Q39 What Are Exports & Imports
Imports and exports help us to write modular JavaScript code. Using Imports and exports we can split our code into multiple files. For example-
//------ lib.js ------< /span> export const sqrt = Math.sqrt < /span> export function square export function diag //------ main.js ------< /span> from 'lib' console.log) // 25console.log) // 5
Now with this, we have reached the final section of JS Interview Questions.
What Is A Javascript Promise What Are The Benefits Of Using A Promise Over A Callback
A JavaScript Promise is a way to write more asynchronous code. A Promise is defined with two functions: a resolve and a reject function. If a Promise is executed successfully, the resolve function is returned to the main program otherwise, the reject function is returned.
Promises allow you to avoid callback hell. This is where you define callback functions within callback functions. Callback hell results in unreadable code. Promises, unlike callbacks, support .then statement. This means that the order in which a block of code runs can be made clearer if you use a Promise.
How Can You Check If An Array Is Empty
To check if an array is empty, you use an if statement:
if
First, the if statement makes sure that an array has been defined. Without this check, our code would return an error if “array_name” could not be found.
Next, our code checks whether the length of array_name is equal to 0. If either of these conditions are true, our if statement will execute.
Don’t Miss: What To Prepare For A Job Interview
Q1 What Is The Difference Between Let Const And Var
Front end developer interview questions cover various variables in JavaScript. Variables are containers for strong values. Earlier, var was the only variable in JavaScript. In ES6, const and let emerged as additional options.
To get a deeper understanding of the differences between var and let, read this article.
What Language Constructions Do You Use For Iterating Over Object Properties And Array Items
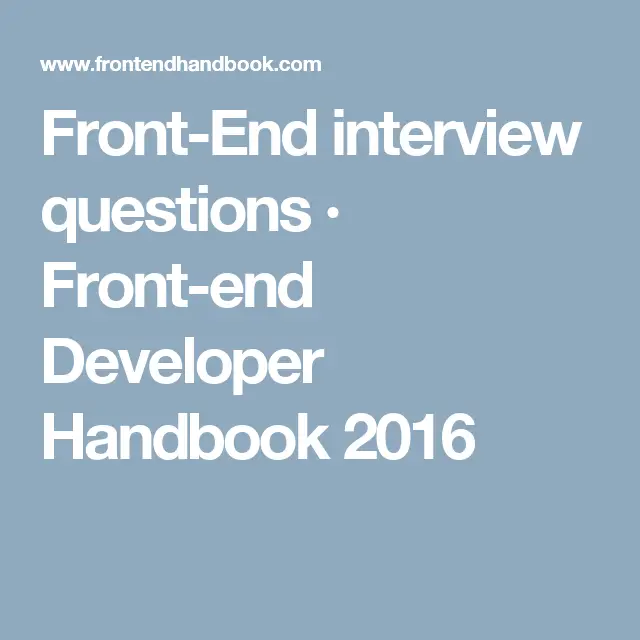
For objects:
- for-in loops – for . However, this will also iterate through its inherited properties, and you will add an obj.hasOwnProperty check before using it.
- Object.keys – Object.keys.forEach ). Object.keys is a static method that will lists all enumerable properties of the object that you pass it.
- Object.getOwnPropertyNames – Object.getOwnPropertyNames.forEach ). Object.getOwnPropertyNames is a static method that will lists all enumerable and non-enumerable properties of the object that you pass it.
For arrays:
Most of the time, I would prefer the .forEach method, but it really depends on what you are trying to do. Before ES6, we used for loops when we needed to prematurely terminate the loop using break. But now with ES6, we can do that with for-of loops. I would use for loops when I need even more flexibility, such as incrementing the iterator more than once per loop.
Also, when using the for-of loop, if you need to access both the index and value of each array element, you can do so with the ES6 Array entries method and destructuring:
const arr = for){console.log
Don’t Miss: How To Prepare For A Big Interview
What Is The Extent Of Your Experience With Promises And/or Their Polyfills
Possess working knowledge of it. A promise is an object that may produce a single value sometime in the future: either a resolved value or a reason that it’s not resolved . A promise may be in one of 3 possible states: fulfilled, rejected, or pending. Promise users can attach callbacks to handle the fulfilled value or the reason for rejection.
