What Is Scope Resolution In Python
Sometimes objects within the same scope have the same name but function differently. In such cases, scope resolution comes into play in Python automatically. A few examples of such behavior are:
- Python modules namely ‘math’ and ‘cmath’ have a lot of functions that are common to both of them – log10, acos, exp etc. To resolve this ambiguity, it is necessary to prefix them with their respective module, like math.exp and cmath.exp.
- Consider the code below, an object temp has been initialized to 10 globally and then to 20 on function call. However, the function call didn’t change the value of the temp globally. Here, we can observe that Python draws a clear line between global and local variables, treating their namespaces as separate identities.
temp = 10# global-scope variabledeffunc: temp = 20# local-scope variableprintprint # output => 10func # output => 20print # output => 10
This behavior can be overridden using the global keyword inside the function, as shown in the following example:
temp = 10# global-scope variabledeffunc:global temp temp = 20# local-scope variableprintprint # output => 10func # output => 20print # output => 20
Question : Find Pairs Of Integers In A List So That Their Sum Is Equal To The Integer X
This problem is interesting. The straightforward solution is to use two nested for loops and check for each combination of elements whether their sum is equal to integer x. Here is what I mean:
def find_pairs: pairs = for in enumerate: for in enumerate: if element_1 + element_2 == x: pairs.add) return pairsl = print)
Fail! It throws an exception: AttributeError: list object has no attribute add
This is what I meant: its easy to underestimate the difficulty level of the puzzles, only to learn that you did a careless mistake again. So the corrected solution is this:
def find_pairs: pairs = for in enumerate: for in enumerate: if element_1 + element_2 == x: pairs.append) return pairsl = print)
Now it depends whether your interviewer will accept this answer. The reason is that you have a lot of duplicated pairs. If he asked you to remove them, you could simply do a post-processing by removing all the duplicates from the list.
Actually, this is a common interview question as well .
Here is another beautiful one-liner solution submitted by one of our readers:
# Solution from user Martin l = match = 9res = setprint
Are Access Specifiers Used In Python
Python does not make use of access specifiers specifically like private, public, protected, etc. However, it does not derive this from any variables. It has the concept of imitating the behaviour of variables by making use of a single or double underscore as prefixed to the variable names. By default, the variables without prefixed underscores are public.
Example:
# to demonstrate access specifiersclassInterviewbitEmployee:# protected members _emp_name = None _age = None# private members __branch = None# constructordef__init__: self._emp_name = emp_name self._age = age self.__branch = branch#public memberdefdisplay:print
You May Like: Examples Of Motivational Interviewing Techniques
What Is The Difference Between Python Arrays And Lists
- Arrays in python can only contain elements of same data types i.e., data type of array should be homogeneous. It is a thin wrapper around C language arrays and consumes far less memory than lists.
- Lists in python can contain elements of different data types i.e., data type of lists can be heterogeneous. It has the disadvantage of consuming large memory.
