What Are The Static Members And Static Member Functions
When a variable in a class is declared static, space for it is allocated for the lifetime of the program. No matter how many objects of that class have been created, there is only one copy of the static member. So same static member can be accessed by all the objects of that class.
A static member function can be called even if no objects of the class exist and the static function are accessed using only the class name and the scope resolution operator ::
What Is The Difference Between C And C++
The main difference between C and C++ are provided in the table below:
C | |
---|---|
C is a procedure-oriented programming language. | C++ is an object-oriented programming language. |
C does not support data hiding. | Data is hidden by encapsulation to ensure that data structures and operators are used as intended. |
C is a subset of C++ | C++ is a superset of C. |
Function and operator overloading are not supported in C | Function and operator overloading is supported in C++ |
Namespace features are not present in C | Namespace is used by C++, which avoids name collisions. |
Functions can not be defined inside structures. | Functions can be defined inside structures. |
new operator is used for memory allocation and deletes operator is used for memory deallocation. |
What Are The Common Causes Of Segmentation Fault In C
View answer
- Tried to write read-only memory .
- Trying to access a nonexistent memory address .
- Trying to access memory the program does not have rights to .
- Sometimes dereferencing or assigning to an uninitialized pointer can be the cause of the segmentation fault.
- Dereferencing the freed memory can also be caused by the segmentation fault.
- A stack overflow is also caused by the segmentation fault.
- A buffer overflow is also a cause of the segmentation fault.
Don’t Miss: How To Prepare For Financial Analyst Interview
Q44 #define Cat X##y Concatenates X To Y But Cat3 Does Not Expand But Gives Preprocessor Warning Why
Ans:In this case, the cat is the macro which is defined by using the preprocessor directive, this will be substituted only at the place where it is called in this example it happens like this
cat##3 which will once again become 1##2##3
here if we use ## in between we can join or concatenate only two variables that why it gives a preprocessor warning.
What Is Dangling Pointer In C
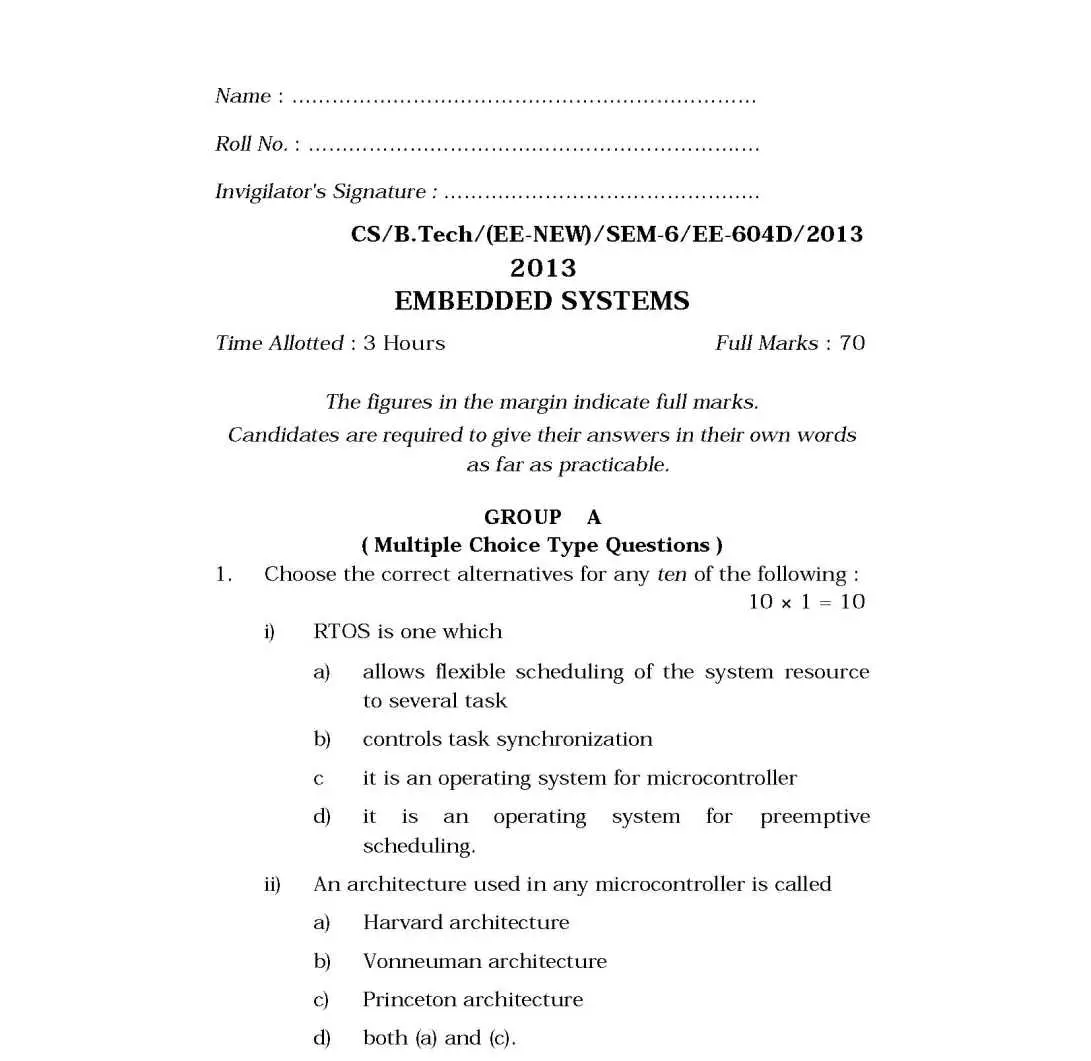
- If a pointer is pointing any memory location, but meanwhile another pointer deletes the memory occupied by the first pointer while the first pointer still points to that memory location, the first pointer will be known as a dangling pointer. This problem is known as a dangling pointer problem.
- Dangling pointer arises when an object is deleted without modifying the value of the pointer. The pointer points to the deallocated memory.
