course Grokking the System Design Interview Ive reviewed that course too.
In this post, I will present a candid review of one of the most famous resources that many people use to prepare for their coding interview: Grokking the Coding Interview.
Read Also: What To Say In A Thank You Email After Interview
Algorithmic Complexity / Big
- Nothing to implement here, you’re just watching videos and taking notes! Yay!
- There are a lot of videos here. Just watch enough until you understand it. You can always come back and review.
- Don’t worry if you don’t understand all the math behind it.
- You just need to understand how to express the complexity of an algorithm in terms of Big-O.
Grokking The Coding Inteview Overview
This course helped over 35k + students to get a job in FANG companies. Earlier the interviews on coding were not given that much importance, but today, everyone has access to coding questions, so the process becomes more difficult and competitive for the students.
To help the candidate they have come up with the 16 types of coding questions, based on the similarities to solve them. Once the user got the idea he can easily solve more questions.
In this course, each pattern consists of algorithms, data structures, and analysis techniques to solve specific problems. The course has 150 problems mapped to 16 patterns.
Advantages:
- Learning becomes easy when all storage is on clouds, instead of SDK and IDE.
- The progress you can check
- Built-in assessments let you check your progress. Assessments are also there to check your skills.
- Pattern: Fast and Slow pointers
- Pattern: Merge Intervals
- Pattern: In- place Reversal of LinkedList
- Pattern: Tree breadth-first search
- Pattern: 0/1 Knapsack
- Pattern: Topological Sort
Benefits:
My final thoughts:
Beginners can try:
Read Also: Questions For Talent Acquisition Interview
Grokking The Coding Free Interview
Grokking Dynamic Programming Patterns for Coding Interviews Learn Interactively Educative: Interactive Courses for Software Developers 4 7 facebook twitter reddit hacker news link. Coding Interview Patterns Coding Interview Patterns 1. Pattern: Sliding Window. Introduction Maximum Sum Subarray of Size K Smallest Subarray with a given sum E Longest Substring with K Distinct Characters E Fruits into Baskets LeetCode No-repeat Substring LeetCode Longest Substring with Same Letters after Replacement LeetCode.
What Is The Difference Between A Shallow Copy And A Deep Copy
A shallow copy returns a reference to the original object and makes no additional copies. For example, making a shallow copy of a list object creates a new list that contains references to all the items of the source list.
A deep copy copies the items in the list and returns a reference to the newly created copies.
You May Like: How To Prepare A Presentation For An Interview
Grokking Dynamic Programming Patterns For Coding Interviews: Full Course Review
Disclaimer: THIS COURSE IS NOT FOR CODE NEWBIES.
When youre preparing for that coding interview, you need all the help you can get.
Especially when it comes to dynamic programming patterns.
Weve found a dynamic programming course And it contains some of the most common dynamic programming problems and solutions.
But first, lets go what dynamic programming is
This post contains affiliate links. I may receive compensation if you buy something. Read my disclosure for more details.
The Best In Python Learning For Free
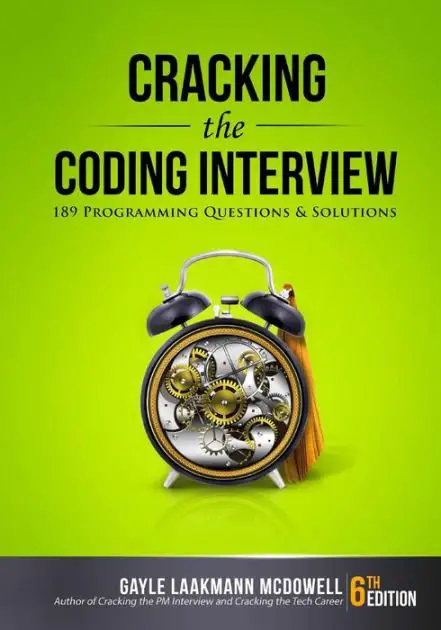
Grokking Python is 100% free and curated by the Educative team: a group of software developers, industry professionals, technical writers, and other assorted geeks dedicated to making developer learning more accessible.
Our content is co-created and vetted by a team that includes over 20 Computer Science PhDs and dozens of Python pros with tons of battle-tested insights to share.
Recommended Reading: How To Get Through An Interview
Grokking The System Design Interview
System Design interviews are arguably some of the most difficult for software engineers.
Making it even more challenging, many college computer science courses and coding bootcamps dont include system design in the curriculum.
But Grokking the System Design Interview bridges that gap.
Its designed to expose you to the most important elements of system design asked in FAANG-level interviews.
What To Do When You Get The Question
Many candidates start coding as soon as they hear the question. That is usually a big mistake. First, take a moment and repeat the question back to the interviewer to make sure that you understand the question. If you misunderstand the question, then the interviewer can clarify.
Always seek clarification about the question upon hearing it, even if you think it is clear. You might discover that you have missed something. It also lets the interviewer know that you are attentive to details.
Consider asking the following questions.
- How big is the size of the input?
- How big is the range of values?
- What kind of values are there? Are there negative numbers? Floating points? Will there be empty inputs?
- Are there duplicates within the input?
- What are some extreme cases of the input?
- How is the input stored? If you are given a dictionary of words, is it a list of strings or a trie?
After you have sufficiently clarified the scope and intention of the problem, explain your high-level approach to the interviewer, even if it is a naive solution. If you are stuck, consider various approaches and explain out loud why it may or may not work. Sometimes your interviewer might drop hints and lead you toward the right path.
Only start coding after you and your interviewer have agreed on an approach and you have been given the green light.
