Is Indentation Required In Python
Indentation in Python is compulsory and is part of its syntax.
All programming languages have some way of defining the scope and extent of the block of codes. In Python, it is indentation. Indentation provides better readability to the code, which is probably why Python has made it compulsory.
Learn the Pros and Cons of Python in our comprehensive blog on the Advantages and Disadvantages of Python.
Why Isn’t All The Memory De
- When Python quits, some Python modules, especially those with circular references to other objects or objects referenced from global namespaces, are not necessarily freed or deallocated.
- Python would try to de-allocate/destroy all other objects on exit because it has its own efficient cleanup mechanism.
- It is difficult to de-allocate memory that has been reserved by the C library.
What Is The Naming Convention In Python For Variables And Functions
Answer#
Function names should be lowercase, with words separated by underscores as necessary to improve readability.
Variable names follow the same convention as function names.
mixedCase is allowed only in contexts where thats already the prevailing style , to retain backwards compatibility.
The Google Python Style Guide has the following convention:
module_name, package_name, ClassName, method_name, ExceptionName, function_name, GLOBAL_CONSTANT_NAME, global_var_name, instance_var_name, function_parameter_name, local_var_name.
A similar naming scheme should be applied to a CLASS_CONSTANT_NAME
Don’t Miss: An Interview With God Netflix
What Are Iterators In Python
- An iterator is an object.
- It remembers its state i.e., where it is during iteration
- __iter__ method initializes an iterator.
- It has a __next__ method which returns the next item in iteration and points to the next element. Upon reaching the end of iterable object __next__ must return StopIteration exception.
- It is also self-iterable.
- Iterators are objects with which we can iterate over iterable objects like lists, strings, etc.
classArrayList:def__init__: self.numbers = number_listdef__iter__: self.pos = 0return selfdef__next__:if): self.pos += 1return self.numberselse:raise StopIterationarray_obj = ArrayListit = iterprint) #output: 2print) #output: 3print)#Throws Exception#Traceback :#...#StopIteration
Write A Class To Represent An Integer And A Function To Return Whether Or Not Is A Palindrome
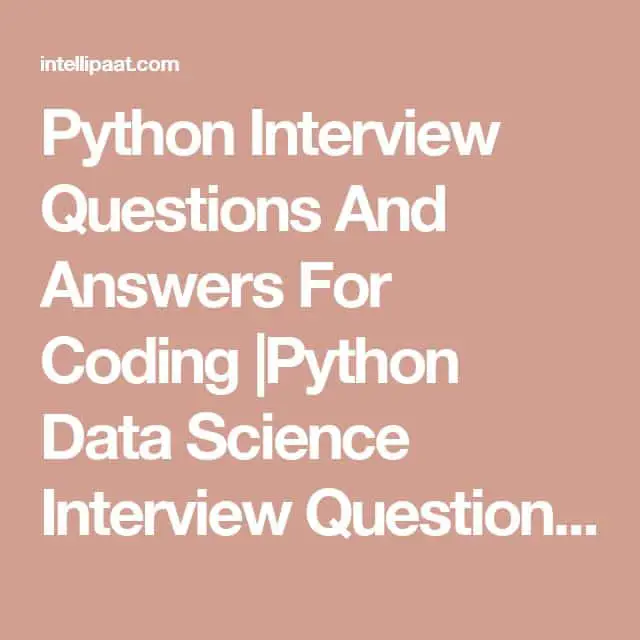
This question tests your ability to write a simple class in Python as well as your ability to think quickly by writing a function to test whether an integer is a palindrome or not. An integer is considered a palindrome if it reads the same forward and backward. The number 34543 is an example of a palindrome, while the number 123 is a non-example. One possible solution to this problem is shown below.
It is a good practice during a coding interview to pay attention to details, including checking for error conditions during the initialization of your class and including docstrings for all classes and functions that you define. Even if you donât explicitly write these down on the whiteboard due to space or time constraints, make sure you mention how you would normally provide such details in actual code.
Don’t Miss: How To Interview For A Leadership Position
What Is Tuple Matching In Python
Tuple Matching in Python is a method of grouping the tuples by matching the second element in the tuples. It is achieved by using a dictionary by checking the second element in each tuple in python programming. However, we can make new tuples by taking portions of existing tuples.
