What Is The Use Of Middleware Redux Thunk
Redux thunk is a middleware which sits between action creator and reducer in the React-Redux flow. It is very useful when we do asynchronous API calls with fetch or axios, which returns a Promise and we then dispatch it to reducer.
Redux thunk mainly works behind the scene. We have used some boiler-plate to use it.
//App.jsimport React from 'react' import ReactDOM from 'react-dom' import Routes from './routes' import store from './store' import from 'react-redux' ReactDOM.render) //store.jsimport from 'redux' import thunk from 'redux-thunk' import rootReducer from '../reducer' const store = createStore) export default store
In Question 22 for React-Redux flow, in action creator, we call an API endpoint with axios. It is a network request and will take some milliseconds or more depending on the connection.
Now, thunk middleware sort of wait for that call, which is a Promise to completed. Once we get the response, which contains the data then only it dispatches it to the reducer.
export const getIndPopData = => dispatch => /India/`).then }) }
So, Redux thunk is required in Projects where we do API calls to some endpoint.
How Are Actions Defined In Redux
Actions in React must have a type property that indicates the type of ACTION being performed. They must be defined as a String constant and you can add more properties to it as well. In Redux, actions are created using the functions called Action Creators. Below is an example of Action and Action Creator:
function addTodo }
What Is Purecomponent In React
Based on the concept of purity in function programming paradigms, a function is said to be pure if it meets the following two conditions:
- Its return values are only determined by its input values.
- Its return values are always the same for the same input values.
React Component is considered pure, it renders the same output for the same state and props.
Read Also: How To Write A Post Interview Follow Up Email
Explain The Useeffect Hook
The useEffect Hook allows you to perform side effects in your components like data fetching, direct DOM updates, timers like setTimeout, and much more.
This hook accepts two arguments: the callback and the dependencies, which allow you to control when the side effect is executed.
Note: The second argument is optional.
Example:
import React, from 'react' const App = => , 2000) }, ) return}export default App
Important Knowledge Areas Of Reactjs And Software Development Where You Should Focus On

We talked about the skillsets and interview questions. While these give you a guideline about the specifics that you should look for during the interview, there are general aspects to consider too. These carry plenty of importance since you want effective developers.
We recommend that you look for the following general skills of ReactJS:
Read Also: Where Can I Watch The Prince Harry Interview
Explain Virtual Dom In React
Sites built with vanilla JavaScript directly updates the Real DOM. It is fine for smaller websites, but as your website grows complex, it slows down the website. This happens because the browser engine has to traverse all nodes even if you update only one node.
Even Angular updates or work on Real DOM. ReactJS doesnt update the Real DOM directly but has a concept of Virtual DOM. This causes a great performance benefit for ReactJS and so its faster than vanilla JavaScript apps and also Angular apps.
- Virtual DOM is actually an in-memory representation of Real DOM. It is a lightweight JavaScript Object which is a copy of Real DOM.
- Whenever setState method is called in your code, ReactJS creates the whole Virtual DOM from scratch.
- At a given time, ReactJS maintains two virtual DOM, one with the updated state Virtual DOM and the other with the previous state Virtual DOM.
- ReactJS uses a differential algorithm to compare both of the Virtual DOM to find the minimum number of steps to update the Real DOM.
After that, it updates the Real DOM, whenever its best to update it.
In Which Lifecycle Event Do You Make Ajax Requests And Why
AJAX solicitations ought to go in the componentDidMount lifecycle event. There are a couple of reasons behind this,
Fiber, the following usage of React’s reconciliation algorithm, will be able to begin and quit rendering as required for execution benefits. One of the exchange offs of this is componentWillMount, the other lifecycle event where it may bode well to influence an AJAX to ask for will be “non-deterministic”. This means React may begin calling componentWillMount at different circumstances at whenever point it senses that it needs to. This would clearly be a bad formula for AJAX requests.
You can’t ensure the AJAX request won’t resolve before the component mounts. In the event that it did, that would imply that you’d be attempting to setState on an unmounted component, which won’t work, as well as React will holler at you for. Doing AJAX in componentDidMount will ensure that there’s a component to update.
Read Also: Interview Questions For Cna Position In Hospital
What Do You Understand By Refs In React
Refs is the shorthand used for references in React. It is an attribute which helps to store a reference to particular DOM nodes or React elements. It provides a way to access React DOM nodes or React elements and how to interact with it. It is used when we want to change the value of a child component, without making the use of props.
For More Information, .
What Are Some Lifecycle Methods Of React Components
- render the most commonly used lifecycle method and the only one required in a class component.
- constructor a method called before mounting a component. This method is not necessary if you didnt initialize state yet or bind methods.
- componentDidMount the first method called after a component is mounted.
- componentDidUpdate this method is implemented once a component is updated, though not at initial rendering.
- componentWillUnmount to be implemented before you umount a component is unmounted and also used to perform due cleanups.