Some common polyfills are $.deferred, Q and Bluebird but not all of them comply with the specification. ES2015 supports Promises out of the box and polyfills are typically not needed these days.
References
Explain The Difference Between Mutable And Immutable Objects
Immutability is a core principle in functional programming, and has lots to offer to object-oriented programs as well. A mutable object is an object whose state can be modified after it is created. An immutable object is an object whose state cannot be modified after it is created.
What is an example of an immutable object in JavaScript?
In JavaScript, some built-in types are immutable, but custom objects are generally mutable.
Some built-in immutable JavaScript objects are Math, Date.
Here are a few ways to add/simulate immutability on plain JavaScript objects.
Object Constant Properties
let myObject =
In non-strict mode, the creation of b fails silently. In strict mode, it throws a TypeError.
Seal
Object.seal creates a “sealed” object, which means it takes an existing object and essentially calls Object.preventExtensions on it, but also marks all its existing properties as configurable: false.
So, not only can you not add any more properties, but you also cannot reconfigure or delete any existing properties .
Freeze
Object.freeze creates a frozen object, which means it takes an existing object and essentially calls Object.seal on it, but it also marks all “data accessor” properties as writable:false, so that their values cannot be changed.
This approach is the highest level of immutability that you can attain for an object itself, as it prevents any changes to the object or to any of its direct properties .
var immutable =Object.freeze
Pros
Cons
References
Don’t Miss: What To Write In Email After Interview
What Are Form Control And Form Groups
Form Control
- It enables validation through the Form Control class.
- It produces a new instance of this class for each input field.
- These instances allow you to verify the field’s values to see if they’ve been touched, untouched, or dirty.
Form Group
- A group of controls is represented by the FormGroup class.
- Multiple control groups are possible in a form.
- If all the controls are valid, the Form Group class returns True.
- It also includes a list of all validation problems.
Front End Developer Interview Questions And Answers 2022
Front-end web development has become one of the IT industrys most high demand domains and job roles today. It is one of the most high-paying domains with great scope for growth and earning. The fundamental skills required in this role are knowledge of CSS, HTML, JavaScript and the working of servers. This makes front-end development a valuable step toward a full-stack development career. The front-end development domain in itself is also quite comprehensive as it covers a wide range of tools and techniques. To understand a candidates grasp on each of these concepts, front-end developer interview questions are diverse and cover several domains.
In todays technology-driven world, programmers must have a high level of proficiency in coding to help them convert the requirements of a client into a finished product. In front-end developer interviews, the hiring professional is always looking for this high proficiency level in candidates who take initiative. The leading IT organisations of the world want to hire developers who can lead app building and management projects and create solutions that are responsive and interactive. This must be done keeping in mind the needs and convenience of the client.
Read Also: How To Decline A Candidate After Interview
Q24 In How Many Ways A Javascript Code Can Be Involved In An Html File
There are 3 different ways in which a JavaScript code can be involved in an HTML file:
- Inline
- Internal
- External
An inline function is a JavaScript function, which is assigned to a variable created at runtime. You can differentiate between Inline Functions and Anonymous since an inline function is assigned to a variable and can be easily reused. When you need a JavaScript for a function, you can either have the script integrated in the page you are working on, or you can have it placed in a file that you call, when needed. This is the difference between an internal script and an external script.
In Html How Do You Separate A Section Of Text
In HTML, you use the following tags to divide a chunk of text:
< br> tagIt’s a character that’s used to break up a line of text. It transfers the text flow to a new line by breaking the existing line.
< p> tagThis tag is used to create a text paragraph.
< blockquote> This tag is used to indicate big quoted passages.