Syntax:To write an empty tuple, you need to write as two parentheses containing nothing-tup1 =
What Do You Understand About Pandas Groupby
A pandas groupby is a feature supported by pandas that are used to split and group an object. Like the sql/mysql/oracle groupby it is used to group data by classes, and entities which can be further used for aggregation. A dataframe can be grouped by one or more columns.
Code:
To perform groupby type the following code:
df.groupby.count
Don’t Miss: How To Prepare For Sdet Interview
What Advantage Does The Numpy Array Have Over A Nested List
Numpy is written in C so that all its complexities are backed into a simple to use a module. Lists, on the other hand, are dynamically typed. Therefore, Python must check the data type of each element every time it uses it. This makes Numpy arrays much faster than lists.
Numpy has a lot of additional functionality that list doesnt offer for instance, a lot of things can be automated in Numpy.
Can I Request For A Support Session If I Need To Better Understand The Topics
Intellipaat is offering 24/7 query resolution, and you can raise a ticket with the dedicated support team at any time. You can avail of email support for all your queries. If your query does not get resolved through email, we can also arrange one-on-one sessions with our support team. However, 1:1 session support is provided for a period of 6 months from the start date of your course.
Read Also: Data Scientist Interview Questions And Answers
What Are Namespaces In Python
A namespace is used for creating unique object names that will not cause a conflict later. Some namespaces are:
- local namespace is temporarily created for a functional call and as soon as the call returns, it is cleared
- global namespace contains names from modules and packages
- built-in namespace includes names and functions of core Python
Ready To Nail Your Next Coding Interview
Whether youâre a coding engineer gunning for a software developer or software engineer role, a tech lead, or youâre targeting management positions at top companies, IK offers courses specifically designed for your needs to help you with your technical interview preparation!
If youâre looking for guidance and help with getting started, As pioneers in the field of technical interview preparation, we have trained thousands of software engineers to crack the most challenging coding interviews and land jobs at their dream companies, such as Google, Facebook, Apple, Netflix, Amazon, and more!
You May Like: How To Crack Job Interview
Explain The : : Enter: : And : : Exit: : Methods In Python
Answer#
Using these magic methods allows you to implement objects which can be used easily with the with statement.
The idea is that it makes it easy to build code which needs some cleandown code executed .
A useful example could be a database connection object :
class DatabaseConnection: def __enter__: # make a database connection and return it ... return self.dbconn def __exit__: # make sure the dbconnection gets closed self.dbconn.close ...
As explained above, use this object with the with statement .
with DatabaseConnection as mydbconn:PEP343 The with statement has a nice writeup as well.
Explain Join And Split Functions In Python
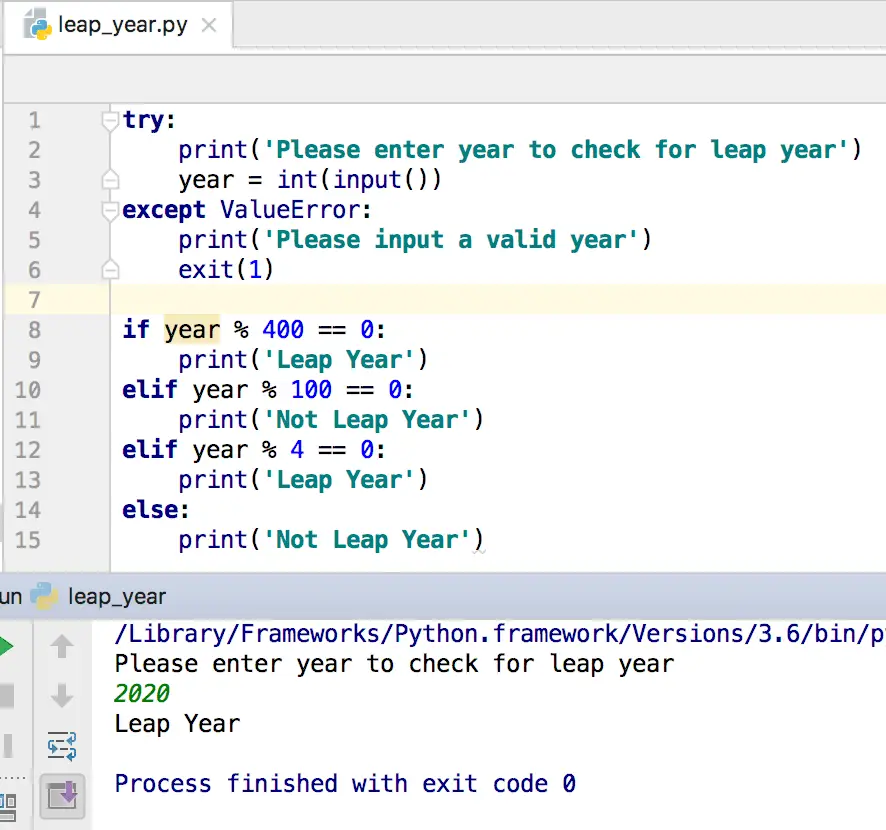
The join function can be used to combine a list of strings based on a delimiter into a single string.