Let’s see this through an example.
In the above example, initially memory is allocated to the pointer variable ptr, and then the memory is deallocated from the pointer variable. Now, pointer variable, i.e., ptr becomes a dangling pointer.
How to overcome the problem of a dangling pointer
The problem of a dangling pointer can be overcome by assigning a NULL value to the dangling pointer. Let’s understand this through an example:
In the above example, after deallocating the memory from a pointer variable, ptr is assigned to a NULL value. This means that ptr does not point to any memory location. Therefore, it is no longer a dangling pointer.
You May Like: What Questions Can You Not Ask In An Interview
What Are The Main Advantages Of Embedded C
Embedded C is the extension of the C programming language. Let’s see the key advantages of Embedded C:
Key advantages of Embedded C:
- The coding speed of Embedded C is high, and it is simple and easy to understand.
- It doesn’t require any hardware changes such as extra memory or space for storage as it performs the same task all the time.
- It is dedicated to its specific task and performs only one task at one time.
- It is mainly used in modern automatic applications. Embedded applications are very suitable for industrial purposes.
What Are Class And Object In C++
A class is a user-defined data type that has data members and member functions. Data members are the data variables and member functions are the functions that are used to perform operations on these variables.
An object is an instance of a class. Since a class is a user-defined data type so an object can also be called a variable of that data type.
A class is defined as-
classA}
For example, the following is a class car that can have properties like name, color, etc. and they can have methods like speed.
You May Like: Python Data Structure Interview Questions
Microcontroller Embedded Software Engineer Interview Questions
Microcontrollers are an important topic that you can expect questions on at your embedded software engineer interview. Take a look at the sample questions below:
What Are The Reasons For Interrupt Latency And How To Reduce It
Following are the various causes of Interrupt Latency:
- Hardware: Whenever an interrupt occurs, the signal has to be synchronized with the CPU clock cycles. Depending on the hardware of the processor and the logic of synchronization, it can take up to 3 CPU cycles before the interrupt signal has reached the processor for processing.
- Pipeline: Most of the modern CPUs have instructions pipelined. Execution happens when the instruction has reached the last stage of the pipeline. Once the execution of an instruction is done, it would require some extra CPU cycles to refill the pipeline with instructions. This contributes to the latency.
