How To Use Innerhtml In React
The dangerouslySetInnerHTML attribute is React’s replacement for using innerHTML in the browser DOM. Just like innerHTML, it is risky to use this attribute considering cross-site scripting attacks. You just need to pass a __html object as key and HTML text as value.
In this example MyComponent uses dangerouslySetInnerHTML attribute for setting HTML markup:
functioncreateMarkup}functionMyComponent/> }
What Are The Advantages Of Formik Over Redux Form Library
Below are the main reasons to recommend formik over redux form library,
What Is The Benefit Of Styles Modules
It is recommended to avoid hard coding style values in components. Any values that are likely to be used across different UI components should be extracted into their own modules.
For example, these styles could be extracted into a separate component:
exportconstcolors=exportconstspace=
And then imported individually in other components:
importfrom'./styles'
Recommended Reading: When To Send Thank You Email After Interview
What Is The Use Of Useeffect React Hooks
The useEffect React Hook is used for performing the side effects in functional components. With the help of useEffect, you will inform React that your component requires something to be done after rendering the component or after a state change. The function you have passed will be remembered by React and call afterwards the performance of DOM updates is over. Using this, we can perform various calculations such as data fetching, setting up document title, manipulating DOM directly, etc, that dont target the output value. The useEffect hook will run by default after the first render and also after each update of the component. React will guarantee that the DOM will be updated by the time when the effect has run by it.
The useEffect React Hook will accept 2 arguments: useEffect
Where the first argument callback represents the function having the logic of side-effect and it will be immediately executed after changes were being pushed to DOM. The second argument dependencies represent an optional array of dependencies. The useEffect will execute the callback only if there is a change in dependencies in between renderings.
Example:
import from 'react' function WelcomeGreetings !` // Calculates output useEffect => ` // Side-effect! }, ) return < div> < /div> // Calculates output}
Can I Use Web Components In React Application
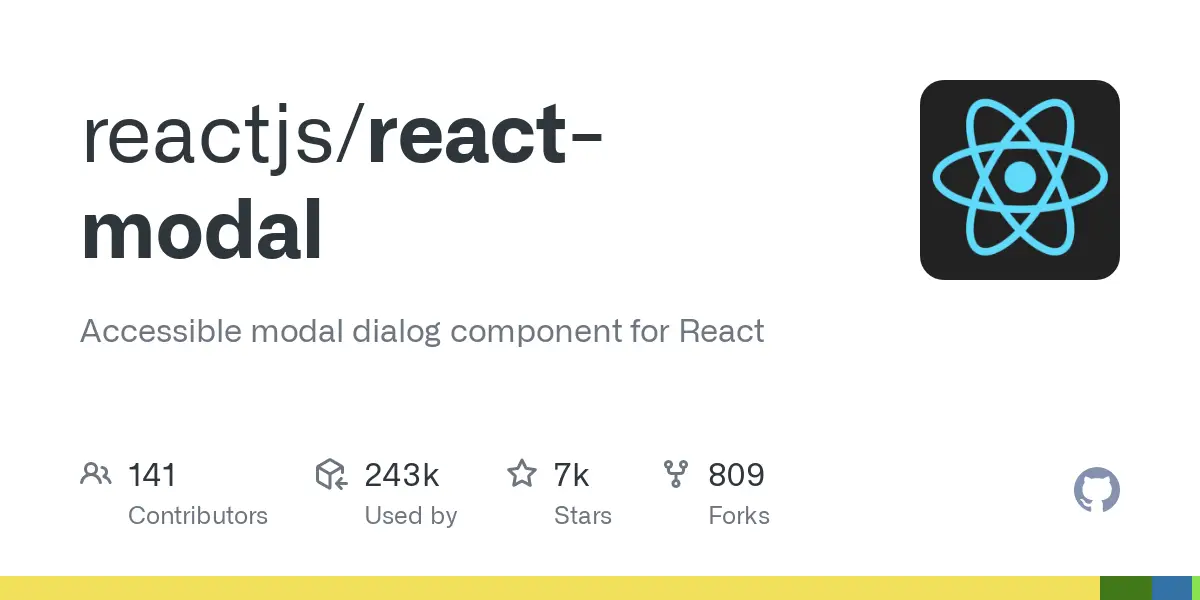
Yes, you can use web components in a react application. Even though many developers won’t use this combination, it may require especially if you are using third-party UI components that are written using Web Components.
For example, let us use Vaadin date picker web component as below,
Also Check: Candidate Did Not Show Up For Interview
What Is Shallow Renderer In React Testing
Shallow rendering is useful for writing unit test cases in React. It lets you render a component one level deep and assert facts about what its render method returns, without worrying about the behavior of child components, which are not instantiated or rendered.