Also Check: How To Reply To Interview Questions
Questions About Data Structures
Why its important
You want to get a sense of the candidates familiarity with high level programming concepts that will impact code performance. Developers with a more traditional academic background should be able to handle these questions better than self-taught programmers, so you should manage your expectations accordingly.
Sorts of questions you might ask
19. Describe the difference between a List vs. a Set
A List is an ordered sequence of elements that may be duplicated whereas a Set is a collection of distinct elements which is unordered.
20. Compare and contrast use cases for a stack vs. a queue
The main differences between stack and queue are that stack uses LIFO method to access and add data elements whereas Queue uses FIFO method to access and add data elements.
Use a queue when you want to get things out in the order that you put them in . Use a stack when you want to get things out in the reverse order than you put them in .
What Is Functional Programming
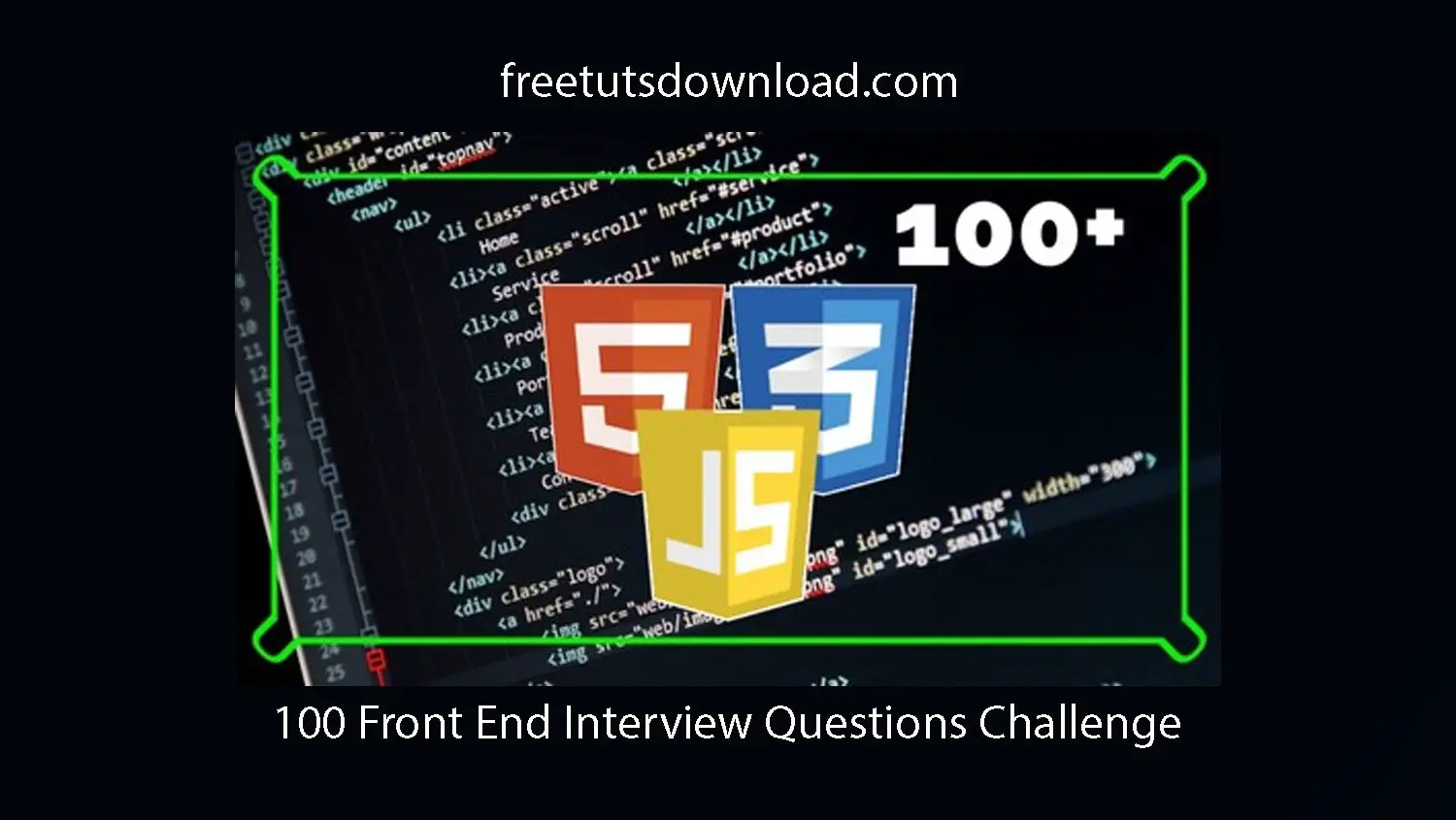
JavaScript supports functional and object-oriented programming. Functional programming is a programming paradigm where programs are written using pure functions.