Interrupt latency can be reduced by ensuring that the ISR routines are short. When a lower priority interrupt gets triggered while a higher priority interrupt is getting executed, then the lower priority interrupt would get delayed resulting in increased latency. In such cases, having smaller ISR routines for lower priority interrupts would help to reduce the delay.
Also, better scheduling and synchronization algorithms in the processor CPU would help minimize the ISR latency.
Don’t Miss: Swift Interview Questions For Senior Developer
What Is The Result Of The Below Code
void demo else }
In Embedded C, we need to know a fact that when expressions are having signed and unsigned operand types, then every operand will be promoted to an unsigned type. Herem the -40 will be promoted to unsigned type thereby making it a very large value when compared to 10. Hence, we will get the statement Greater than 10 printed on the console.
How Can We Improve The 8051 Microcontroller Performance
The simplest way to improve the performance of the 8051 microcontrollers is to increase the clock frequency. The 8051 microcontrollers allow the use of clock speeds well beyond the 12MHz limit of the original devices. The best way to improve the performance is to make internal changes to the microcontroller so that fewer oscillator cycles are required to execute each machine instruction.
Read Also: Interview Questions For Power Bi Developer
What Are The C++ Access Specifiers
In C++ there are the following access specifiers:
Public: All data members and member functions are accessible outside the class.
Protected: All data members and member functions are accessible inside the class and to the derived class.
Private: All data members and member functions are not accessible outside the class.
What Do You Understand By A Null Pointer In Embedded C
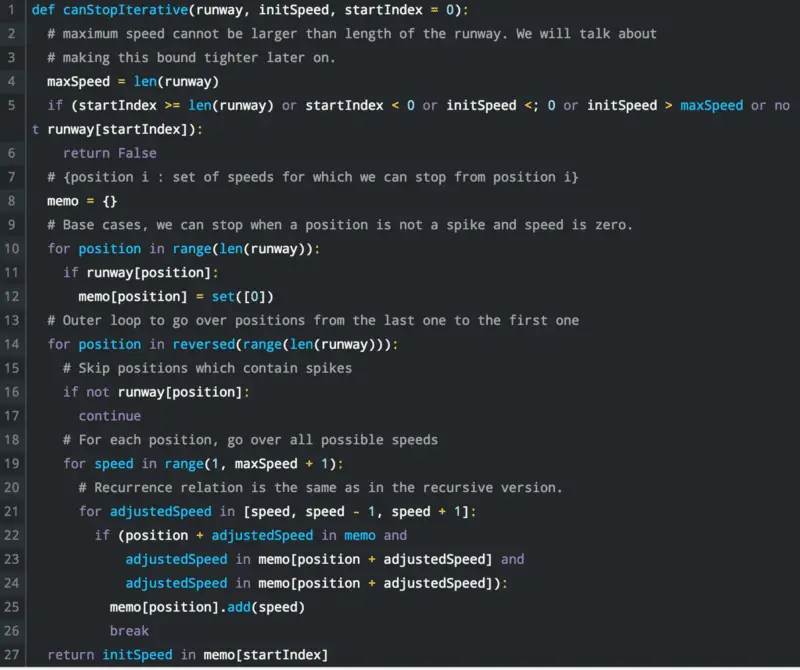
A null pointer is a pointer that does not point to any valid memory location. It is defined to ensure that the pointer should not be used to modify anything as it is invalid. If no address is assigned to the pointer, it is set to NULL.
Syntax:
data_type *pointer_name = NULL
One of the uses of the null pointer is that once the memory allocated to a pointer is freed up, we will be using NULL to assign to the pointer so that it does not point to any garbage locations.
Read Also: Software Engineer Interview Cheat Sheet
Q26 Differentiate Between Getch And Getche
Ans: Both the functions are designed to read characters from the keyboard and the only difference is that
getch: reads characters from the keyboard but it does not use any buffers. Hence, data is not displayed on the screen.
getche: reads characters from the keyboard and it uses a buffer. Hence, data is displayed on the screen.
//Example
#include& amp lt stdio.h& amp gt #include& amp lt conio.h& amp gt int main
//Output
Your entered character is xPlease enter another character zYour new character is z
Bit Manipulation Embedded Software Engineer Interview Questions
In your embedded software engineer interview, you can expect questions on bit manipulation. Here are some sample questions:
Q1. Return the integer in the range missing from the given nums array containing n distinct numbers in the range .