For example, if you have the following component:
functionMyComponent> < /span> < spanclassName=> < /span> < /div> )}
Then you can assert as follows:
importShallowRendererfrom'react-test-renderer/shallow'// in your testconstrenderer=newShallowRendererrenderer.renderconstresult=renderer.getRenderOutputexpect.toBeexpect.toEqual
Can I Use Javascript Urls In React169
Yes, you can use javascript: URLs but it will log a warning in the console. Because URLs starting with javascript: are dangerous by including unsanitized output in a tag like < a href> and create a security hole.
constcompanyProfile= // It will log a warning< ahref=> More details< /a>
Remember that the future versions will throw an error for javascript URLs.
Recommended Reading: How To Reject Candidate After Interview
Why Should Component Names Start With Capital Letter
If you are rendering your component using JSX, the name of that component has to begin with a capital letter otherwise React will throw an error as an unrecognized tag. This convention is because only HTML elements and SVG tags can begin with a lowercase letter.
classSomeComponentextendsComponent
You can define component class which name starts with lowercase letter, but when it’s imported it should have capital letter. Here lowercase is fine:
classmyComponentextendsComponent}exportdefaultmyComponent
While when imported in another file it should start with capital letter:
importMyComponentfrom'./MyComponent'
What are the exceptions on React component naming?
The component names should start with an uppercase letter but there are few exceptions to this convention. The lowercase tag names with a dot are still considered as valid component names.For example, the below tag can be compiled to a valid component,
render
What Are The Lifecycle Methods Of React
Before React 16.3
React 16.3+
Read Also: How To Get Ready For A Phone Interview
Can You Dispatch A Redux With A Timeout If Yes Explain
The answer is Yes, If you want to play with timeout you need a JS function that is setTimeout There is no specific method that differs from JS to redux.
Redux offers other ways to deal with complex things, but you should only use those when you see that you are repeating too much code. Unless you have this problem, use the language provider and go for a simple solution.
store.dispatchsetTimeout => {
What Are The Limitations With Hocs
Higher-order components come with a few caveats apart from its benefits. Below are the few listed in an order,
Dont use HOCs inside the render method:It is not recommended to apply a HOC to a component within the render method of a component.
render
The above code impacts on performance by remounting a component that causes the state of that component and all of its children to be lost. Instead, apply HOCs outside the component definition so that the resulting component is created only once.
Static methods must be copied over:When you apply a HOC to a component the new component does not have any of the static methods of the original component
// Define a static methodWrappedComponent.staticMethod=function// Now apply a HOCconstEnhancedComponent=enhance // The enhanced component has no static methodtypeofEnhancedComponent.staticMethod==='undefined'// true
You can overcome this by copying the methods onto the container before returning it,
functionenhance// Must know exactly which method to copy :(Enhance.staticMethod=WrappedComponent.staticMethod returnEnhance }
Refs arent passed through:For HOCs you need to pass through all props to the wrapped component but this does not work for refs. This is because ref is not really a prop similar to key. In this case you need to use the React.forwardRef API
Also Check: Email To Schedule An Interview
What Are The Popular React
ESLint is a popular JavaScript linter. There are plugins available that analyse specific code styles. One of the most common for React is an npm package called eslint-plugin-react. By default, it will check a number of best practices, with rules checking things from keys in iterators to a complete set of prop types.
Another popular plugin is eslint-plugin-jsx-a11y, which will help fix common issues with accessibility. As JSX offers slightly different syntax to regular HTML, issues with alt text and tabindex, for example, will not be picked up by regular plugins.
Customize Your Own React Coding Tests
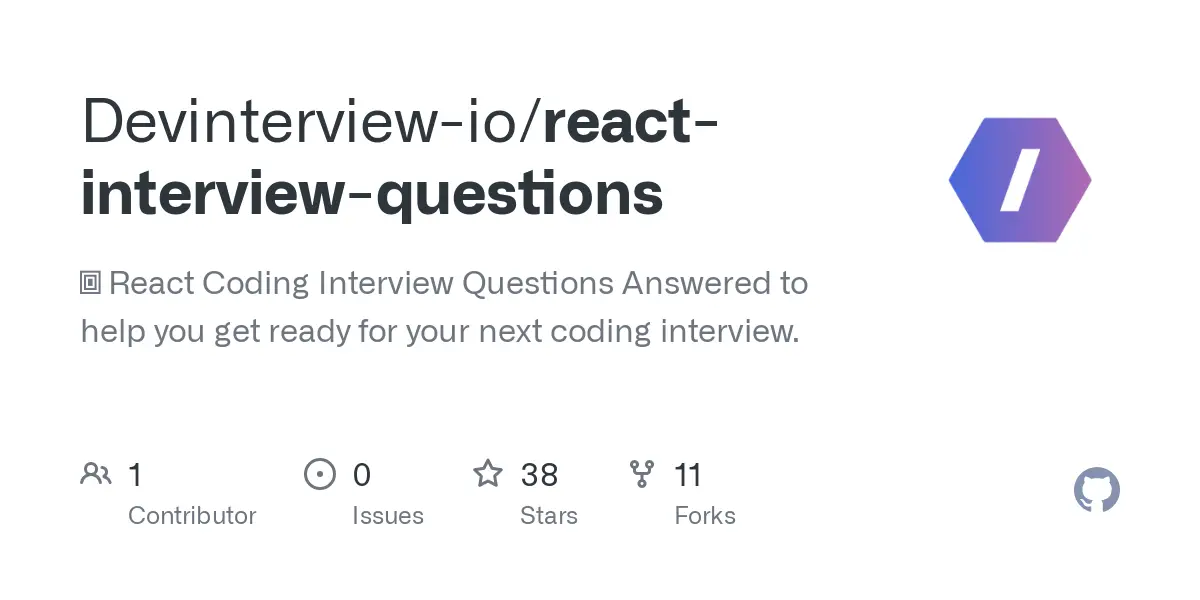
Perhaps you would like to get more out of our React coding tests? No problem!