import arraya = array.arrayfor i in a:print #OUTPUT: 1 2 3a = array.array #OUTPUT: TypeError: an integer is required a = for i in a:print #OUTPUT: 1 2 string
Question 1: Find All Permutations Of A String
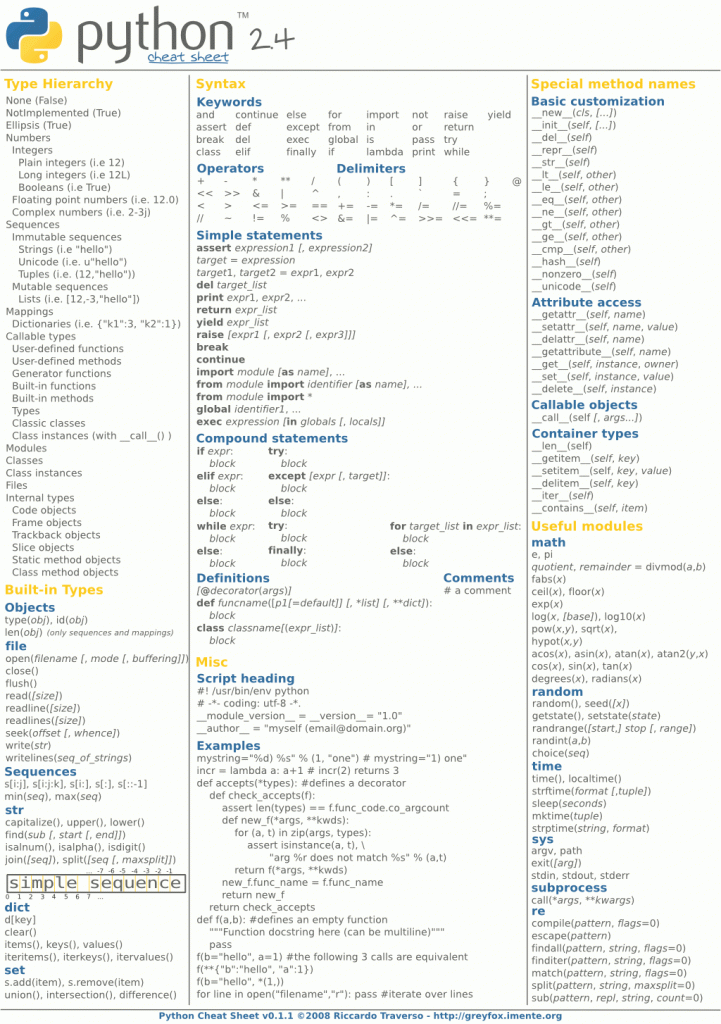
This is a common problem of many coding interviews. Similar to the anagrams problem presented in the question above, the purpose of this question is twofold. First, the interviewers check your creativity and ability to solve algorithmic problems. Second, they check your pre-knowledge of computer science terminology.
What is a permutation? You get a permutation from a string by reordering its characters. Lets go back to the anagram problem. Two anagrams are permutations from each other as you can construct the one from the other by reordering characters.
Here are all permutations from a few example strings:
- hello
- hi
- bye
Conceptually, you can think about a string as a bucket of characters. Lets say the string has length n. In this case, you have n positions to fill from the bucket of n characters. Having filled all n positions, you obtain a permutation from the string. You want to find ALL such permutations.
My first idea is to solve this problem recursively. Suppose, we already know all permutations from a string with n characters. Now, we want to find all permutations with n+1 characters by adding a character x. We obtain all such permutations by inserting x into each position of an existing permutation. We repeat this for all existing permutations.
However, as a rule-of-thumb: avoid overcomplicating the problem in a coding interview at all costs! Dont try to be fancy!
Thus, I went back to implement the recursive solution described above.
Recommended Reading: How To Present Your Portfolio At An Interview
Introduction To Python 3 Cheat Sheet
Python 3 cheat sheets are one of the most effective methods of learning. A good cheat sheet concentrates on the most important learning points while ignoring the rest. All the page is used to give value in this cheat sheet, which covers all we need to know to progress from beginner to advance. Python 3 cheat sheet covers the conditionals, types of containers, modules, conversions, algebra, and formatting.
The following article provides an outline for Python 3 Cheat Sheet. Python is a high-level programming language that was designed by Guido Van Rossum and developed by the Python software foundation in 1990. It has a paradigm of Object-oriented, imperative and procedural reflective. The parent company of python is a Python software foundation. It is used for web development, software development, and system scripting.
Web development, programming languages, Software testing & others
Q101 How Do You Make 3d Plots/visualizations Using Numpy/scipy
Ans: Like 2D plotting, 3D graphics is beyond the scope of NumPy and SciPy, but just as in the 2D case, packages exist that integrate with NumPy. Matplotlib provides basic 3D plotting in the mplot3d subpackage, whereas Mayavi provides a wide range of high-quality 3D visualization features, utilizing the powerful VTK engine.