The split function can be used to split a string into a list of strings based on a delimiter.
string = “This is a string.”
string_list = string.split #delimiter is space character or
print #output:
print) #output: This is a string.
You May Like: How To Prepare For Amazon Behavioral Interview
What Are Negative Indexes And Why Do We Utilize Them
Python sequences are indexed, and they include both positive and negative values. Positive numbers are indexed with ‘0’ as the first index and ‘1’ as the second index, and so on.
The index for a negative number begins with ‘-1,’ which is the last index in the sequence, and ends with ‘-2,’ which is the penultimate index, and the sequence continues like a positive number. The negative index is used to eliminate all new-line spaces from the string and allow it to accept the last character S. The negative index can also be used to represent the correct order of the string.
Python Basic Interview Questions
1. Briefly explain some characteristics of Python.
Python is a general purpose, high-level, interpreted language. It was specifically developed with the purpose of making the content readable. Python has often been compared to the English language, and it also has fewer syntactic constructions compared to other languages.
2. What are some distinct features of Python?
Some distinct features of Python are:
3. What is Pythonpath?
A Pythonpath tells the Python interpreter to locate the module files that can be imported into the program. It includes the Python source library directory and source code directory. You can preset Pythonpath as a Python installer.
4. Why do we use the Pythonstartup environment variable?
The variable consists of the path in which the initialization file carrying the Python source code can be executed. This is needed to start the interpreter.
5. What is the Pythoncaseok environment variable?
The Pythoncaseok environment variable is applied in Windows with the purpose of directing Python to find the first case insensitive match in an import statement.
Read Also: How To Do A Telephone Interview
Additional Situational Interview Questions For Python Developers
What do you do if theres a disagreement within your team? |
Are you comfortable giving in-depth presentations? |
Are you able to explain complex technologies in simple terms? |
What is your preferred way of communication with the team? |
How do you convince someone to agree with you? |
How to deal with a team member who disagrees with you? |
Have you ever worked directly with clients or have been in a customer-facing role in the past? If not, would you like to? |
What was the last presentation you gave? |
What are the qualities of a successful team or project leader? |
What Is The Difference Between Nparray And Npasarray
What is the difference between NumPys np.array and np.asarray? When should I use one rather than the other? They seem to generate identical output.
Answer#
The definition of asarray is:
def asarray:return array
So it is like array, except it has fewer options, and copy=False. array has copy=True by default.
The main difference is that array will make a copy of the object, while asarray will not unless necessary.
Also Check: What To Ask When Being Interviewed
How To Pass A Dictionary To A Function As Keyword Parameters In Python
Id like to call a function in python using a dictionary with matching key-value pairs for the parameters.
Here is some code:
d = dictdef f: printf#This prints but I'd like it to just print test.#I'd like it to work similarly for more parameters:d = dictdef f2: printf2#Is this possible?
Answer#
Just include the ** operator to unpack the dictionary. This solves the issue of passing a dictionary to a function as a keyword parameter.