Those are the common lifecycle methods. Some are rarer, including shouldComponentUpdate, static getDerivedStateFromProps, getSnapshotBeforeUpdate, static getDerivedStateFromError, and so on. This question may also easily fall under ReactJS experienced interview questions for senior developers if you need to establish their understanding of components.
Don’t Miss: What To Ask When Interviewing Someone
How To Use A Library Like Jquery In React Which Interacts Directly With Dom
Using jQuery in React is not recommended as it directly interacts with the DOM and is generally a bad practice. We should always try first to find a React equivalent of the jQuery plugin or write the plugin ourselves. But many times there are plugins, which are only available in jQuery and no other alternative in ReactJS and also very time consuming to write ourselves. In such cases, we can use the method described below.
We attach a ref element to the root DOM element. Inside componentDidMount, we will get a reference to it, so that we can pass it to the jQuery plugin.
To prevent React from interacting with the DOM after mounting, we will return an empty < div /> from our render method. The < div /> element has nothing, so React will not update it, leaving the jQuery plugin to manage only that part of the DOM. Also, we will use componetWillUnmount to unmount the jQuery plugin, after its work with DOM is done.
class anotherJqueryPlugin extends React.Component componentWillUnmount render /> }}
Refs are generally avoided in React and should be used with precautions. It is because they directly interact with the Real DOM and in React that is not desirable behaviour.
We can, however, use refs in the following situations.
- Interaction with third party libraries like jQuery.
- Doing animations
- Managing focus when we move to a text box
There are many ways to use refs but we will see the way introduced in React 16.3 and that is by using React.createRef
How Would You Create A Form In React
React forms are identical to HTML forms. However, the state is contained in the state property of the component in React and is updateable only via the setState method.
Therefore, the elements in a React form cant directly update their state. Their submission is handled by a JS function, which has full access to the data entered into the form by a user.
The following code demonstrates creating a form in React:
handleSubmit render
16. What are the advantages of using Redux?
Also Check: What Is A Video Interview
Reactjs Interview Questions: Miscellaneous
Some miscellaneous questions about the ReactJS development process, application, properties, functionality, and other associated aspects are asked in an interview. Below is the listed description of some such questions that will help you impressively explain them. So, letâs have a look:
Question 1: What is meant by a higher-order component in ReactJS?
The high-order component is the advanced technology to reuse the component’s logic with specific patterns. They are not a part of React API and provide a new component taking the old one.
Question 2: How does the React Hook differ from classes?
Hooks do not work inside the class but provide a platform to use the classes. Hooks are the functions that connect the Reactstate with the lifestyle features from the functional components.
Question 3: How to design the automated redirect functionality post-login in ReactJS?
The automated redirects functionality post-login in React JS functions by grouping the bunch of hooks rather than following the same redirection route.
Question 4: Explain redux flow structure?
Redux follows a unidirectional data flow with one-way binding data. When the user interacts with the application, an action is dispatched in return. Here in this state, the root reducer function appears with the dispatched action.
Using A Function Component
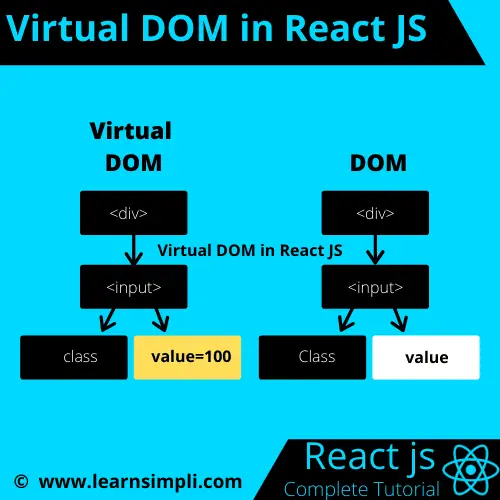
The useEffect hook can be used with function components:
const Homepage = => , ) return < div> Homepage< /div> }
The useEffect hook is more flexible than the lifecycle methods used for class components. It receives two parameters:
The value passed as the second argument controls when the callback is executed:
- If the second parameter is undefined, the callback is executed every time that the component is rendered.
- If the second parameter contains an array of variables, then the callback will be executed as part of the first render cycle and will be executed again each time an item in the array is modified.
- If the second parameter contains an empty array, the callback will be executed only once as part of the first render cycle. The example above shows how passing an empty array can result in similar behaviour to the componentDidMount hook within a function component.
What are the most common approaches for styling a React application?
Also Check: How To Make A Teacher Portfolio For Interviews
Why Can’t Browsers Read Jsx
JSX is not a valid JavaScript code, and there is no built-in implementation that allows the browser to read and understand it. We need to transpile the code from JSX into valid JavaScript code that the browser can understand, and we use Babel, a JavaScript compiler/transpiler, to accomplish this.
Note: create-react-app uses Babel internally for the JSX to JavaScript conversion, but you can also set up your own babel configuration using Webpack.
You can read more about JSX in React in this article.