Next in this Python Interview Questions blog, lets have a look at some MCQs
Read Also: What To Write In A Post Interview Email
Q84 Explain What Flask Is And Its Benefits
Ans: Flask is a web microframework for Python based on Werkzeug, Jinja2 and good intentions BSD license. Werkzeug and Jinja2 are two of their dependencies. This means it will have little to no dependencies on external libraries. It makes the framework light while there is a little dependency to update and fewer security bugs.
A session basically allows you to remember information from one request to another. In a flask, a session uses a signed cookie so the user can look at the session contents and modify them. The user can modify the session if only it has the secret key Flask.secret_key.
What Is The Use Of Help And Dir Functions
help function in Python is used to display the documentation of modules, classes, functions, keywords, etc. If no parameter is passed to the help function, then an interactive help utility is launched on the console.dir function tries to return a valid list of attributes and methods of the object it is called upon. It behaves differently with different objects, as it aims to produce the most relevant data, rather than the complete information.
- For Modules/Library objects, it returns a list of all attributes, contained in that module.
- For Class Objects, it returns a list of all valid attributes and base attributes.
- With no arguments passed, it returns a list of attributes in the current scope.
Recommended Reading: How To Conduct A Successful Interview
What Is Supervised Learning
Supervised learning is a machine learning algorithm of inferring a function from labeled training data. The training data consists of a set of training examples.
Example: 01
Knowing the height and weight identifying the gender of the person. Below are the popular supervised learning algorithms.
- Support Vector Machines
What Is Python What Are The Benefits Of Using Python
Python is a high-level, interpreted, general-purpose programming language. Being a general-purpose language, it can be used to build almost any type of application with the right tools/libraries. Additionally, python supports objects, modules, threads, exception-handling, and automatic memory management which help in modelling real-world problems and building applications to solve these problems.
Benefits of using Python:
- Python is a general-purpose programming language that has a simple, easy-to-learn syntax that emphasizes readability and therefore reduces the cost of program maintenance. Moreover, the language is capable of scripting, is completely open-source, and supports third-party packages encouraging modularity and code reuse.
- Its high-level data structures, combined with dynamic typing and dynamic binding, attract a huge community of developers for Rapid Application Development and deployment.
Recommended Reading: Is An Interview With God A Christian Movie
What Is Lambda In Python Why Is It Used
Lambda is an anonymous function in Python, that can accept any number of arguments, but can only have a single expression. It is generally used in situations requiring an anonymous function for a short time period. Lambda functions can be used in either of the two ways:
- Assigning lambda functions to a variable:
mul = lambda a, b : a * bprint) # output => 10
- Wrapping lambda functions inside another function:
defmyWrapper:returnlambda a : a * nmulFive = myWrapperprint) # output => 10
How Do You Make Sure Which Machine Learning Algorithm To Use
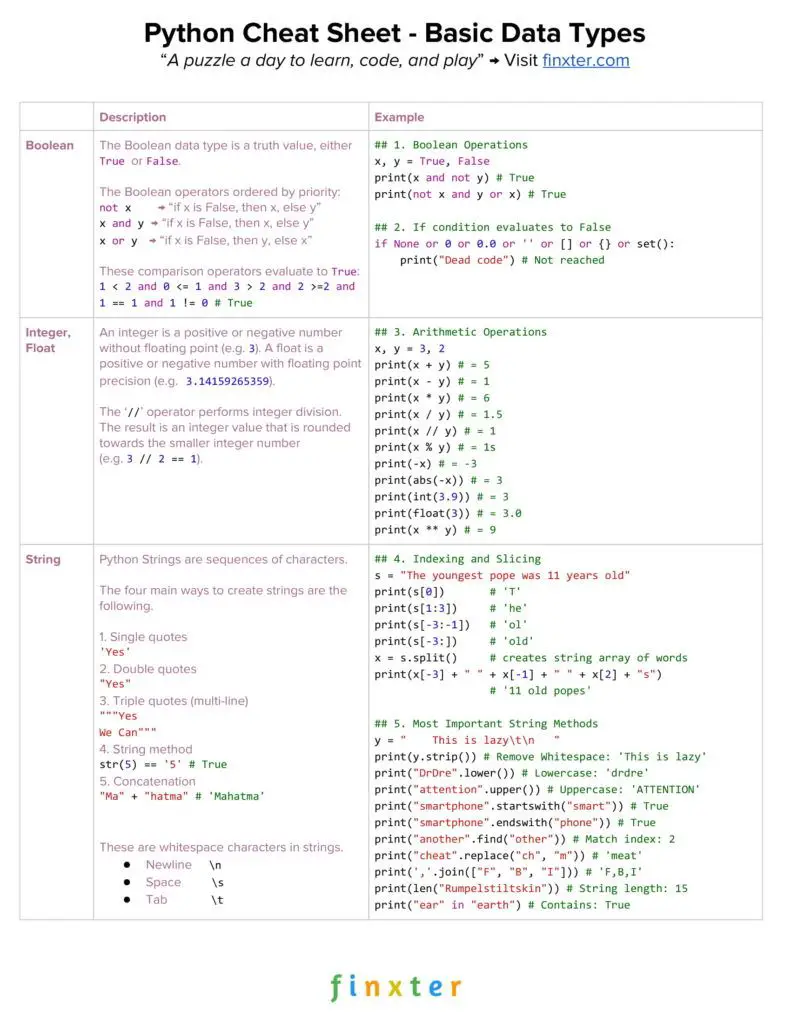
It completely depends on the dataset we have. If the data is discrete we use SVM. If the dataset is continuous we use linear regression.
So there is no specific way that lets us know which ML algorithm to use, it all depends on the exploratory data analysis .
EDA is like interviewing the dataset As part of our interview we do the following:
- Classify our variables as continuous, categorical, and so forth.
- Summarize our variables using descriptive statistics.
- Visualize our variables using charts.
Based on the above observations select one best-fit algorithm for a particular dataset.
Read Also: How To Interview An Engineer
How To Negotiate A Job Offer
Negotiating a job offer from a FAANGcompany can seem daunting. After all, you have just managed to snag a cushy position. You may be wondering if negotiating salary will put you at a disadvantage. But as a software engineer, you deserve the best, and you shouldnât settle for anything else. Here are our hot tips for negotiating a job offer:
- Do your homework to find out the salary range for the position. This will give you a fair idea about the room for negotiation.
- It is best to let the company make the first move and tell you the offer. In case they ask you to give them what you expect, always quote the higher end of the expected salary range.
- Once you receive the offer, it is time to come back with a counter. But donât quote a number out of thin air. Ask around, take the advice of software engineers or software developers working in FAANG, speak to other managers, and then give them a number.
- Ask about the benefits that come along with the compensation. Even if the company is not ready to negotiate on the compensation, try to see if you can get more benefits like health insurance cover for your family members or travel allowance, etc.
- If you are not happy with the offer, donât be afraid to walk away. It is better to try elsewhere than forcing yourself to work for a lesser salary package.
Python Interview Cheat Sheet
Python Cheat Sheet can be used also for your Python Job interview. Before your technical interview, you can check this sheet and you can use it as Python Interview Sheet. You can find all the basic terms of Python programming languages in this cheat sheet. So, the questions in you technical Python interview will be mostly on this Python Interview Cheat Sheet.
In your Python interview, maybe they will ask how to use Python tubles? Maybe they want to learn, how to get the last term in a Python list. Or maybe their question will be, how to get different types of inputs from the user.
Beside basic questions, in the Python Interview, they can ask complex questions about a programming code part. They can ask any specific parts of a Python program in the interview. Python Interview Cheat Sheet can remind you basic terms for this complex questions.
Don’t Miss: How To Prepare Software Engineer Interview
Programming Languages You Should Master For Faang Coding Interviews
Mastering programming languages is a non-negotiable if you want to excel in FAANG coding interviews. Programming languages form the building blocks of everything that a software engineer is required to do.