Pure functions produce the same output. This makes them easier to read, debug, and interpret. One way to think about pure functions is like a calculator. If you evaluate 9 multiplied by 9 in a calculator, 81 will always be returned.
JavaScript offers features like first-class functions and higher-order functions to support the functional programming paradigm. Other functional programming languages include Lisp, Haskell, and Elm.
Recommended Reading: How To Pass The Us Citizenship Test And Interview
How Can You Share Code Between Files
This depends on the JavaScript environment.
On the client , as long as the variables/functions are declared in the global scope , all scripts can refer to them. Alternatively, adopt the Asynchronous Module Definition via RequireJS for a more modular approach.
On the server , the common way has been to use CommonJS. Each file is treated as a module and it can export variables and functions by attaching them to the module.exports object.
ES2015 defines a module syntax which aims to replace both AMD and CommonJS. This will eventually be supported in both browser and Node environments.
How Do You Organize Your Code
In the past, I’ve used Backbone for my models which encourages a more OOP approach, creating Backbone models and attaching methods to them.
The module pattern is still great, but these days, I use React/Redux which utilize a single-directional data flow based on Flux architecture. I would represent my app’s models using plain objects and write utility pure functions to manipulate these objects. State is manipulated using actions and reducers like in any other Redux application.
I avoid using classical inheritance where possible. When and if I do, I stick to these rules.
Also Check: How To Send Interview Thank You Email
What Is A Sql Injection
Another type of attack. If you have a search form, for example, and when a user types in a name like “Kelly” you take that string and do an SQL lookup for it. Let’s say your SQL looks like this:
`SELECT * FROM Users WHERE UserId = yourVariable`
An attacker could put something malicious in the search bar like `Kelly OR 1=1`, and now it will return a list of all users instead of just the requested one.
Basic Front End Developer Interview Questions
1. What does DOCTYPE stand for, and what does it do?
Answer: DOCTYPE stands for Document Type, and it is associated with DTD . The task of DOCTYPE is to tell the user browser what version of HTML specification the document is representing. For example, the doctype declaration for HTML 5 is < !DOCTYPE HTML> .
Check HTML vs HTML5 here .
2. What is the difference between null and undefined?
Answer: undefined represents no value at all, whereas null is a value. When we define a variable and do not assign it a value, then the variable would be undefined. On the other hand, if we want a variable with no value, then we can simply assign a null value to it.
Example:
var x var y= null Here x is undefined and y is null.
3. What is Lazy Loading?
Answer: It is a technique of loading content on the user’s browser according to the user’s needs. This helps to optimize the resource usage for the user as well as the server. For instance, if you search for something on Google Search then in return it shows only a few results on the first page, rather than filling the user request by all the search results in bulk. Google Search shows only a few contents and gives an option to the user to fetch more if needed.
4. What is Coercion in JS?
Answer: Coercion or Type Coercion in JS is a method of converting the data type of a variable. Using the coercion process, we can convert a string to a number, or an object to a Boolean, and so on. For example:
For example:
2. Explicit Coercion
Don’t Miss: How To Interview Job Candidates
What Is The `this` Keyword In Javascript
`this` is a little tricky in JavaScript. Its value is determined by what the function you are inside of is called. In the global state, `this` is set to the window object. The value of `this` also depends on whether or not you are in strict mode. Inside a top-level function, a strict mode `this` will be undefined, whereas a non-strict mode `this` will be the window object. It’s also worth knowing that the value of `this` can be overwritten with the bind method.