So here is an example:
d = dictdef f2: print p1, p2f2
What Is The Difference Between Range & Xrange
Functions in Python, range and xrange are used to iterate in a for loop for a fixed number of times. Functionality-wise, both these functions are the same. The difference comes when talking about Python version support for these functions and their return values.
range Method | xrange Method |
In Python 3, xrange is not supported instead, the range function is used to iterate in for loops | The xrange function is used in Python 2 to iterate in for loops |
It returns a list | It returns a generator object as it doesnt really generate a static list at the run time |
It takes more memory as it keeps the entire list of iterating numbers in memory | It takes less memory as it keeps only one number at a time in memory |
Recommended Reading: What Questions To Ask In An Interview At The End
Python Interview Questions & Answers 2023
Q 1) What is the difference between a module and a package in Python?
A 1) Each Python program file is a module that imports other modules like objects. Thus, a module is a way to structure the program. The folder of a Python program is called a package of modules.
Refer to the below-mentioned table for differences-
Module | |
A module is responsible to hold file_init_.py for user-oriented code. | Does not apply to any module in runtime for any user-specific code. |
Modifies the user-interpreted code. | A file containing python code. |
This can be asked during python interview questions make sure to categories your answer and give your response that is structural in manner.
Our learners also read python course free!
Q 2) What are the built-in types available in Python?
A 2) One of the most common python interview question, There are mutable and immutable built-in types.
The mutable ones include:
Data Science Courses |
Q 3) What is lambda function in Python?
A 3) It is often used as an inline function and is a single expression anonymous function. It is used to make a new function object and return them at runtime.
Lambda is an anonymous function in Python that can accept any number of arguments and can have any number of parameters. However, the lambda function can have only a single expression or statement. Usually, it is used in situations that require an anonymous function for a short time period. Lambda functions can be used in either of the two ways:
a = lambda x,y : x+y
print)
What Are The Important Features Of Python
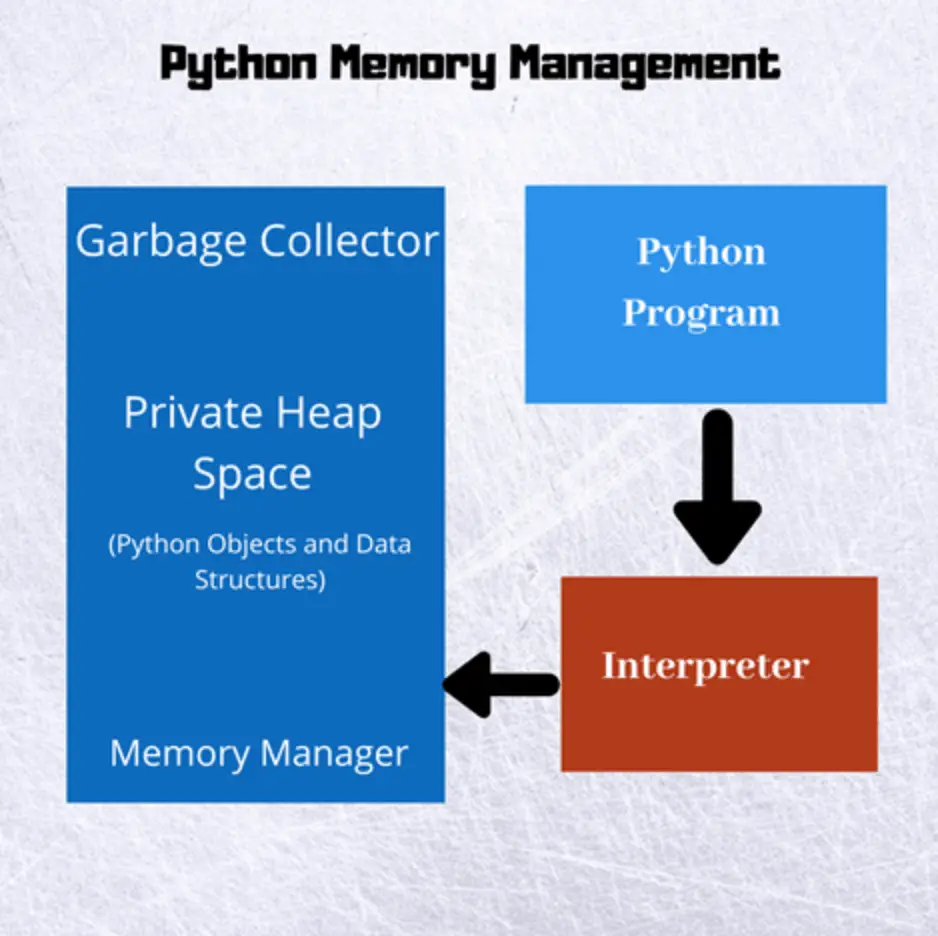
- Python is a scripting language. Python, unlike other programming languages like C and its derivatives, does not require compilation prior to execution.