Hereâs a list of all coding technologies that should form part of your programming interview cheat sheet before you step inside the interview room.
- âAlgorithms: In simple terms, algorithms refer to a formula for solving a problem. The various types of algorithms you should be familiar with include:
Bubble sort, insertion sort, and merge sort: The three classic algorithms are bubble sort, insertion sort, and merge sort. Bubble sort is one of the simplest sorting algorithms. This formula considers an entire array and compares each neighboring number. The numbers are then swapped, and the pattern continues for the whole list. In other words, if adjacent elements are in the wrong order, this algorithm swaps the adjacent elements. The next sorting algorithm is the insertion sort, where the elements are compared with each other in a sequential manner and arranged in a particular order. It is similar to arranging a deck of cards. The final one is merge sort which is also known as a divide and conquer algorithm. It divides an unsorted list into a number of sublists, and each contains a single element. Then it merges these sublists in a repeated fashion to create a new sublist.
Detect Cycle In Undirected Graph
Given an undirected graph, return true if the given graph contains at least one cycle, else return false.Input : graph = , , , , ]Output: True
For undirected graph, we dont need to keep track of the whole stack tree . For every vertex v, if there is an adjacent u such that u is already visited and u is not a parent of v, then there is a cycle in the graph.
Time complexity is the same as the normal DFS, which is O.
Recommended Reading: How To Pass An Interview
What Are Dict And List Comprehensions
Python comprehensions, like decorators, are syntactic sugar constructs that help build altered and filtered lists, dictionaries, or sets from a given list, dictionary, or set. Using comprehensions saves a lot of time and code that might be considerably more verbose . Let’s check out some examples, where comprehensions can be truly beneficial:
- Performing mathematical operations on the entire list
my_list = squared_list = # list comprehension# output => squared_dict = # dict comprehension# output =>
- Performing conditional filtering operations on the entire list
my_list = squared_list = # list comprehension# output => squared_dict = # dict comprehension# output =>
- Combining multiple lists into one
Comprehensions allow for multiple iterators and hence, can be used to combine multiple lists into one.
a = b = # parallel iterators# output => # nested iterators# output =>
- Flattening a multi-dimensional list
A similar approach of nested iterators can be applied to flatten a multi-dimensional list or work upon its inner elements.
my_list = ,,]flattened = # output =>
Note: List comprehensions have the same effect as the map method in other languages. They follow the mathematical set builder notation rather than map and filter functions in Python.
Python For Data Science
This Python cheat sheet is specially designed for data sciences purposes, unlike the previous ones intended for development.
However, it doesnt mean that it only covers data science, as it covers almost all the basics of Python, which is equally helpful for developers.
It is a one-page cheat sheet that aims to provide quick answers to data types and conversions, lists, operations, libraries, NumPy arrays, methods, variables and calculations, strings and functions methods, and helping you in installing Python.
Don’t Miss: What To Wear To A Job Interview Female
Question : Remove All Duplicates From An Integer List
Given a list, the goal is to remove all elements, which exist more than once in the list. Note that you should be careful not to remove elements while iterating over a list.
Wrong example of modifying a list while iterating over it :
lst = list)for element in lst: if element > = 5: lst.removeprint#
As you can see, modifying the sequence over which you iterate causes unspecified behavior. After it removes the element 5 from the list, the iterator increases the index to 6. The iterator assumes this is the next element in the list. However, thats not the case. As we have removed the element 5, element 6 is now at position 5. The iterator simply ignores the element. Hence, you get this unexpected semantics.
Yet, there is a much better way how to remove duplicates in Python. You have to know that sets in Python allow only a single instance of an element. So after converting the list to a set, all duplicates will be removed by Python. In contrast of the naive approach , this method has linear runtime complexity. The reason is that creation of a set is linear in the number of set elements. Now, we simply have to convert the set back to a list and voilà, the duplicates are removed.
lst = list) + list)lst = list)print# # Does this also work for tuples? Yes!lst = lst = list)print#