How Is A Web Developer Different From A Web Designer
Web Developer |
|
Build web applications using languages like HTML, CSS, and JavaScript |
Design web applications using tools like Adobe Photoshop, Sketch |
They frequently use JavaScript frameworks for more streamlined development |
They frequently use Adobe Creative Cloud for most of the design needs |
It requires good coding skills |
It requires good graphic design skills |
Have to keep themselves up to date with the latest web frameworks and libraries |
Have to keep themselves up to date with the latest design trends and color palettes |
Also Check: What Type Of Questions Should You Ask In An Interview
Q27 What Is The Difference Between Local Storage & Session Storage
Local Storage The data is not sent back to the server for every HTTP request reducing the amount of traffic between client and server. It will stay until it is manually cleared through settings or program.
Session Storage It is similar to local storage the only difference is while data stored in local storage has no expiration time, data stored in session storage gets cleared when the page session ends. Session Storage will leave when the browser is closed.
In case you are facing any challenges with these JavaScript Interview Questions, please comment on your problems in the section below.
Whats The Difference Between A Relative Fixed Absolute And Statically Positioned Element

- Relative the element is positioned relative to its normal position.
- Fixed the element is positioned related to the browser window.
- Absolute the element is positioned absolutely to its first positioned parent.
- Static this is the default value, all elements are in order as they appear in the document.
Don’t Miss: When To Send Follow Up Email After Interview
Explain Why The Following Doesn’t Work As An Iife: Function Foo What Needs To Be Changed To Properly Make It An Iife
IIFE stands for Immediately Invoked Function Expressions. The JavaScript parser reads function foo as function foo and , where the former is a function declaration and the latter is an attempt at calling a function but there is no name specified, hence it throws Uncaught SyntaxError: Unexpected token ).
Here are two ways to fix it that involves adding more parentheses: ) and ). Statements that begin with function are considered to be function declarations by wrapping this function within , it becomes a function expression which can then be executed with the subsequent . These functions are not exposed in the global scope and you can even omit its name if you do not need to reference itself within the body.
You might also use void operator: void function foo . Unfortunately, there is one issue with such approach. The evaluation of given expression is always undefined, so if your IIFE function returns anything, you can’t use it. An example:
const foo =void{return'foo'
What Is Progressive Rendering
Progressive rendering is a process that is utilized generally to boost the web page’s rendering content process. Now the rendering process is utilized in modern web development to enhance the mobile data uses of the user, async HTML fragments, prioritizing visible content, and lazy loading of images.
Recommended Reading: How To Interview With Google
Q46 What Will Be The Output Of The Following Code
var X = var Output = ) console.log
The output would be undefined. The delete operator is used to delete the property of an object. Here, x is an object which has the property foo, and as it is a self-invoking function, we will delete the foo property from object x. After doing so, when we try to reference a deleted property foo, the result is undefined.
What Are Pipes In Angular Explain Its Types
Pipes are simple functions that accept an input value, process it, and return an altered value as an output, in a more technical sense. Angular has several built-in pipes. You can, however, make custom pipes to meet your specific requirements.
The following are some major features:
Pure Pipes
These are pipes with just pure functions. As a result, a pure pipe uses no internal state, and the output remains constant as long as the parameters provided remain constant. When Angular detects a change in the arguments being given, it invokes the pipe. Throughout all components, a single instance of the pure pipe is used.
Impure Pipes
Angular calls an impure pipe for every change detection cycle, independent of the change in the input fields. For these pipes, it produces multiple pipe instances. The inputs to these pipes can be changed.
All pipes are pure by default. On the other hand, the pure attribute can be used to identify impure pipes, as demonstrated below.
export class DemopipePipe implements PipeTransform {
Also Check: How To Interview An Applicant For A Job