With the DevSkiller online task wizard, you can build your very own custom React codings tests. This great feature allows you to set the technology you would like to assess, the difficulty level, and the duration of each test. You can even control the type of React interview questions you ask. Best of all, testing is carried out remotely so you can send test invitations to candidates all over the world. Our range of React coding tests are a proficient solution to finding your next developer.
Read Also: Interview Questions For Accounting Assistant
Name A Few Techniques To Optimize React App Performance
First, Use React.Suspense and React.Lazy for Lazy Loading Components. This will only load component when it is needed.
importLazyComponentfrom'./LazyComponent' constLazyComponent=React.lazy=> import)
Second, Use React.memo for Component MemoizationReact.memo is a higher order component that will render the component and memoizes the result. Before the next render, if the new props are the same, React reuses the memoized result skipping the next rendering
importReactfrom'react' constMyComponent=React.memo
Note: If React.memo has a useState, useReducer or useContext Hook in its implementation, it will still re-render when state or context change.
The more often the component renders with the same props, the heavier and the more computationally expensive the output is, the more chances are that component needs to be wrapped in React.memo.
Third, Use React.Fragment to Avoid Adding Extra Nodes to the DOM React Fragments do not produce any extra elements in the DOM Fragments child components will be rendered without any wrapping DOM node.
This is a cleaner alternative rather than adding divs in the code.
functionApp
You can also use the short syntax < > < /> for declaring a Fragment.
functionApp
Fourth, Use Reselect / Re-reselect in Redux to Avoid Frequent Re-render.Reselect is a library for building memoized selectors that is commonly used for redux.
Advantages
Re-reselect is a lightweight wrapper around Reselect to enhance selectors with deeper memoization and cache management.
What Is Key Prop And What Is The Benefit Of Using It In Arrays Of Elements
A key is a special string attribute you should include when creating arrays of elements. Key prop helps React identify which items have changed, are added, or are removed.
Most often we use ID from our data as key:
consttodoItems=todos.map=> < likey=> < /li> )
When you don’t have stable IDs for rendered items, you may use the item index as a key as a last resort:
consttodoItems=todos.map=> < likey=> < /li> )
Note:
Also Check: Need Help With Interview Skills
What Is A Switching Component
A switching component is a component that renders one of many components. We need to use object to map prop values to components.
For example, a switching component to display different pages based on page prop:
importHomePagefrom'./HomePage'importAboutPagefrom'./AboutPage'importServicesPagefrom'./ServicesPage'importContactPagefrom'./ContactPage'constPAGES=constPage==> /> }// The keys of the PAGES object can be used in the prop types to catch dev-time errors.Page.propTypes=
How To Prevent Re
- Reason for re-renders in React:
- Re-rendering of a component and its child components occur when props or the state of the component has been changed.
- Re-rendering components that are not updated, affects the performance of an application.
Consider the following components:
class Parent extends React.Component componentDidMount ) }render }class Message extends React.Component } render < /p> < /div> ) }}
- The Parent component is the parent component and the Message is the child component. Any change in the parent component will lead to re-rendering of the child component as well. To prevent the re-rendering of child components, we use the shouldComponentUpdate method:
**Note- Use shouldComponentUpdate method only when you are sure that its a static component.
class Message extends React.Component }shouldComponentUpdate render < /p> < /div> ) }}
As one can see in the code above, we have returned false from the shouldComponentUpdate method, which prevents the child component from re-rendering.