Read Also: Java Crash Course For Interview
What Is The Difference Between == And Is In Python
Equality testing uses the values of objects to determine whether they are equal.
Identity testing uses the memory location of the objects to determine if they are equal.
-
== checks if two values are the same
-
is checks for identity, which checks to see if a string is the same as another string while taking into account whitespaces and other differences
For example, two lists can be equal if they have the same contents, but they aren’t necessarily identical.
How Much Does It Cost
Educative.io offers a few different pricing options for Grokking the Coding Interview.
The first main option is a subscription.
You can choose a monthly, yearly, or two-year service. These subscriptions give you access to all of Educative.ios hundreds of courses.
Subscription prices range from about $15 a month up to $60 per month.
Keep in mind, the annual plans are billed yearly.
Alternatively, Educative.io also sells their courses individually.
If you choose that option, this course costs around $80 for yearly access.
Also Check: How To Watch Oprah’s Interview With Harry And Meghan
But I Already Know Those Answers Why Practice
Because interviews can be stressful.
This can lead to rambling, fumbling, spacing out, and an endless list of other unflattering side effects.
And its that nervous response that may lead to you not getting the jobEven if you do great with the technical stuff.
This post contains affiliate links. I may receive compensation if you buy something. Read my disclosure for more details.
Grokking The Coding Interview: Pros And Cons
There are good reasons why we cant afford to skip the pros and cons of the platform we are reviewing.
One reason is to isolate the key takeaways of the review quickly.
Another and more important reason is that no software is perfect. We must ensure that any software or platform that scales through our decision-making process is ticking enough of the right boxes.
I think the pros should be considered first.
You May Like: Where Can I Watch Meghan And Harry Oprah Interview
Whats The Interview Process Like In A Nutshell
If I were asked to summarize all the resources above to you, heres what I would share:
- I would say that you typically get between 30 minutes to an hour to solve a coding question.
- You typically get a choice of programming language like C++, Python, Java, or JavaScript, but not alwaysin some instances you do not have a choice.
- Outside of the coding portion, they are free to ask you pretty much anything, from language-specific questions to favorite projects, but any interview with a coding portion tends to be dominated by it.
And heres some general advice to consider: you are going to be dealing with people who may be pretty tired of interviews. Smile if you can. Try to show enthusiasm. Have a conversation, and try to talk to the interviewer the way you might communicate if the two of you were pair programming, but they were more senior.
Who This Course Is For:
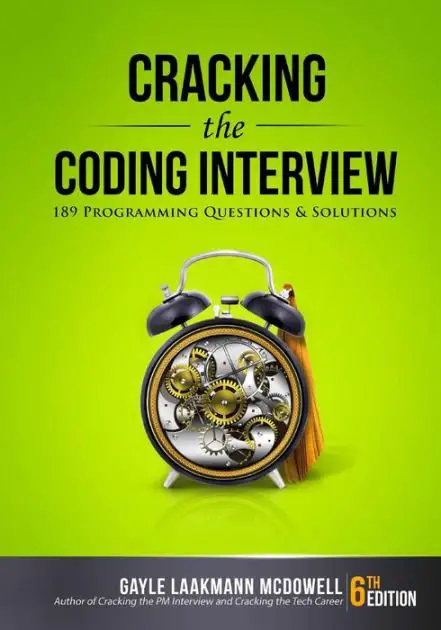
- Computer science students, Software professionals who wants to learn and improve their ability of problem solving in coding interview.
- 16,052 Students
Energetic software engineer with 10+ years experience developing robust code for high-volume businesses. Highly familiar with a wide variety of Software Engineering, Database, Web as well as Data Science. Progressively engage my experience through my passion for creating application using best practice intuitive experience as focus. Using my diverse skills set in order to provide a super service that will assist a range of clients. Possess a Masters Degree in Computer Science. I have developed several web and desktop apps for big tech company such as: Microsoft, Boeing, Bluetooth
You May Like: What Are Good Questions To Ask At A Job Interview
Recommended Reading: Why Do You Want To Work In Healthcare Interview Answer
Grokking Coding Patterns On Educativeio
Hi, did anybody here used “Grokking the Coding Interview: Patterns for Coding Questions”?
I want to know how the course is? and if it’s worth taking and anybody willing to sell it on a discount I am ready to buy.
eh, I did it. tbh it wasn’t worth it in my opinion. Its just the same interview questions that you find on leetcode, separated by techniques. Some of them that say medium or hard are now leetcode easy’s and mediums respectively when you search them up. You’re better off using leetcode and the discussions page imo than pay 80 bucks for it.
Also here is an article of all the techniques/patterns they go over and by go over I mean a short paragraph on what the technique is then just questions and solutions using said technique.
Im actually going to disagree with this opinion. I found myself lacking some unknown basics when doing leetcode and having a hard time picking up the patterns. I’m just a bit too old / ADHD to be able to learn efficiently through videos . I really appreciate the text format of this course and have found it to be exactly what I needed so far.
What Is A Lambda Function In Python
A lambda function works like any other Python function but anonymously and is contained in a single line of code. Lambda functions can accept multiple parameters but can only accept one expression.
Syntax of a lambda function:
lambda parameter: expression
The function evaluates the expression and returns its results.
Example of a lambda function with a single parameter:
x = lambda y : y + 4
The function takes a single parameter y and returns y+4.
Example of a lambda function with multiple parameters:
x = lambda y, z : y * z
Here, the function takes two parameters y and z. It returns their product.
Recommended Reading: How To Answer System Design Interview Questions