What Are The Possible Return Types Of Render Method
Below are the list of following types used and return from render method,
Read Also: How To Prepare For Java Interview For 5 Years Experience
What Is The Difference Between Setstate And Replacestate Methods
When you use setState the current and previous states are merged. replaceState throws out the current state, and replaces it with only what you provide. Usually setState is used unless you really need to remove all previous keys for some reason. You can also set state to false/null in setState instead of using replaceState.
What Is The Life Cycle Of A Reactjs Class Component
Each component in react goes through the life cycle of React which keeps monitoring and updating the component. The lifecycle of the component is divided into four phases.
constructor:-
- The constructor for react component is used before it is mounted.
- In class component, while implementing the constructor for a React. component subclass, always you should use super before any other statement.
- Otherwise, this.props will be undefined in the constructor.
getDerivedStateFromProps:- getDerivedStateFromProps the method is called right before rendering the element in the DOM.
render:- The render a method is the only required method in a class component. render should always be pure, which means it does not modify the component state, it returns the same result every time it gets called.
componentDidMount:- componentDidMount gets invoked immediately after the component gets inserted .
2. Updating:- In this phase when the component gets rendered whenever there is a change in the components state or properties.
React has five built-in methods that get called, in this order, when a component is updated:
getDerivedStateFromProps:- getDerivedStateFromProps are invoked right before invoking the render method, both on the initial mount and on subsequent updates.
Recommended Reading: Exit Interview Questions For Executives
Choose One Component Lifecycle Method That Youve Used On A Project How Did You Use It
Tip: If youve worked with React, you know that a react components lifecycle includes its birth , its growth , and its death .
Meanwhile, component lifecycle methods are functions that a component can execute during specific lifestyle phases. The best way to answer this question is to think back to a specific example from a project youve worked on, whether for a job or a student project.
- Example: When building a chat application, we used the componentDidMount lifecycle method to fetch data from a server. Then fill in specifics and details as needed.
Simple as that!
How To Use React Label Element
If you try to render a < label> element bound to a text input using the standard for attribute, then it produces HTML missing that attribute and prints a warning to the console.
< labelfor=> < /label> < inputtype=id=/>
Since for is a reserved keyword in JavaScript, use htmlFor instead.
< labelhtmlFor=> < /label> < inputtype=id=/>
Don’t Miss: How To Prepare For A Manager Position Interview
How To Prevent Unnecessary Updates Using Setstate
You can compare the current value of the state with an existing state value and decide whether to rerender the page or not. If the values are the same then you need to return null to stop re-rendering otherwise return the latest state value.
For example, the user profile information is conditionally rendered as follows,
getUserProfile=user=> else }}) }
Main Part: Tech And Soft Skills Check

The biggest part is checking tech skills. During the preparation, we create a list of questions, and this helps us. We already know what to ask. Very often, I combine this conversation with some practical tasks. We can discuss some topics and then I propose a small coding task to check the discussed areas from a practical side.
Sometimes I create a conversation around the coding challenge. For some candidates, such a format is easier than theoretical discussion. Of course, very often you need to update the discussion scenario on the fly as, during the conversation, you understand that its needed to discuss something else or the same thing but more deeply.
Recommended Reading: How To Study For Case Interviews
How To Pass Data Between Sibling Components Using React Router
We can pass data between React sibling components using React Router using history.push and match.params.
Let look into the code. We have a Parent component App.js. We have two Child Components HomePage and AboutPage. Everything is inside a Router from React-router Route. We also have a route for /about/. This is where we will pass the data.
import React, from react class App extends Component }> Home< /NavLink> < /li> < li> < NavLink to="/about" activeStyle=}> About < /NavLink> < /li> < /ul> < Route path="/about/:aboutId" component= /> < Route path="/about" component= /> < Route path="/" component= /> < /div> < /Router> ) }}export default App
The HomePage is a simple functional component, which have a button. On clicking the button we are using props.history.push , which is used to programatically navigate to /about/data
export default function HomePage return }
The AboutPage is also a simple functional component, which gets the passed data by props.match.params.aboutId
export default function AboutPage return }
The Page after clicking on the button in the HomePage looks like below.
How To Prevent A Function From Being Called Multiple Times
If you use an event handler such as onClick or onScroll and want to prevent the callback from being fired too quickly, then you can limit the rate at which callback is executed. This can be achieved in the below possible ways,
Read Also: Nlp Data Scientist Interview Questions
Frequently Asked Questions About Reactjs
Love to compete?
Join Topcoder Challenges
React is an open-source JavaScript-based library created by Facebook for making UIs. Since its release in 2013, Reacts popularity has been rapidly increasing, it has even been adopted by some big companies like Instagram, Whatsapp, and many more. Despite being relatively new, React is the most popular front-end library today.
What Are The Differences Between Redux
Both Redux Thunk and Redux Saga take care of dealing with side effects. In most of the scenarios, Thunk uses Promises to deal with them, whereas Saga uses Generators. Thunk is simple to use and Promises are familiar to many developers, Sagas/Generators are more powerful but you will need to learn them. But both middleware can coexist, so you can start with Thunks and introduce Sagas when/if you need them.
You May Like: How To Practice For Coding Interviews