Also Check: Interview Question What Do You Know About Our Company
How To Create React Class Components Without Es6
If you dont use ES6 then you may need to use the create-react-class module instead. For default props, you need to define getDefaultProps as a function on the passed object. Whereas for initial state, you have to provide a separate getInitialState method that returns the initial state.
varGreeting=createReactClass },handleClick: function,render: function< /h1> }})
Note: If you use createReactClass then auto binding is available for all methods. i.e, You don’t need to use .bind with in constructor for event handlers.
Why Are Inline Ref Callbacks Or Functions Not Recommended
If the ref callback is defined as an inline function, it will get called twice during updates, first with null and then again with the DOM element. This is because a new instance of the function is created with each render, so React needs to clear the old ref and set up the new one.
classUserFormextendsComponentrender> < inputtype='text'ref=/> // Access DOM input in handle submit< buttontype='submit'> Submit< /button> < /form> )}}
But our expectation is for the ref callback to get called once, when the component mounts. One quick fix is to use the ES7 class property syntax to define the function
classUserFormextendsComponentsetSearchInput==> render> < inputtype='text'ref=/> // Access DOM input in handle submit< buttontype='submit'> Submit< /button> < /form> )}}
**Note:** In React v16.3,
You May Like: What Are The Interview Questions For Excel
What Is The Purpose Of Displayname Class Property
The displayName string is used in debugging messages. Usually, you dont need to set it explicitly because its inferred from the name of the function or class that defines the component. You might want to set it explicitly if you want to display a different name for debugging purposes or when you create a higher-order component.
For example, To ease debugging, choose a display name that communicates that its the result of a withSubscription HOC.
functionwithSubscriptionWithSubscription.displayName=`WithSubscription` returnWithSubscription }functiongetDisplayName
What Is React Hooks
![[Question] How to get typesafe injection · Issue #256 · mobxjs/mobx ... [Question] How to get typesafe injection · Issue #256 · mobxjs/mobx ...](https://www.interviewprotips.com/wp-content/uploads/question-how-to-get-typesafe-injection-issue-256-mobxjsmobx.png)
React Hooks are the built-in functions that permit developers for using the state and lifecycle methods within React components. These are newly added features made available in React 16.8 version. Each lifecycle of a component is having 3 phases which include mount, unmount, and update. Along with that, components have properties and states. Hooks will allow using these methods by developers for improving the reuse of code with higher flexibility navigating the component tree.
Using Hook, all features of React can be used without writing class components. For example, before React version 16.8, it required a class component for managing the state of a component. But now using the useState hook, we can keep the state in a functional component.
Recommended Reading: How To Answer Typical Interview Questions
What Are The Core Principles Of Redux
Redux follows three fundamental principles:
What Are Error Boundaries In React V16
Error boundaries are components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the component tree that crashed.
A class component becomes an error boundary if it defines a new lifecycle method called componentDidCatch or static getDerivedStateFromError :
classErrorBoundaryextendsReact.Component}componentDidCatchstaticgetDerivedStateFromError }render< /h1> }returnthis.props.children}}
After that use it as a regular component:
< ErrorBoundary> < MyWidget/> < /ErrorBoundary>
Don’t Miss: How To Prepare For A Phone Interview With A Recruiter
What Is Redux Thunk
Redux Thunk middleware allows you to write action creators that return a function instead of an action. The thunk can be used to delay the dispatch of an action, or to dispatch only if a certain condition is met. The inner function receives the store methods dispatch and getState as parameters.
Displaying Data Coming From An Api
If you are a mid-level experienced React developer, you must have enough working experience for handling APIs. In this coding challenge, you will be provided with an API that will return some data, maybe, an array of objects. You have to display the data in the UI.
The main motive here is to check how and where the API is called by the developer. In React, there are two ways to call APIs.
- Axios
- fetch API
While fetch API is inbuilt, Axios is installed through NPM and is considered a better option. So first, install Axios using npm and then use the following API to fetch the data.
https://jsonplaceholder.typicode.com/posts/1/commentsimport React, from"react" import axios from"axios" const App = => = await axios.get setData } useEffect => , ) return })}< /ui> < /div> ) } exportdefault App
As mentioned earlier, the interviewers want to see how you call the API.
Here, Axios is used. Also, to handle the asynchronous request, ES6 async/await syntax is used. You can also use promises.
Another important part is where you call the API. Here, the API call is made in the useEffect hook with an empty dependency array, meaning, the data will be available when the component is mounted.
Don’t Miss: How To Write A Follow Up Interview Thank You Email
What Are Stateless Components
If the behaviour of a component is independent of its state then it can be a stateless component. You can use either a function or a class for creating stateless components. But unless you need to use a lifecycle hook in your components, you should go for function components. There are a lot of benefits if you decide to use function components here they are easy to write, understand, and test, a little faster, and you can avoid the this keyword altogether.