- Python is dynamically typed, which means you don’t have to specify the kinds of variables when declaring them or anything.
- Python is well suited to object-oriented programming since it supports class definition, composition, and inheritance.
Recommended Reading: Accounts Receivable Specialist Interview Questions
What Is Python If Statement
Python if Statement is used for decision-making operations. It contains a body of code that runs only when the condition given in the if statement is true. If the condition is false, then the optional else statement runs, which contains some code for the else condition.
When you want to justify one condition while the other condition is not true, then you use Python if-else statement.
Python if Statement Syntax:
Lets see an example of Python if else Statement:
Lets see an example of Python if else Statement:
def main: x,y =2,8 if: st= "x is less than y" printif __name__ == "__main__": main
Q Explain The Various File Processing Modes Available In Python
Questions based on file processing are essential during the Python interview.
Read-only, write-only, read-write, and append are the three file processing modes in Python. The previous models are renamed “rt” for read-only, “wt” for write, etc. Similarly, a binary file can be opened by preceding it with “b” and the file accessing flags .
Must Read: Top 20 Red Hat Linux System Administrator Interview Questions
Recommended Reading: How To Prep For Coding Interview
Explain How You Can Minimize The Memcached Server Outages In Your Python Development
- When one instance fails, several of them goes down, this will put a larger load on the database server when lost data is reloaded as the client make a request. To avoid this, if your code has been written to minimize cache stampedes, then it will leave a minimal impact
- Another way is to bring up an instance of memcached on a new machine using the lost machines IP address
- Code is another option to minimize server outages as it gives you the liberty to change the Memcached server list with minimal work
- Setting timeout value is another option that some Memcached clients implement for Memcached server outage. When your Memcached server goes down, the client will keep trying to send a request till the time-out limit is reached.
Q Is It Possible To Generate A Random Number Using Python
Python’s random module is used here to come up with a random.
Consider some of the following: An integer number in the range of 1 to 0.
import random
To generate an integer in between a certain range :
import random
n = random.randint
As a Python interview question, the panelists can ask you to write a basic program like this one in Python.
Recommended Reading: Cracking The Pm Interview Audiobook
What Is The Use Of Managepy
It is an automatically built file inside each Django project. It is a flat wrapper encompassing the Django-admin.py. It possesses the following usage:
- It establishes your project’s package on sys.path.
- It fixes the DJANGO_SETTING_MODULE environment variable to point to your project’s setting.py file.
Explain The G Object In Flask
Ans: If we want to hold any data during the application context, the Flask g object is used as a global namespace for keeping any data. Its not suitable for storing data within requests. Actually, this letter g stands for global. Suppose we need to store a global variable in an application context, then g object is best in place of creating global variables. Here the g object will work as a request in a separate Flask g object. It always saves self-defined global variables.
You May Like: Embedded C Coding Interview Questions
Q2 What Are The Key Features Of Python
- Python is an interpreted language. That means that, unlike languages like C and its variants, Python does not need to be compiled before it is run. Other interpreted languages include PHP and Ruby.
- Python is dynamically typed, this means that you dont need to state the types of variables when you declare them or anything like that. You can do things like x=111 and then x=”I’m a string” without error
- Python is well suited to object orientated programming in that it allows the definition of classes along with composition and inheritance. Python does not have access specifiers .
- In Python, functions are first-class objects. This means that they can be assigned to variables, returned from other functions and passed into functions. Classes are also first class objects
- Writing Python code is quick but running it is often slower than compiled languages. FortunatelyPython allows the inclusion of C-based extensions so bottlenecks can be optimized away and often are. The numpy package is a good example of this, its really quite quick because a lot of the number-crunching it does isnt actually done by Python
- Python finds use in many spheres web applications, automation, scientific modeling, big data applications and many more. Its also often used as glue code to get other languages and components to play nice. Learn more about Big Data and its applications from the Data Engineering Training.