Example 1:
Input: nums =
Output: 2
Explanation: n = 3 because there are 3 numbers, so all numbers are in the range . 2 is the missing number in the range as it does not appear in the array.
Example 2:
Input: nums =
Output: 2
Explanation: n = 2 because there are 2 numbers, so all numbers are in the range . 2 is the missing number in the range as it does not appear in the array.
Solution:
We are given distinct numbers with only one missing number in the range 1 to n .
So we can write, sum of numbers in given array + missing number = = ) / 2. Hence, the missing number = ) / 2 – sum of numbers in the given array.
Time complexity: O, as we are traversing along the array.
int missingNumber(vector & nums) return Nsum - sumOfnums }
Also Check: Interview Questions About The American Dream
What Do You Understand By Machine Code
Machine Code is the language of the computer rather than the programmer. A computer only accepts the instructions in the form of machine code or object code. When a programmer interprets the code, it is complex and error-prone. Whether in languages such as C, C++, and Java, all software must ultimately be translated into machine code to be executed by the computer.
What Is Machine Code
Computers only accept instructions in machine code or object code. It is the language of the computer RATHER than the Programmer. The interpretation of the code by the programmer is complex and error-prone. Whether in assembly, C, C++, Java, or Ada, all software must ultimately be translated into machine code to be executed by the computer.
Also Check: Front End Interview Questions With Answers
Q32 How Does Taking The Address Of Local Variables To Result In Unoptimized Code
Ans:The most powerful optimization for the compiler is register allocation. That is it operates the variable from the register, then a memory.
Generally, local variables are allocated in registers. However, if we take the address of a local variable, the compiler will not allocate the variable to register.
+ Embedded C Interview Questions And Answers
Q1. Can A Variable Be Both Const And Volatile?
The const keyword make sure that the value of the variable declared as const cant be changed. This statement holds true in the scope of the program. The value can still be changed by outside intervention. So, the use of const with volatile keyword makes perfect sense.
Q2. Advantages And Disadvantages Of Using Macro And Inline Functions?
The advantage of the macro and inline function is that the overhead for argument passing and stuff is reduced as the function are in-lined. The advantage of macro function is that we can write type insensitive functions. It is also the disadvantage of macro function as macro functions cant do validation check. The macro and inline function also increases the size of the executable.
Q3. What Is Null Pointer And What Is Its Use?
The NULL is a macro defined in C. Null pointer actually me a pointer that does not point to any valid location. We define a pointer to be null when we want to make sure that the pointer does not point to any valid location and not to use that pointer to change anything. If we dont use null pointer, then we cant verify whether this pointer points to any valid location or not.
Q4. Can Structures Be Passed To The Functions By Value?
Q5. Why Cannot Arrays Be Passed By Values To Functions?
In C, the array name itself represents the address of the first element. So, even if we pass the array name as argument, it will be passed as reference and not its address.
int *p
main
Read Also: Apple Machine Learning Engineer Interview
What Is The Dma Address And How To Deal With It
A: Direct memory access speeds up memory operations by permitting an input or output device to convey or receive data directly to or from the main memory, bypassing the CPU.
DMA addresses have physical addresses. During data transfer, it is a device that straight drives the data and address bus. As a result, its only a physical address.
When Would An Embedded System Require An Infinite Loop
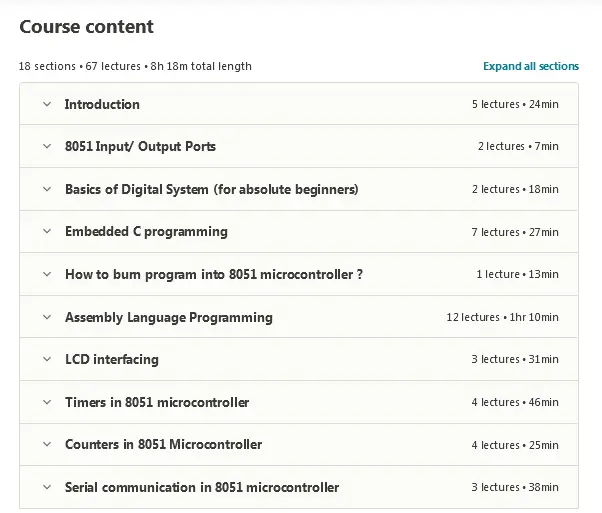
Answering this question can be an opportunity for you to showcase your in-depth knowledge. Interviewers may ask this question to know about your understanding of embedded systems. You can outline the situation when an embedded system needs an infinite loop and explain how you deal with it.
Example:Embedded systems may need an infinite loop in case their job is unfinished. Somehow if the software stops working, the hardware can be rendered useless. In order to make sure the embedded system works permanently, we can surround functional parts of the embedded system with infinite loops. By saying working for forever, I mean working for weeks, months or years based on necessity.
Read more:15 Software Developer Skills And How To Develop Them
You May Like: How To Interview Someone For A Podcast
Embedded System Interview Questions
The Indeed Editorial Team comprises a diverse and talented team of writers, researchers and subject matter experts equipped with Indeed’s data and insights to deliver useful tips to help guide your career journey.
Embedded systems are computer systems that perform certain tasks for larger devices, like robotic vacuum cleaners, self-checkout kiosks and wireless parking meters. For software engineers seeking a job building these kinds of devices, an interview provides an opportunity to show their knowledge of how embedded systems work. By reviewing embedded system interview questions and sample answers, you can prepare for your interview more effectively.
In this article, we share 15 embedded system interview questions with sample answers and provide a list of interview tips for software developers.
Q38 What Is Preprocessor
Ans:A preprocessor is a Program That processes its input data to produce output that is used as input to another program. The output is said to be a preprocessed form of the input data, which is often used by some subsequent programs like compilers. The amount and kind of processing done depend on the nature of the preprocessor.
Some Pre-processors Are Only Capable Of Performing Relatively Simple Textual Substitutions And Macro Expansions, while others have the power of full-fledged programming languages.
A Common Example From Computer programming is the processing performed on source code before the next step of compilation. In some computer languages there is a phase of translation known as preprocessing. It can also include macro processing, file inclusion, and language extensions.
Don’t Miss: What Questions Should You Ask In An Interview
List Out Some Of The Commonly Found Errors In Embedded Systems
Some of the commonly found errors in embedded systems are
- Damage of memory devices static discharges and transient current
- Address line malfunctioning due to a short in circuit
- Data lines malfunctioning
- Due to garbage or errors some memory locations being inaccessible in storage
- Inappropriate insertion of memory devices into the memory slots
- Wrong control signals
Best Questions To Ask Embedded Systems Programmers
September 29, 2014 By Aimee Kalnoskas
Trying to decide if your potential new embedded programmer hire has what it takes? Need a little help with embedded-specific interview questions? Or do you simply want to take a break and have fun with a test?
Electro-Tech-Online member known as amol. guilhane07 has compiled 16 questions to ask when recruiting embedded systems programmers. We list the questions here but we arent giving you the answers right away. Try the test yourself and then follow the link at the end to Amols pdf with questions and answers. Let us know how you did and if you found the questions useful.
Tip: Click the code for a clearer image in a new window.
An obligatory and significant part of the recruitment process for embedded systems programmers seems to be the C Test. Over the years, I have had to both take and prepare such tests and in doing so have realized that these test can be very informative for both the interviewer and interviewee. Furthermore, when given outside the pressure of an interview situation, they can also be quite entertaining .
With these ideas in mind, I have attempted to construct a test that is heavily slanted towards the requirements of embedded systems. This is a lousy test to give to someone seeking a job writing compilers! The questions are almost all drawn from situations I have encountered over the years. Some of them are very tough, however, they should all be informed.
Preprocessor
Infinite Loops
Data Declarations:
Static
Recommended Reading: What Is Ux Design Interview Questions
What Do You Understand By A Function Pointer
A function pointer is a pointer that points to a function instead of a variable. That’s why a function pointer is completely different from the class of other pointers. A function pointer stores the address of a particular function so that the concerned program can avail of it through function invoking.